title: FastAPI中实现动态条件必填字段的实践
date: 2025/04/03 00:06:20
updated: 2025/04/03 00:06:20
author: cmdragon
excerpt:
在 FastAPI 中,使用 Pydantic 模型实现动态条件必填字段时,需结合 Field
的 depends
参数、@model_validator(mode='before')
装饰器和条件判断逻辑。例如,用户注册接口根据 register_type
动态决定 email
或 mobile
字段是否必填,并在 accept_promotion=True
时要求至少填写一种联系方式。通过 @model_validator
在类型转换前验证字段值,确保数据符合条件。测试用例和常见报错解决方案帮助调试和优化验证逻辑。
categories:
- 后端开发
- FastAPI
tags:
- Pydantic
- FastAPI
- 动态必填字段
- 数据验证
- 用户注册
- 模型验证器
- 422错误处理
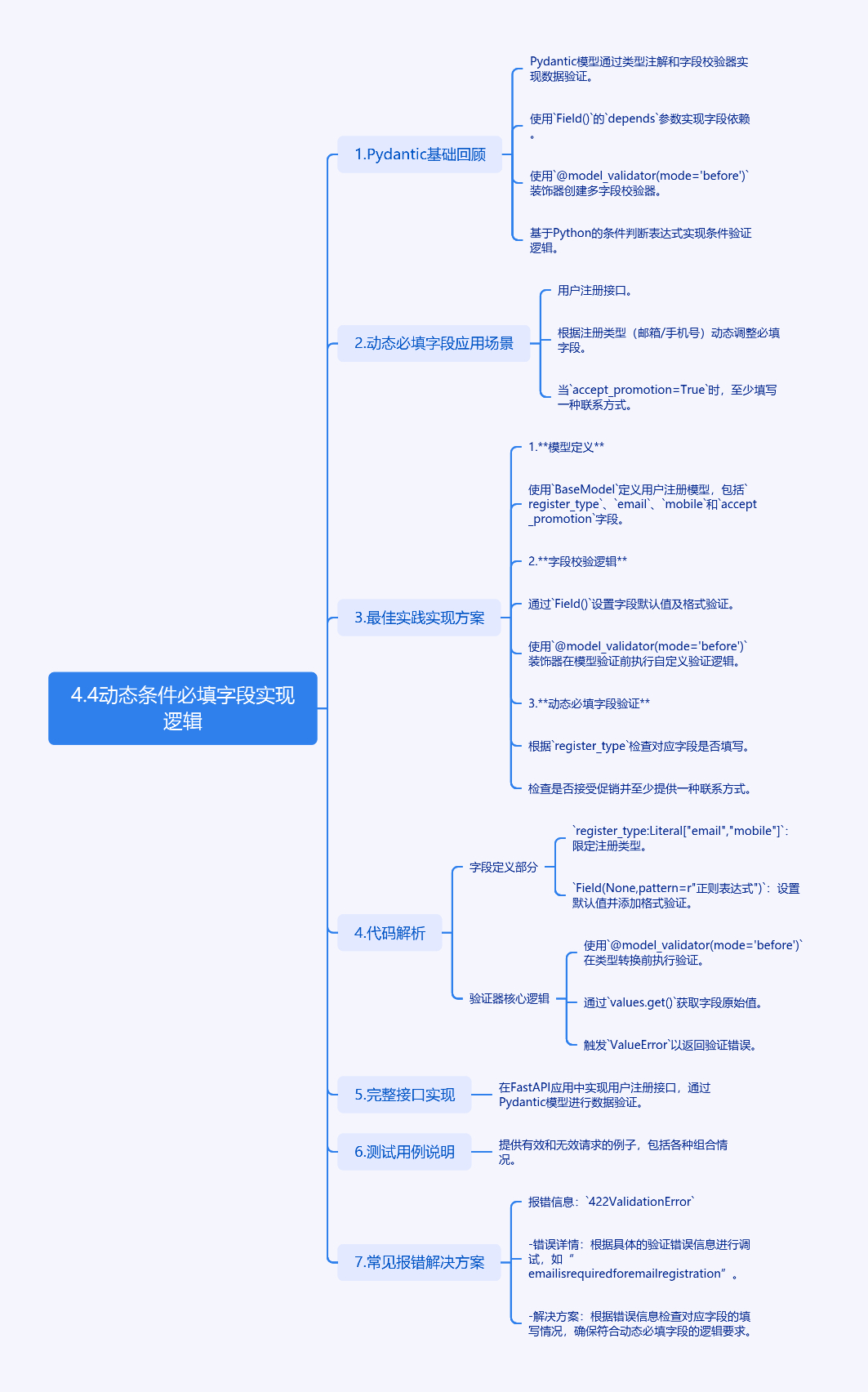

扫描二维码关注或者微信搜一搜:编程智域 前端至全栈交流与成长
1. Pydantic 基础回顾
在 FastAPI 框架中,Pydantic
模型通过类型注解和字段校验器(validators)实现数据验证。当我们需要实现根据某个字段的值动态决定其他字段是否必填
时,需要组合使用以下特性:
- Field 依赖声明:使用
Field()
的depends
参数 - 多字段校验器:
@model_validator(mode='before')
装饰器 - 条件验证逻辑:基于 Python 的条件判断表达式
2. 动态必填字段应用场景
假设我们需要开发一个用户注册接口,根据不同的注册类型(邮箱/手机号)动态调整必填字段:
- 当
register_type=email
时,email
字段必填 - 当
register_type=mobile
时,mobile
字段必填 - 当
accept_promotion=True
时,必须填写至少一种联系方式
3. 最佳实践实现方案
from pydantic import BaseModel, Field, model_validator
from typing import Optional, Literal
class UserRegistration(BaseModel):
register_type: Literal["email", "mobile"] # 限定注册类型枚举值
email: Optional[str] = Field(None, pattern=r"^[\w\.-]+@[\w\.-]+\.\w+$")
mobile: Optional[str] = Field(None, pattern=r"^1[3-9]\d{9}$")
accept_promotion: bool = False
@model_validator(mode