GUI编程
告诉大家怎么学
- 这是什么?
- 它怎么玩?
- 该如何去我们平时运用?
组件
- 窗口
- 弹窗
- 面板
- 文本框
- 列表框
- 按钮
- 图片
- 监听事件
- 鼠标事件
- 键盘事件
- 破解工具
1、简介
GUI的核心技术:Swing ,AWT
- 界面不美观
- 需要jre环境
为什么我们要学习?
- 可以写出自己心中想要的小工具
- 工作时候,也可能需要维护Swing界面,概率极小
- 了解MVC架构,了解监听
2、AWT
2.1、Awt介绍
- 包含了很多类和接口,GUI
- 元素: 窗口、按钮、文本框
- java.awt
2.2 、组件和容器
1. Frame
- 弹出单个窗口
import java.awt.*;
//GUI的第一个程序
public class GuiProject {
public static void main(String[] args) {
Frame frame = new Frame("第一个GUI窗口程序");
frame.setVisible(true);//设置窗口可见性
frame.setSize(400,200);//设置窗口大小
frame.setBackground(new Color(0x0F0FC0));//升值窗口颜色
frame.setLocation(200,200);//设置窗口起始位置
frame.setResizable(false);//设置窗口大小是否可调,默认为true
}
}
现在的问题:无法关闭窗口,需要停止java程序
- 弹出多个窗口
import java.awt.*;
public class MultipleWindows {
public static void main(String[] args) {
for (int i = 0; i < 100; i++) {
new MyFrame(i%20*80,i/20*200,400,400,new Color(i*100));
}
}
}
class MyFrame extends Frame{
static int id=0;
MyFrame(int x,int y,int w,int h,Color color){
super("第"+(++id)+"个窗口");
setBounds(x,y,w,h);//设置窗口大小和位置
setVisible(true);//设置窗口可见性
setBackground(color);//设置窗口颜色
}
}
2.面板Panel
解决了窗口关闭问题
public class TestPanel {
public static void main(String[] args) {
Frame frame = new Frame();//创建窗口
Panel panel = new Panel();//创建面板
frame.setVisible(true);//设置可见性
frame.setBackground(Color.blue);//设置颜色
frame.setBounds(200,200,500,500);//设置窗口位置大小
panel.setBackground(Color.cyan);//设置面板颜色
panel.setBounds(50,50,400,400);//设置面板位置大小
frame.setLayout(null);//设置布局
frame.add(panel);//添加面板到窗口
//监听窗口事件
frame.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {//监听关闭按钮
System.exit(0);//退出进程
}
});
}
}
2.3、布局管理器
- 流式布局
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class TasteFlowLayout {
public static void main(String[] args) {
Frame frame = new Frame();
Button button1 = new Button("button1");
Button button2 = new Button("button2");
Button button3 = new Button("button3");
//frame.setLayout(new FlowLayout(FlowLayout.CENTER));//中心
frame.setLayout(new FlowLayout(FlowLayout.RIGHT));//右
//frame.setLayout(new FlowLayout(FlowLayout.LEADING));//左
frame.setVisible(true);
frame.setBounds(100,100,500,500);
frame.setBackground(new Color(0x8C2424));
frame.add(button1);//添加按钮
frame.add(button2);
frame.add(button3);
//监听窗口事件
frame.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {//监听关闭按钮
System.exit(0);//退出进程
}
});
}
}
- 东西南北中
import java.awt.*;
public class TasteBorderLayout {
public static void main(String[] args) {
Frame frame = new Frame("TasteBorderLayout");
Button east = new Button("East");
Button west = new Button("West");
Button south = new Button("South");
Button north = new Button("North");
Button center = new Button("Center");
frame.setVisible(true);
frame.setSize(300,300);
frame.add(east,BorderLayout.EAST);
frame.add(west,BorderLayout.WEST);
frame.add(south,BorderLayout.SOUTH);
frame.add(north,BorderLayout.NORTH);
frame.add(center,BorderLayout.CENTER);
}
}
``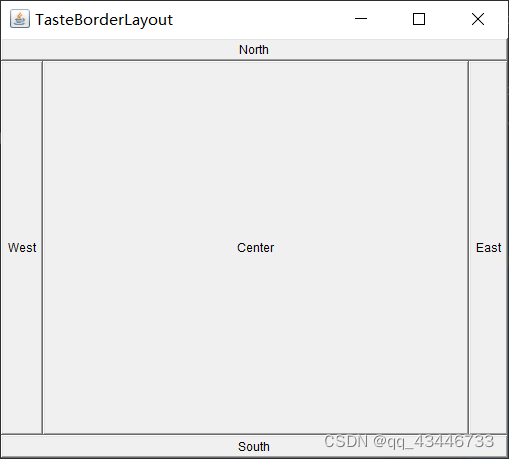
`
- 表格布局
```java
import java.awt.*;
public class TestGritLayout {
public static void main(String[] args) {
Frame frame = new Frame("TestGritLayout");
Button but1 = new Button("but1");
Button but2 = new Button("but2");
Button but3 = new Button("but3");
Button but4 = new Button("but4");
Button but5 = new Button("but5");
Button but6 = new Button("but6");
frame.setLayout(new GridLayout(3,2));//表格布局3行2列
frame.add(but1);
frame.add(but2);
frame.add(but3);
frame.add(but4);
frame.add(but5);
frame.add(but6);
frame.pack();//自动布局
frame.setVisible(true);
}
}
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class TestLayout {
public static void main(String[] args) {
Frame frame = new Frame("测试Layout");
Panel panel1 = new Panel(new BorderLayout());//创建面板,面板传入布局方式
Panel panel2 = new Panel(new GridLayout(2,1));//创建面板
Panel panel3 = new Panel(new BorderLayout());//创建面板
Panel panel4 = new Panel(new GridLayout(2,2));//创建面板
