集合
结构
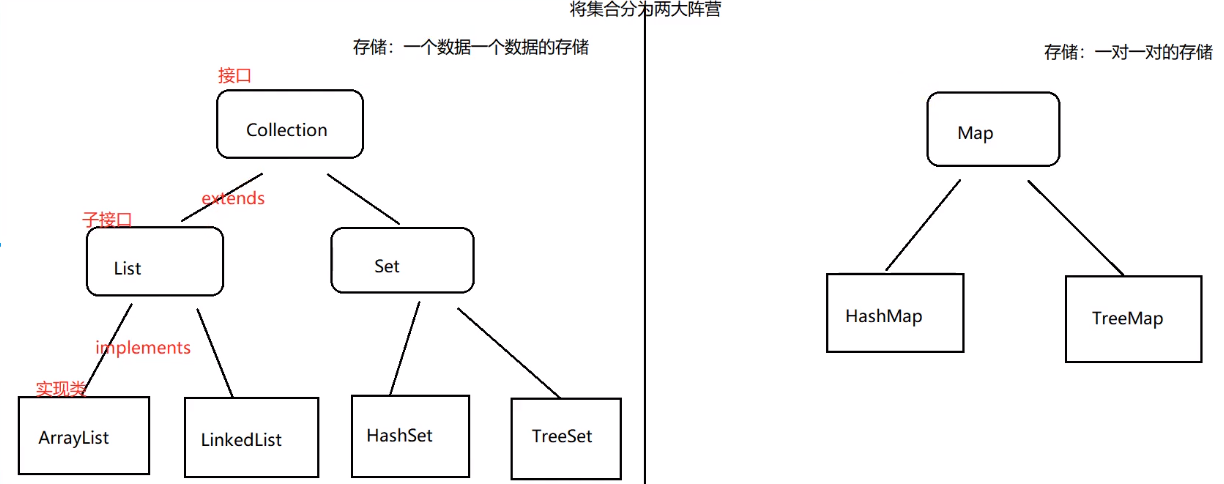
一、Collection(接口)
常用方法
- 增加:add(E e) addAll(Collection<? extends E> c)
泛型受限,定义上界 - 删除:clear() remove(Object o)
- 修改:
- 查看:iterator() size()
- 判断:contains(Object o) equals(Object o) isEmpty()
//创建对象:接口不能创建对象,利用实现类创建对象:
Collection col = new ArrayList();
//调用方法:
//集合有一个特点:只能存放引用数据类型的数据,不能是基本数据类型
//基本数据类型自动装箱,对应包装类。int--->Integer
col.add(18);
col.add(12);
col.add(11);
col.add(17);
System.out.println(col/*.toString()*/);
List list = Arrays.asList(new Integer[]{11, 15, 3, 7, 1});
col.addAll(list);//将另一个集合添加入col中
System.out.println(col);
//col.clear();清空集合
System.out.println(col);
System.out.println("集合中元素的数量为:"+col.size());
System.out.println("集合是否为空:"+col.isEmpty());
boolean isRemove = col.remove(15);//移除对象
System.out.println(col);
System.out.println("集合中数据是否被删除:"+isRemove);
Collection col2 = new ArrayList();
col2.add(18);
col2.add(12);
col2.add(11);
col2.add(17);
Collection col3 = new ArrayList();
col3.add(18);
col3.add(12);
col3.add(11);
col3.add(17);
System.out.println(col2.equals(col3));
System.out.println(col2==col3);//地址一定不相等 false
System.out.println("是否包含元素:"+col3.contains(117));
Collection集合的遍历
-
增强for循环
for(Object o:col){ System.out.println(o); }
-
iterator()迭代器
Iterator it = col.iterator(); while(it.hasNext()){ System.out.println(it.next()); }
- 迭代器拓展
- 通过hasNext()来判断是否有下一个元素,如果有下一个元素,那么返回true;如果没有下一个元素,那么返回false。
- next()方法将元素获取到,并且将“指针”下移。
- 迭代器拓展
List接口
总结:
- 有序可重复
- 非线程安全
常用方法
继承,拥有父接口Collection的全部方法
-
增加:add(int index, E element)
-
删除:remove(int index) remove(Object o)
-
修改:set(int index, E element)
-
查看:get(int index)
-
判断:
List list = new ArrayList(); list.add(13); list.add(17); list.add(6); list.add(-1); list.add(2); list.add("abc"); System.out.println(list); list.add(3,66); System.out.println(list); list.set(3,77); System.out.println(list); list.remove(2);//在集合中存入的是Integer类型数据的时候,调用remove方法调用的是:remove(int index) System.out.println(list); list.remove("abc"); System.out.println(list); Object o = list.get(0); System.out.println(o);
List集合的遍历
-
普通for循环:
for(int i = 0;i<list.size();i++){ System.out.println(list.get(i)); }
-
增强for循环:
for(Object obj:list){ System.out.println(obj); }
-
迭代器:
Iterator it = list.iterator(); while(it.hasNext()){ System.out.println(it.next()); }
ArrayList实现类(JDK 1.7)
-
底层重要属性
- private transient Object[] elementData;底层的数组类型Object类型
- private int size;数组中有效数据的个数
-
在JDK1.7中:在调用构造器的时候给底层数组elementData初始化,数组初始化长度为10:
public ArrayList(int initialcapacity){ super(); if (initialCapacity < ) throw new IllegalArgumentException("Illegal Capacity:"+initialCapacity); this.elementData=new Object[initialCapacity];
-
调用add()方法
public boolean add(E e){//调用add方法向底层数组中添加元素 ensureCapacityInternal(minCapacity:size+1);//确保数组空间有剩余 elementData[size++]=e;//最开始size为0,添加元素以后size+1操作 return true;//添加成功返回true
数组中的10个位置都满了的时候就开始进行数组的扩容,扩容长度为原数组的1.5倍:
public boolean add(E e){ ensureCapacityInternal(minCapacity:size+1);//调用ensureCapacityInternal()函数,函数参数为11 elementData[size++]=e; return true;
private void ensureCapacityInternal(int mincapacity){//参数大小为11 if(minCapadity-elementData.length>0)//minCapadity为11,elementData.length为10,条件成立 grow(minCapacity);//调用grow()函数,参数为11,扩容
private void grow(int minCapadity){//参数大小为11 // overflow-conscious code int oldCapadity=elementData.length; int newCapacity = oldCapacity + (oldCapacity >> 1);//扩容1.5倍 elementData = Arrays.copyof(elementData, newCapacity);//将elementData的指向从老数组变为新数组:扩容的本质 //将老数组中的内容复制到新数组中,然后返回新数组
ArrayList实现类(JDK 1.8)
-
底层重要属性,
和JDK 1.7保持一致: Object[] elementData int size
-
区别
调用空构造器时,创建空数组,默认长度为0;调用add()方法时,才会分配默认空间为10(default_size赋值),之后每次达到阈值时扩容1.5倍。