一、整体逻辑
- 两个无关的进程A、B;
- 创建两个有名管道1和2;
- 进程A中以只写的方式打开管道1,以只读的方式打开管道2;
- 进程B中以只读的方式打开管道1,以只写的方式打开管道2;
- 在进程A中fork()一个子进程,父进程负责写管道1,fgets()从键盘录入,子进程负责读管道2,分别用两个while循环不断读写;
- 在进程B中fork()一个子进程,父进程负责读管道1,子进程负责写管道2,fgets()从键盘录入,分别用两个while循环不断读写;
- 完成通信
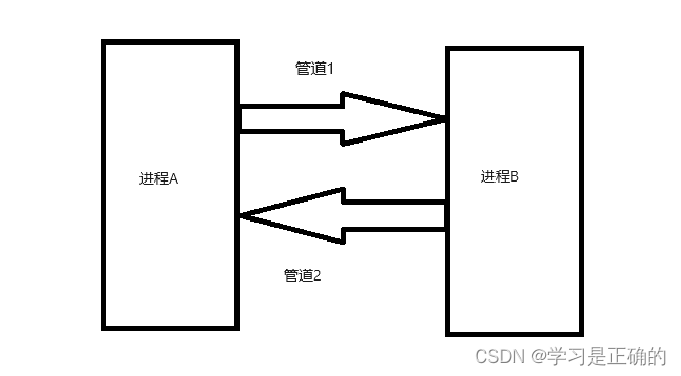
二、代码实现
#include <sys/types.h>
#include <sys/stat.h>
#include <stdlib.h>
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <string.h>
#include <time.h>
int main(int argc, char const *argv[])
{
time_t timer;
struct tm *tblock;
timer = time(NULL);
int ret = access("fifo1",F_OK);
if(ret==-1)
{
printf("fifo1管道不存,创建管道!\n");
ret = mkfifo("fifo1",0664);
if(ret==-1)
{
perror("mkfifo");
exit(0);
}
}
ret = access("fifo2",F_OK);
if(ret==-1)
{
printf("fifo2管道不存,创建管道!\n");
ret = mkfifo("fifo2",0664);
if(ret==-1)
{
perror("mkfifo");
exit(0);
}
}
int fd1 = open("fifo1",O_WRONLY);
if(fd1==-1)
{
perror("open");
exit(0);
}
int fd2 = open("fifo2",O_RDONLY);
if(fd2==-1)
{
perror("open");
exit(0);
}
pid_t pid = fork();
char buf[1024]={0};
if(pid==0)
{
while(1)
{
int len = read(fd2,buf,sizeof(buf));
if(len==0)
{
printf("B断开连接!\n");
exit(0);
}
else if(len>0)
{
timer = time(NULL);
tblock = localtime(&timer);
printf("%sB say:%s\n",asctime(tblock),buf);
memset(buf,0,sizeof(buf));
}
else if(len==-1)
{
perror("read");
}
}
}
else if(pid>0)
{
while(1)
{
fgets(buf,sizeof(buf),stdin);
timer = time(NULL);
tblock = localtime(&timer);
write(fd1,buf,sizeof(buf));
printf("%sA say:%s\n",asctime(tblock),buf);
memset(buf,0,sizeof(buf));
}
}
else if(pid==-1)
{
perror("fork");
exit(0);
}
return 0;
}
#include <sys/types.h>
#include <sys/stat.h>
#include <stdlib.h>
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <string.h>
#include <time.h>
int main(int argc, char const *argv[])
{
time_t timer;
struct tm *tblock;
int ret = access("fifo1",F_OK);
if(ret==-1)
{
printf("fifo1管道不存,创建管道!\n");
ret = mkfifo("fifo1",0664);
if(ret==-1)
{
perror("mkfifo");
exit(0);
}
}
ret = access("fifo2",F_OK);
if(ret==-1)
{
printf("fifo2管道不存,创建管道!\n");
ret = mkfifo("fifo2",0664);
if(ret==-1)
{
perror("mkfifo");
exit(0);
}
}
int fd1 = open("fifo1",O_RDONLY);
if(fd1==-1)
{
perror("open");
exit(0);
}
int fd2 = open("fifo2",O_WRONLY);
if(fd2==-1)
{
perror("open");
exit(0);
}
pid_t pid = fork();
char buf[1024] = {0};
if(pid==0)
{
while(1)
{
fgets(buf,sizeof(buf),stdin);
timer = time(NULL);
tblock = localtime(&timer);
write(fd2,buf,sizeof(buf));
printf("%sB say:%s\n",asctime(tblock),buf);
memset(buf,0,sizeof(buf));
}
}
else if(pid>0)
{
while(1)
{
int len = read(fd1,buf,sizeof(buf));
if(len==0)
{
printf("A断开连接\n");
exit(0);
}
else if(len>0)
{
timer = time(NULL);
tblock = localtime(&timer);
printf("%sA say:%s\n",asctime(tblock),buf);
memset(buf,0,sizeof(buf));
}
else if(len==-1)
{
perror("read");
}
}
}
else if(pid==-1)
{
perror("fork");
exit(0);
}
return 0;
}
三、效果展示
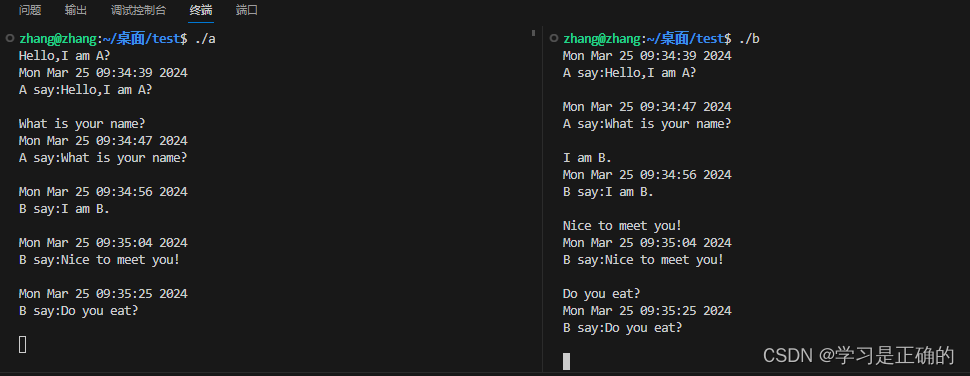