frame.setLayout(new GridLayout(2,1));//表格布局2行1列
frame.setVisible(true);
frame.setSize(500,500);;
frame.setLocation(500,500);;
panel1.add(new Button("panel1-1"),BorderLayout.EAST);
panel1.add(new Button("panel1_2"),BorderLayout.WEST);
panel1.add(panel2,BorderLayout.CENTER);
panel2.add(new Button("panel2-1"));
panel2.add(new Button("panel2-2"));
panel3.add(new Button("panel3-1"),BorderLayout.EAST);
panel3.add(new Button("panel3_2"),BorderLayout.WEST);
panel3.add(panel4,BorderLayout.CENTER);
for (int i = 0; i < 4; i++) {
panel4.add(new Button("panel4-"+i));
}
frame.add(panel1);
frame.add(panel3);
//监听窗口事件
frame.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {//监听关闭按钮
System.exit(0);//退出进程
}
});
}
}
总结
- Frame是一个顶级窗口
- Panel无法单独显示;必须添加到某个容器里
- 布局管理器
- 流式
- 东西南北中
- 表格
- 大小,定位,背景颜色,可见性,监听
2.4、事件监听
当某一个事情发生的时候干什么
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class ButtonAction {
public static void main(String[] args) {
Frame frame = new Frame("按钮事件监听");
Button button = new Button("button");
button.addActionListener(new MyAction());//添加事件监听
frame.setSize(500,500);
frame.setVisible(true);
frame.add(button,BorderLayout.CENTER);//把按钮放在窗口中心
windowClose(frame);//关闭窗口事件
}
private static void windowClose(Frame frame){//提出窗口关闭事件,做成方法方便调用
frame.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
super.windowClosed(e);
System.exit(0);
}
});
}
}
class MyAction implements ActionListener{//继承按钮监听接口
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("按钮按下");
}
}
多个按钮共享一个事件
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class ButtonsAction {
public static void main(String[] args) {
Frame frame = new Frame("多个按钮共用一个按钮事件");
Button button1 = new Button("open");
Button button2 = new Button("close");
//设置触发事件时e.getActionCommand()获取到的值
//可以显示的定义命令触发会显示的值,如果不定义则显示默认值
//button1.setActionCommand("按钮1");
//多个按钮只写一个监听类
MyActions myAction = new MyActions();
button1.addActionListener(myAction);//设置按钮监听
button2.addActionListener(myAction);
frame.setVisible(true);
frame.setSize(500,500);
frame.add(button1,BorderLayout.EAST);
frame.add(button2,BorderLayout.WEST);
windowClose(frame);
}
public static void windowClose(Frame frame){//提出关闭窗口事件
frame.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
}
class MyActions implements ActionListener{
private Frame frame;
@Override
public void actionPerformed(ActionEvent e) {
System.out.println(e.getActionCommand());
if(e.getActionCommand().equals("open")){//当按下open按键时打开新窗口
if(frame==null){
System.out.println("打开新窗口");
frame=new Frame("新打开的窗口");
frame.setBackground(Color.blue);
frame.setSize(300,300);
frame.setVisible(true);
}
} else if (e.getActionCommand().equals("close")) {//当按下close按键时关闭新窗口
if (frame!=null){
System.out.println("关闭新窗口");
ButtonsAction.windowClose(frame);
frame=null;
}
}
}
}
2.5、输入框 TextField 监听
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class TextFieldAction {
public static void main(String[] args) {
new MyTextField();
}
}
class MyTextField extends Frame{
public MyTextField(){
TextField textField = new TextField();
setSize(500,500);
setVisible(true);
add(textField);
MyActionListening myActionListening = new MyActionListening();
textField.addActionListener(myActionListening);
textField.setEchoChar('+');//设置替换字符,比如输入密码时的'*'
//pack();
addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
}
class MyActionListening implements ActionListener{
@Override
public void actionPerformed(ActionEvent e) {
TextField text = (TextField) e.getSource();
System.out.println(text.getText());
// text.setText("");
}
}
2.6、简易计算器,组合+内部类回顾复习
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class TestCalculator {
public static void main(String[] args) {
new Calculator();
}
}
class Calculator extends Frame{
public Calculator(){
//创建3个文本框
TextField num1 = new TextField(10);//字符数
