4.4 流操作
List<Integer> numbers = Arrays.asList(1, 2, 1, 3, 3, 2, 4);
numbers.stream().filter(i ->i % 2 == 0)
.distinct();
List<Integer> collect = numbers.stream()
.filter((i) -> i == 5)
.limit(3)
.collect(Collectors.toList());
List<String> words = Arrays.asList("java8","lambdas","in","action");
List<Integer> wordLengths = words.stream()
.map((s)->s.substring(0,1))
.map(String::length)
.collect(Collectors.toList());
wordLengths.stream().forEach(System.out::print);
List<String[]> collect1 =
words.stream()
.map(w -> w.split(""))
.distinct()
.collect(Collectors.toList());
words.stream().map(w->w.split(""))
.flatMap(Arrays::stream)
.distinct()
.collect(Collectors.toList());
if(words.stream().anyMatch(s->s.length() >5)){
}
if(words.stream().allMatch(s->s.length() > 4)){
}
if(words.stream().noneMatch(s->s.length() > 5)){
}
Optional<String> any = words.stream()
.filter(s -> s.length() > 5).findAny();
Optional<Integer> first = words.stream().map(String::length).findFirst();
Integer reduce = collect2.stream().reduce(0, (a, b) -> a + b);
Integer reduce1 = collect2.stream().reduce(0, Integer::sum);
Optional<Integer> reduce2 = collect2.stream().reduce(Integer::max);
Optional<Integer> reduce3 = collect2.stream().reduce(Integer::min);
IntSummaryStatistics collect1 = apples.stream().collect(summarizingInt(Apple::getWeight));
double average = collect1.getAverage();
int max = collect1.getMax();
String d = apples.stream().map(Apple::getColor).collect(joining(","));
Integer sumApple = apples.stream().map(Apple::getWeight).reduce(0, (a, b) -> a + b);
apples.stream().collect(reducing(0,Apple::getWeight,Integer::sum));
apples.stream().map(Apple::getWeight).reduce(Integer::sum).get();
apples.stream().mapToInt(Apple::getWeight).sum();
Map<String, Long> collect4 = apples.stream().collect(groupingBy(Apple::getColor,counting()));
Map<String, Optional<Apple>> collect5 = apples.stream().collect(groupingBy(Apple::getColor,maxBy(Comparator.comparingInt(Apple::getWeight))));
Map<String, Apple> collect6 = apples.stream()
.collect(groupingBy(Apple::getColor, collectingAndThen(maxBy(Comparator.comparingInt(Apple::getWeight)), Optional::get)));
Map<String, HashSet<Boolean>> collect7 = apples.stream().collect(groupingBy(Apple::getColor, mapping(a -> {
if (a.getWeight() > 100) {
return true;
}
return false;
}, toCollection(HashSet::new))));
Map<Boolean, List<Dish>> collect8 = menus.stream().collect(partitioningBy(Dish::isVegetarian));
Map<Boolean, Map<Dish.Type, List<Dish>>> collect9 = menus.stream()
.collect(partitioningBy(Dish::isVegetarian, groupingBy(Dish::getType)));
Map<Boolean, Dish> collect10 = menus.stream()
.collect(partitioningBy(Dish::isVegetarian, collectingAndThen(maxBy(Comparator.comparingInt(Dish::getCalories)), Optional::get)));
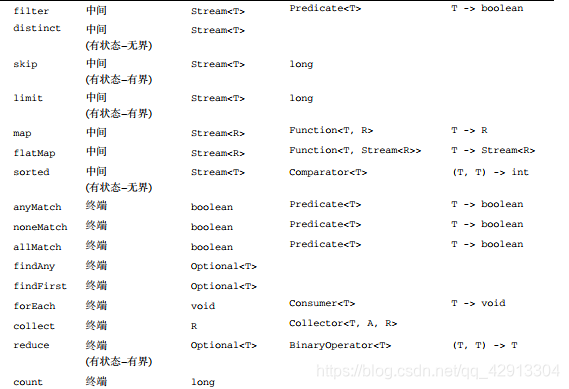
4.5 构建流
Stream<String> java = Stream.of("java", "demo");
int[] numbers = {1,2,3,45,5};
IntStream stream = Arrays.stream(numbers);
Stream<Integer> boxed1 = stream.boxed();