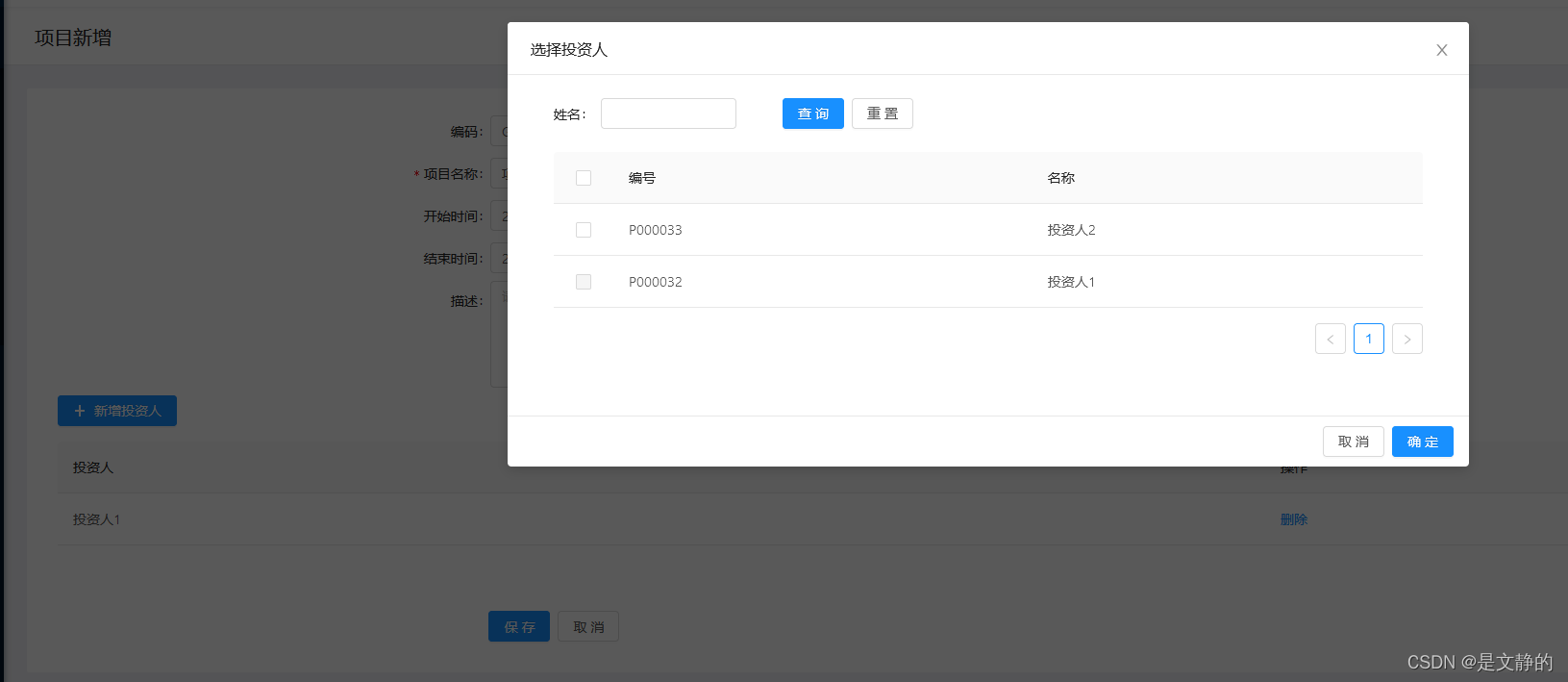
<template>
<a-modal
title="选择投资人"
:visible="visible"
:confirmLoading="confirmLoading"
:width="1000"
@cancel="handleCancel"
@ok="handleSubmit"
:maskClosable="false"
>
<a-card :bordered="false">
<div class="table-page-search-wrapper">
<a-form layout="inline">
<a-row :gutter="48">
<a-col :md="6" :sm="24">
<a-form-item label="姓名">
<a-input v-model="queryParam.chn_name" placeholder=""/>
</a-form-item>
</a-col>
<a-col :md="!advanced && 6 || 24" :sm="24">
<span class="table-page-search-submitButtons" :style="advanced && { float: 'right', overflow: 'hidden' } || {} ">
<a-button type="primary" @click="$refs.table.refresh(true)">查询</a-button>
<a-button style="margin-left: 8px" @click="() => queryParam = {}">重置</a-button>
</span>
</a-col>
</a-row>
</a-form>
</div>
<a-table
ref="table"
size="default"
rowKey="record_id"
:columns="columns"
:data-source="projectData"
:alert="options.alert"
:rowSelection="{selectedRowKeys: selectedRowKeys, onChange: onSelectChange, getCheckboxProps: getCheckboxProps}"
>
<span slot="investor" slot-scope="text, record">
{{ record.chn_name }}
</span>
</a-table>
</a-card>
</a-modal>
</template>
<script>
import moment from 'moment/moment'
import { STable } from '@/components'
import { getInvestorPageList } from '@/api/project/business/investor'
const statusMap = {
1: {
status: 'default',
text: '无效'
},
2: {
status: 'processing',
text: '发布'
}
}
export default {
components: {
STable
},
data () {
return {
visible: false,
confirmLoading: false,
mdl: {},
projectData: [],
investorData: [],
advanced: false,
queryParam: {},
columns: [
{
title: '编号',
dataIndex: 'index_code'
},
{
title: '名称',
dataIndex: 'investor',
scopedSlots: { customRender: 'investor' }
}
],
selectedRowKeys: [],
selectedRows: [],
optionAlertShow: false
}
},
filters: {
statusTextFilter (type) {
return statusMap[type].text
},
statusShowFilter (type) {
return statusMap[type].status
}
},
created () {
this.tableOption()
},
methods: {
add (investorData) {
this.investorData = investorData
console.log(this.investorData)
this.loadprojectList()
this.visible = true
},
loadprojectList () {
getInvestorPageList()
.then(res => {
this.projectData = res.result.data
console.log(this.projectData)
})
},
getCheckboxProps (record) {
return ({
props: {
disabled: this.investorData.some(item => item.investor.record_id === record.record_id),
chn_name: record.chn_name
}
})
},
clear () {
this.selectedRowKeys = []
},
tableOption () {
if (!this.optionAlertShow) {
this.options = {
alert: { show: true, clear: () => { this.selectedRowKeys = [] } },
rowSelection: {
selectedRowKeys: this.selectedRowKeys,
onChange: this.onSelectChange
}
}
this.optionAlertShow = true
} else {
this.options = {
alert: false,
rowSelection: null
}
this.optionAlertShow = false
}
},
handleSubmit () {
this.visible = false
this.$emit('select', this.selectedRowKeys)
this.clear()
},
onSelectChange (selectedRowKeys, selectedRows) {
this.selectedRowKeys = selectedRowKeys
this.selectedRows = selectedRows
},
toggleAdvanced () {
this.advanced = !this.advanced
},
resetSearchForm () {
this.queryParam = {
date: moment(new Date())
}
},
handleBatchDelete () {
this.$message.error(`${this.selectedRowKeys.length} 条数据待删除`)
},
handleCancel () {
this.visible = false
},
handleOk () {
this.$refs.table.refresh()
}
}
}
</script>