TextField num2 = new TextField(10);
TextField num3 = new TextField(20);
//创建1 个按钮
Button button = new Button("=");
button.addActionListener(new CalculatorListener(num1,num2,num3));
//创建1和标签
Label label = new Label("+");
setLayout(new FlowLayout());//流式布局
add(num1);
add(label);
add(num2);
add(button);
add(num3);
pack();
setVisible(true);
}
}
class CalculatorListener implements ActionListener{
TextField num1,num2,num3;
//获取3个属性
public CalculatorListener(TextField num1, TextField num2, TextField num3) {
this.num1 = num1;
this.num2 = num2;
this.num3 = num3;
}
@Override
public void actionPerformed(ActionEvent e) {
int n1 = Integer.parseInt(num1.getText());
int n2 = Integer.parseInt(num2.getText());
num3.setText(""+(n1+n2));
num1.setText("");
num2.setText("");
}
}
完全改造为面向对象
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class TestCalculator {
public static void main(String[] args) {
new Calculator();
}
}
class Calculator extends Frame{
TextField num1 = new TextField(10);
TextField num2 = new TextField(10);
TextField num3 = new TextField(20);
public Calculator(){
//创建3个文本框
//创建1 个按钮
Button button = new Button("=");
button.addActionListener(new CalculatorListener(this));
//创建1和标签
Label label = new Label("+");
setLayout(new FlowLayout());//流式布局
add(num1);
add(label);
add(num2);
add(button);
add(num3);
pack();
setVisible(true);
}
}
class CalculatorListener implements ActionListener{
//组合,获取计算器对象
private Calculator calculator;
public CalculatorListener(Calculator calculator) {
this.calculator = calculator;
}
@Override
public void actionPerformed(ActionEvent e) {
int n1 = Integer.parseInt(calculator.num1.getText());
int n2 = Integer.parseInt(calculator.num2.getText());
calculator.num3.setText(""+(n1+n2));
calculator.num1.setText("");
calculator.num2.setText("");
}
}
简化为内部类
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class TestCalculator {
public static void main(String[] args) {
new Calculator().calculate();
}
}
class Calculator extends Frame{
//创建3个文本框
TextField num1 = new TextField(10);
TextField num2 = new TextField(10);
TextField num3 = new TextField(20);
public void calculate(){
//创建1 个按钮
Button button = new Button("=");
button.addActionListener(new CalculatorListener());
//创建1和标签
Label label = new Label("+");
setLayout(new FlowLayout());//流式布局
add(num1);
add(label);
add(num2);
add(button);
add(num3);
pack();
setVisible(true);
}
class CalculatorListener implements ActionListener{
//组合,获取计算器对象
@Override
public void actionPerformed(ActionEvent e) {
int n1 = Integer.parseInt(num1.getText());
int n2 = Integer.parseInt(num2.getText());
num3.setText(""+(n1+n2));
num1.setText("");
num2.setText("");
}
}
}
2.7、画笔
import java.awt.*;
public class TestPaintbrush {
public static void main(String[] args) {
new TextDrawing().Window();
}
}
class TextDrawing extends Frame{
public void Window(){
setVisible(true);
setBounds(100,100,500,500);
}
@Override
public void paint(Graphics g) {//重写画笔方法
super.paint(g);
g.setColor(Color.blue);//设置颜色
g.draw3DRect(100,300,100,100,false);//画一个空心矩形
for (int i = 0; i < 100; i++) {
g.fill3DRect(100,100,100,100,false);//画一个实体矩形
g.setColor(new Color(i*10086));//画一个闪闪的矩形
try {
Thread.sleep(50);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
//用完画笔把他还原成原来的颜色:黑色0x0000
g.setColor(Color.black);//设置颜色
}
}
2.8、鼠标监听
目标:实现鼠标画画
import java.awt.*;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.util.ArrayList;
import java.util.Iterator;
public class MouseListening {
public static void main(String[] args) {
new MyMouseListening();
}
}
class MyMouseListening extends Frame {
private ArrayList points;
public MyMouseListening(){
points=new ArrayList<>();//创建表对象
setVisible(true);
setBounds(100,100,500,500);
addMouseListener(new Mouse());//添加鼠标监听事件
}
@Override
public void paint(Graphics g) {//画笔
g.setColor(Color.blue);//设置画笔颜色:蓝色
