- 代码
class Node{
int val;
Node next;
Node (int val, Node next) {
this.val=val;
this.next=next;
}
Node (int val){
this (val,null);
}
}
public class Queue {
private Node head=null;
private Node tail=null;
private int size=0;
public void offer(int i){
Node node=new Node(i);
if (tail==null){
head=node;
}else{
tail.next=node;
}
tail=node;
size++;
}
public int poll(){
if(head==tail){
return Integer.parseInt( null );
}
Node oldhead=head;
head=head.next;
if(head==null){
tail=null;
}
size--;
return oldhead.val;
}
public int peek(){
if(head==tail){
return Integer.parseInt( null );
}
Node oldhead=head;
return oldhead.val;
}
public int size(){
return size;
}
public static void main(String[] args) {
Queue Offer=new Queue();
Offer.offer(1);
Offer.offer(2);
Offer.offer(3);
System.out.println(Offer.size());
System.out.println(Offer.poll());
}
}
- 代码测试用例:
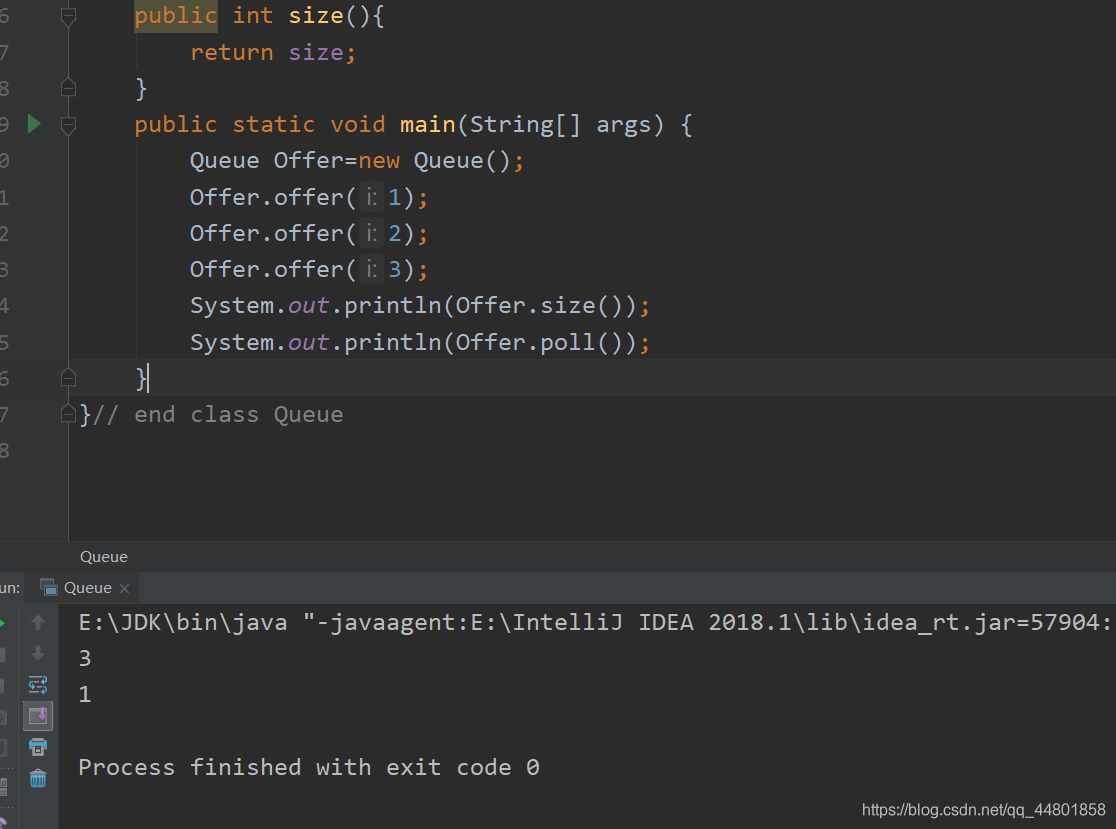