第3章 栈和队列
3.3 栈和队列的应用
综合应用题 第1题

#include <stdio.h>
#include <stdlib.h>
#include <iostream>
#define MaxSize 10
using namespace std;
typedef char ElemType;
typedef struct{
ElemType stack[MaxSize];
int top;
}SqStack;
void InitStack(SqStack &S)
{
S.top=-1;
}
int Push(SqStack &S,ElemType x)
{
if(S.top==MaxSize-1)
{
cout<<"栈满"<<endl;
exit(0);
}
S.stack[++S.top]=x;
return 1;
}
int Pop(SqStack &S,ElemType &x)
{
if(S.top==-1)
{
cout<<"栈空"<<endl;
return 0;
}
x=S.stack[S.top--];
return 1;
}
void PrintStack(SqStack S)
{
while(S.top!=-1)
{
cout<<S.stack[S.top]<<" ";
S.top--;
}
cout<<endl;
}
bool StatckEmpty(SqStack &S)
{
if(S.top==-1)
return true;
else
return false;
}
int main() {
SqStack S;
InitStack(S);
string s;
bool flag=true;
char c='0';
cout<<"请输入算术表达式:" <<endl;
cin>>s;
for(int i=0;i<s.length();i++)
{
switch(s[i])
{
case '(': Push(S,s[i]); break;
case '[': Push(S,s[i]); break;
case '{': Push(S,s[i]); break;
case ')':Pop(S,c);
if(c!='(')
return 0;
break;
case ']':Pop(S,c);
if(c!='[')
return 0;
break;
case '}':Pop(S,c);
if(c!='{')
return 0;
break;
default:break;
}
}
if(!StatckEmpty(S))
cout<<"/匹配失败!"<<endl;
else
cout<<"匹配成功!"<<endl;
return 0;
}
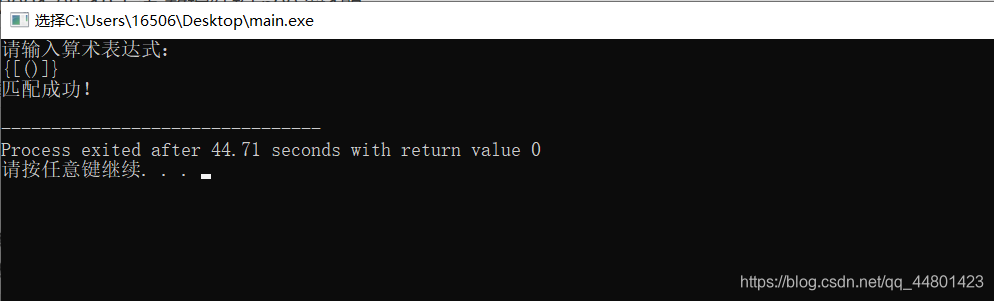