Iterator iterator = (Iterator) points.iterator();//创建列表迭代器
while (iterator.hasNext()){//判断是否还有下一个
Point point = (Point) iterator.next();//指针指向下一个,并返回当前数据
g.fillOval(point.x, point.y,10,10);//画个圆点
}
}
public void addPaint(Point point){//中间商
points.add(point);
}
private class Mouse extends MouseAdapter{//鼠标监听,继承鼠标适配器
@Override
public void mouseClicked(MouseEvent e) {//单击事件
super.mouseClicked(e);
System.out.println("单击");
MyMouseListening frame = (MyMouseListening)e.getSource();
frame.addPaint(new Point(e.getX(),e.getY()));//添加鼠标的坐标
frame.repaint();//重画页面
}
@Override
public void mousePressed(MouseEvent e) {//按压事件
super.mousePressed(e);
System.out.println("按压");
}
@Override
public void mouseReleased(MouseEvent e) {//鼠标释放事件
super.mouseReleased(e);
System.out.println("释放");
}
@Override
public void mouseEntered(MouseEvent e) {//鼠标进入页面事件
super.mouseEntered(e);
System.out.println("进入");
}
@Override
public void mouseExited(MouseEvent e) {//鼠标离开页面事件
super.mouseExited(e);
System.out.println("退出");
}
}
}
2.9、窗口监听
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class WindowListener {
public static void main(String[] args) {
new MyFrame();
}
}
class MyFrame extends Frame{
public MyFrame(){
setVisible(true);
setBounds(100,100,500,500);
setBackground(Color.blue);
addWindowListener(new WindowAdapter() {//添加窗口监听事件
@Override
public void windowClosing(WindowEvent e) {
super.windowClosing(e);
System.out.println("已关闭");
System.exit(0);
}
@Override
public void windowActivated(WindowEvent e) {
super.windowActivated(e);
MyFrame frame = (MyFrame) e.getSource();
frame.setTitle("我回来了");
System.out.println("已激活");
}
@Override
public void windowDeactivated(WindowEvent e) {
super.windowDeactivated(e);
MyFrame frame = (MyFrame) e.getSource();
frame.setTitle("我走了");
System.out.println("非激活");
}
});
}
}
2.10、键盘监听
import java.awt.*;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
public class KeyListener {
public static void main(String[] args) {
new KeyFrame();
}
}
class KeyFrame extends Frame {
public KeyFrame(){
setBounds(100,200,500,500);
setVisible(true);
addKeyListener(new KeyAdapter() {//添加键盘监听
@Override
public void keyPressed(KeyEvent e) {//键盘按下事件
super.keyPressed(e);
int keyCode = e.getKeyCode();
System.out.println(keyCode);
if(keyCode==KeyEvent.VK_UP){
//按下上键
System.out.println("你按下了上键");
}
}
});
}
}
所有键值都是KeyEvent 类里的属性常量
3、Swing
3.1、窗口,面板 JFrame
import javax.swing.*;
import java.awt.*;
public class TestJFrame {
public static void main(String[] args) {
new MyJFrame().init();
}
}
class MyJFrame extends JFrame{
public void init(){
Container contentPane = getContentPane();//要创建一个容器设置页面颜色
contentPane.setBackground(Color.YELLOW);
setBounds(100,100,500,500);
setVisible(true);
JLabel label = new JLabel("这是一个标签");
label.setHorizontalAlignment(SwingConstants.CENTER);//设置标签居中
add(label);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);//关闭窗口
}
}
3.2、弹窗
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class TestDialog {
public static void main(String[] args) {
new MyJFrame1().init();
}
}
class MyJFrame1 extends JFrame {
public void init(){
setBounds(100,100,500,500);
setTitle("主窗口测试弹窗");
setVisible(true);
Container container = getContentPane();
JButton jButton = new JButton("按下打开弹窗");
jButton.setSize(400,200);
jButton.addActionListener(new ActionListener() {//按钮动作监听
@Override
public void actionPerformed(ActionEvent e) {
new MyDialog();
}
});
container.add(jButton);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);//关闭窗口,如果不写点叉号默认隐藏窗口
}
}
class MyDialog extends JDialog{//弹窗
public MyDialog() {
this.add(new JLabel("这是一个弹窗标签")) ;
setBounds(100,100,400,400);
setVisible(true);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
}
}
3.3、标签
图标
import javax.swing.*;
import java.awt.*;
public class IconLabel {
public static void main(String[] args) {
new DiagramLabel(15,20);
}
}
class DiagramLabel extends JFrame implements Icon{
private int width;//图标的宽
private int height;//图标的高
public DiagramLabel(){}//无参构造
public DiagramLabel(int width,int height){//有参构造
this.width= width;
this.height=height;
JLabel jLabel = new JLabel("DiagramLabel",this,SwingConstants.CENTER);//创建一个标签,显示图标,显示在窗口中间
setBounds(100,100,500,500);
setVisible(true);
add(jLabel);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);//关闭窗口监听
}
//实现Icon接口
@Override
public void paintIcon(Component c, Graphics g, int x, int y) {
g.fillOval(x,y,width,height);
}
@Override
public int getIconWidth() {
return width;
}
@Override
public int getIconHeight() {
return height;
}
}
图片
import javax.swing.*;
import java.awt.*;
import java.net.*;
//显示图片标签
public class PictureLabel {
public static void main(String[] args) {
//PictureLabel.class.getResource("icon.png"):获取这个类目录下的文件,返回一个URL地址
new TestLabel(PictureLabel.class.getResource("icon.png")).init();
}
}
class TestLabel extends JFrame{
private URL url;
public TestLabel(){}
public TestLabel(URL url){
this.url=url;
}
public void init(){
JLabel jLabel = new JLabel("PictureLabel");//实例化一个标签
ImageIcon icon = new ImageIcon(url);//实例化图片
jLabel.setIcon(icon);//将图片设置到标签上
jLabel.setHorizontalAlignment(SwingUtilities.CENTER);//显示在窗口的中间
Container container = getContentPane();//获取容器
container.add(jLabel);//将标签添加到容器
setBounds(100,100,500,500);
setVisible(true);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
}
}
3.4、面板
面板间距
import javax.swing.*;
import java.awt.*;
//测试面板间距
public class JPanelDemo extends JFrame {
public JPanelDemo() {
Container container = getContentPane();
container.setLayout(new GridLayout(2,2,10,10));//后面的参数是面板之间的间距
JPanel jPanel1 = new JPanel(new GridLayout(1, 2));
JPanel jPanel2 = new JPanel(new GridLayout(2, 1));
JPanel jPanel3 = new JPanel(new GridLayout(2, 2));
JPanel jPanel4 = new JPanel(new GridLayout(3, 2));
jPanel1.add(new JButton("1-1"));
jPanel1.add(new JButton("1-2"));
jPanel2.add(new JButton("2-1"));
jPanel2.add(new JButton("2-2"));
for (int i = 0; i < 4; i++) {
jPanel3.add(new JButton("3-"+i));
}
for (int i = 0; i < 6; i++) {
jPanel4.add(new JButton("4-"+i));
}
container.add(jPanel1);
container.add(jPanel2);
container.add(jPanel3);
container.add(jPanel4);
setBounds(100,100,500,500);
setVisible(true);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
}
public static void main(String[] args) {
new JPanelDemo();
}
}
滚动条
import javax.swing.*;
import java.awt.*;
//测试窗口滚动条
public class JScrollDemo extends JFrame {
public JScrollDemo(){
Container container = getContentPane();
//文本域
JTextArea textArea = new JTextArea(10, 50);//10行50列的文本域
textArea.setText("这是测试的文本域");
//scroll面板
JScrollPane jScrollPane = new JScrollPane(textArea);
container.add(jScrollPane);
setBounds(100,100,200,200);
setVisible(true);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
}
public static void main(String[] args) {
new JScrollDemo();
}
}
3.5、按钮
- 图片按钮
import javax.swing.*;
import java.awt.*;
//图片按钮
public class IconJButton extends JFrame {
public IconJButton() throws HeadlessException {
Container container = getContentPane();
Icon icon = new ImageIcon(IconJButton.class.getResource("icon.png"));//创建一个图标
JButton button = new JButton("按钮");
button.setIcon(icon);//设置图标
button.setToolTipText("这是一个图片按钮");//鼠标悬浮提示
container.add(button);
setSize(500,500);
setVisible(true);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
}
public static void main(String[] args) {
new IconJButton();
}
}
- 单选按钮
import javax.swing.*;
import java.awt.*;
//测试单选按钮
public class RadioButton extends JFrame {
public RadioButton() throws HeadlessException {
Container container = getContentPane();
//单选按钮
JRadioButton radioButton1 = new JRadioButton("radioButton1");
JRadioButton radioButton2 = new JRadioButton("radioButton2");
JRadioButton radioButton3 = new JRadioButton("radioButton3");
//由于是单选框,就需要将按钮分组,一个组只能选择一个,如果不分组就所有按钮都可以选择
ButtonGroup buttonGroup = new ButtonGroup();
buttonGroup.add(radioButton1);
buttonGroup.add(radioButton2);
buttonGroup.add(radioButton3);
//添加按钮到容器中
container.add(radioButton1,BorderLayout.CENTER);
container.add(radioButton2,BorderLayout.NORTH);
container.add(radioButton3,BorderLayout.SOUTH);
setSize(500,500);
setVisible(true);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
}
public static void main(String[] args) {
new RadioButton();
}
}
- 复选按钮
import javax.swing.*;
import java.awt.*;
//测试多选框
public class Checkbox extends JFrame {
public Checkbox() throws HeadlessException {
Container container = getContentPane();
//多选框按钮
JCheckBox checkBox1 = new JCheckBox("checkBox1");
JCheckBox checkBox2 = new JCheckBox("checkBox2");
JCheckBox checkBox3 = new JCheckBox("checkBox3");
//添加按钮到容器中
container.add(checkBox1,BorderLayout.CENTER);
container.add(checkBox2,BorderLayout.NORTH);
container.add(checkBox3,BorderLayout.SOUTH);
setSize(500,500);
setVisible(true);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
}
public static void main(String[] args) {
new Checkbox();
}
}
3.6、列表
- 下拉框
import javax.swing.*;
import java.awt.*;
public class ComboBox extends JFrame {
public ComboBox() throws HeadlessException {
Container container = getContentPane();
JComboBox comboBox = new JComboBox();
comboBox.addItem(null);
comboBox.addItem("正在热映");
comboBox.addItem("已下架");
comboBox.addItem("正在制作");
container.add(comboBox);
setSize(500,500);
setVisible(true);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
}
public static void main(String[] args) {
new ComboBox();
}
}
- 列表框
import javax.swing.*;
import java.awt.*;
import java.util.Vector;
public class ListBox extends JFrame {
public ListBox() throws HeadlessException {
Container container = getContentPane();
String[] strings = {"1","2","3"};
Vector vector = new Vector();
vector.add("张三");
vector.add("王五");
vector.add("李四");
JList jList1 = new JList(strings);//创建列表框
JList jList2 = new JList(vector);//创建列表框
container.add(jList1,BorderLayout.WEST);
container.add(jList2,BorderLayout.EAST);
setSize(500,500);
setVisible(true);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
}
public static void main(String[] args) {
new ListBox();
}
}
- 应用场景
- 下拉框:选择地区,或者一些单个选项
- 列表框:展示信息,一般动态扩容
3.7、文本框
- 文本框
import javax.swing.*;
import java.awt.*;
public class TextBox extends JFrame {
public TextBox(){
Container container = getContentPane();
JTextField textField = new JTextField("文本框1",10);
JTextField textField1 = new JTextField("文本框2",20);
container.setLayout(new FlowLayout());
container.add(textField);
container.add(textField1);
setSize(500,500);
setVisible(true);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
}
public static void main(String[] args) {
new TextBox();
}
}
- 密码框
import javax.swing.*;
import java.awt.*;
public class PasswordBox extends JFrame {
public PasswordBox(){
Container container = getContentPane();
JPasswordField passwordField = new JPasswordField("密码框",20);
container.setLayout(new FlowLayout());
container.add(passwordField);
setSize(500,500);
setVisible(true);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
}
public static void main(String[] args) {
new PasswordBox();
}
}
- 文本域
import javax.swing.*;
import java.awt.*;
public class TextArea extends JFrame {
public TextArea(){
Container container = getContentPane();
JTextArea textArea = new JTextArea(20,10);
JTextArea textArea1 = new JTextArea(20,10);
textArea.setText("文本域1");
textArea1.setText("文本域2");
container.setLayout(new FlowLayout());
container.add(textArea);
container.add(textArea1);
setSize(500,500);
setVisible(true);
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
}
public static void main(String[] args) {
new TextArea();
}
}
贪吃蛇
帧,如果时间片足够小,就是动画,一秒30帧,连起来就是动画,拆开就是静态的图片
StartGame.java文件
实现主窗口的类,游戏窗口可以改变大小,游戏界面也会自适应大小
升级版的狂神的贪吃蛇
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.ComponentAdapter;
import java.awt.event.ComponentEvent;
public class StartGame {
public StartGame() {
JFrame frame = new JFrame("贪吃蛇Plus");
GamePanel gamePanel = new GamePanel();
frame.add(gamePanel);//添加面板
//new Dialog();
frame.addComponentListener(new ComponentAdapter() {//监听窗口大小改变事件
@Override
public void componentResized(ComponentEvent e) {
super.componentResized(e);
JFrame component = (JFrame) e.getComponent();
System.out.println("窗口位置改变");
gamePanel.setHeight(component.getSize().height);//动态调整游戏窗口
gamePanel.setWidth(component.getSize().width);
}
});
frame.setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
frame.setLocation(500,100);
frame.setSize(920,850);//窗口会缩水高缩50,宽缩20
//frame.setResizable(false);
frame.setVisible(true);
}
public static void main(String[] args) {
new StartGame();
}
}
GamePanel.java文件
游戏画笔和功能类
package src.GuiProject.SnakePlus;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.Random;
//画笔
public class GamePanel extends JPanel implements KeyListener, ActionListener {
private int height=800;//窗口大小
private int width=900;
private int fontSize;//显示字体大小
protected int[] bodyX = new int[500];//身体坐标
protected int[] bodyY = new int[500];
private int foodX;//食物坐标
private int foodY;
private int length;//蛇的长度
private int score;//积分
private int speed;//游戏速度
private String direction;//蛇头朝向
private Boolean start;//开始标志
private Boolean defeated;//失败标志
private Boolean triumph;//获胜标志
protected Timer timer = new Timer(100,this);//定时器
protected Random random = new Random();//随机数
protected Material material = new Material();//素材
public GamePanel(){
init();
this.setFocusable(true);//获得焦点事件
this.addKeyListener(this);//添加键盘监听事件
}
public void init(){
bodyX[0]=100;bodyY[0]=50;//身体坐标
bodyX[1]=75;bodyY[1]=50;
bodyX[2]=50;bodyY[2]=50;
length=3; //长度
foodX=25*random.nextInt((width/25)-1);//食物坐标
foodY=25*random.nextInt((height/25)-1);
direction="R";//蛇头朝向右
start=false;//游戏不开始
defeated=false;//游戏不失败
triumph=false;//游戏不获胜
score=0;//积分
fontSize=40;//设置字体大小
speed=200;//游戏速度
timer.setDelay(speed);//设置定时器
timer.start();//开启定时器
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
material.g=g;
//设置画布颜色和大小
g.setColor(Color.pink);
g.fillRect(0,0,width,height);
painting();//画蛇的身体
//画获得积分和蛇的长度
material.writeString("长度:"+length,Math.max(width-100,0),fontSize,fontSize/2,Color.white);
material.writeString("积分:"+score,Math.max(width-100,0),fontSize*2,fontSize/2,Color.white);
material.writeString("食物坐标:"+foodX+","+foodY,Math.max(width-200,0),fontSize*3,fontSize/2,Color.white);
material.writeString("小蛇坐标:"+bodyX[0]+","+bodyY[0],Math.max(width-200,0),fontSize*4,fontSize/2,Color.white);
//画提示语
if (!start){
material.writeString("按下空格开始游戏",(width-fontSize*8)/2,(height-fontSize)/2,fontSize,Color.white);
}else if (defeated) {
material.writeString("游戏失败,按下空格重新开始",(width-fontSize*12)/2,(height-fontSize)/2,fontSize,Color.red);
}
else if(triumph){
material.writeString("恭喜你达到了最大长度!",(width-fontSize*10)/2,(height-fontSize)/2-20,fontSize,Color.RED);
material.writeString("长度:"+length+" 积分:"+score,(width-fontSize*8)/2,(height-fontSize)/2+20,fontSize,Color.RED);
}
}
/******************画蛇的身体******************************************/
private void painting(){
material.food(foodX,foodY);
material.DrawSnake(bodyX[0],bodyY[0],direction);//画蛇头
for (int i = 1; i < length; i++) {
material.body(bodyX[i],bodyY[i]);
}
}
/******************监听事件******************************************/
@Override
public void actionPerformed(ActionEvent e) {
//--------------------判断吃食物------刷新食物的位置----------------------
if(bodyX[0]==foodX&&bodyY[0]==foodY){
foodX=25*random.nextInt((width/25)-1);//食物坐标
foodY=25*random.nextInt((height/25)-1);
length++;
score+=10;//加积分
//--------------重新设置定时器时间--------------------
if(score%100==0) {//吃10个食物加快一次速度
speed-=5;
timer.setDelay(speed);//设置定时器
}
///----------长度到达最大值获得胜利-------------
if(length>=(width*height/25)-1){
triumph=true;
}
}
//--------------判断失败--------------------------
for (int i = 1; i <length ; i++) {
if(bodyX[0]==bodyX[i]&&bodyY[0]==bodyY[i]){
defeated=true;
break;
}
}
//------------------刷新身体的位置-----------------------
if(start&&!defeated&&!triumph){
for (int i = length-1; i > 0; i--) {
bodyX[i]=bodyX[i-1];
bodyY[i]=bodyY[i-1];
}
switch (direction){
case "R":bodyX[0]+=25;if(bodyX[0]>(width-25))bodyX[0]=0; break;
case "L":bodyX[0]-=25;if(bodyX[0]<0)bodyX[0]=width-25; break;
case "D":bodyY[0]+=25;if(bodyY[0]>height-25)bodyY[0]=0; break;
case "U":bodyY[0]-=25;if(bodyY[0]<0)bodyY[0]=height-25; break;
}
if(bodyX[0]%25!=0)bodyX[0]+=25-(bodyX[0]%25);//修正位置
if(bodyY[0]%25!=0)bodyY[0]+=25-(bodyY[0]%25);
}
repaint();//重画画面
timer.start();
}
/****************************键盘监听******************************************/
@Override
public void keyPressed(KeyEvent e) {
int keyCode = e.getKeyCode();
if((!start||defeated||triumph)&&keyCode!=KeyEvent.VK_SPACE)keyCode=0;//避免游戏停止时误触
switch (keyCode){
case KeyEvent.VK_SPACE://空格
if(defeated){
defeated=false;
init();
start=true;
}else start=!start;
break;
case KeyEvent.VK_RIGHT:
if(!direction.equals("L"))direction="R";
break;
case KeyEvent.VK_LEFT:
if(!direction.equals("R"))direction="L";
break;
case KeyEvent.VK_DOWN:
if(!direction.equals("U"))direction="D";
break;
case KeyEvent.VK_UP:
if(!direction.equals("D"))direction="U";
break;
}
}
@Override
public void keyTyped(KeyEvent e) {
}
@Override
public void keyReleased(KeyEvent e) {
}
public void setHeight(int height) {
this.height = height-50;
if(this.height<foodY+25){//改变窗口导致食物在窗口外,就刷新食物位置
foodY=25*random.nextInt((width/25)-1);//食物坐标
repaint();//重画画面
}
}
public void setWidth(int width) {
this.width = width-20;
if(this.width<foodX+25){//改变窗口导致食物在窗口外,就刷新食物位置
foodX=25*random.nextInt((width/25)-1);//食物坐标
repaint();//重画画面
}
}
}
Material.java文件
游戏的资源类,所有东西都是画出来的,没有外部资源
package src.GuiProject.SnakePlus;
import java.awt.*;
public class Material {
Graphics g;
private int[][] rightLocation = new int[][]{
{0,0,12,25},
{0,0,25,25},
{10,15,10,10},
{10,0,10,10},
{12,17,5,5},
{12,2,5,5}
};
private int[][] leftLocation = new int[][]{
{13,0,12,25},
{0,0,25,25},
{4,15,10,10},
{4,0,10,10},
{6,17,5,5},
{6,2,5,5}
};
private int[][] upLocation = new int[][]{
{0,13,25,12},
{0,0,25,25},
{14,3,10,10},
{1,3,10,10},
{16,5,5,5},
{3,5,5,5}
};
private int[][] downLocation = new int[][]{
{0,0,25,12},
{0,0,25,25},
{14,10,10,10},
{1,10,10,10},
{16,12,5,5},
{3,12,5,5}
};
private Color[] color = new Color[]{Color.red,Color.white,Color.black};
private void painting(int x,int y,int[][] paint){
for (int i = 0; i < 6; i++) {
if (i % 2 == 0) g.setColor(color[i / 2]);
if (i==0)g.fillRect(paint[i][0]+x, paint[i][1]+y, paint[i][2], paint[i][3]);
else g.fillOval(paint[i][0]+x, paint[i][1]+y, paint[i][2], paint[i][3]);
}
}
public void left(int x,int y){
painting(x,y,leftLocation);
}
public void right(int x,int y){
painting(x,y,rightLocation);
}
public void up(int x,int y){
painting(x,y,upLocation);
}
public void down(int x,int y){
painting(x,y,downLocation);
}
public void body(int x,int y){
g.setColor(Color.green);
g.fillRect(x, y, 23, 23);
}
public void food(int x,int y){
g.setColor(Color.YELLOW);
g.fillOval(x, y, 25, 25);
}
public void DrawSnake(int x,int y,String direction){//画带方向的蛇头
switch (direction){
case "R": painting(x,y,rightLocation); break;
case "L": painting(x,y,leftLocation); break;
case "D": painting(x,y,downLocation); break;
case "U": painting(x,y,upLocation); break;
}
}
public void writeString(String text,int x,int y,int size,Color color){
if (x<0)x=0;
if (y<0)y=0;
g.setColor(color);
g.setFont(new Font("微软雅黑",Font.BOLD,size));
g.drawString(text,x,y);
}
}