一、String:
1. 概念:
String:表示字符串:
字符串是常量;它们的值在创建之后不能更改。
String是一种特殊的引用类型:
默认值:null
字符串是常量;它们的值在创建之后不能更改。
String是一种特殊的引用类型:
默认值:null
2.构造方法:
String():无参构造
String(byte[] bytes) :将字节数转换成字符串;
public String(byte[] bytes, int index,int length):将字节数组的一部分转换成字符串;
public String(char[] value):将字符数组转化成字符串 ; toCharArray():将字符串转换成字符;
public String(char[] value, int index, int count):将字符数组的一部分转换成字符串;
public String(String original):将一个字符串常量构造成一个字符串对象。
String(byte[] bytes) :将字节数转换成字符串;
public String(byte[] bytes, int index,int length):将字节数组的一部分转换成字符串;
public String(char[] value):将字符数组转化成字符串 ; toCharArray():将字符串转换成字符;
public String(char[] value, int index, int count):将字符数组的一部分转换成字符串;
public String(String original):将一个字符串常量构造成一个字符串对象。
3.最大特点:
字符串一旦被赋值,其值不能被改变。
4.常用的判断功能:
boolean equals(Object obj):将此字符串与指定的对象比较;
boolean equalsIgnoreCase(String str)将此 String 与另一个 String 比较,不考虑大小写;
boolean contains(String str):判断当前大川中是否包含子字符串 (重点);
boolean startsWith(String str):以当前str字符串开头(重点);
boolean endsWith(String str):以当前str字符串结尾(重点);
boolean isEmpty():判断字符串是否为空。
boolean equalsIgnoreCase(String str)将此 String 与另一个 String 比较,不考虑大小写;
boolean contains(String str):判断当前大川中是否包含子字符串 (重点);
boolean startsWith(String str):以当前str字符串开头(重点);
boolean endsWith(String str):以当前str字符串结尾(重点);
boolean isEmpty():判断字符串是否为空。
5.常用的获取功能:
public int length():获取字符串的长度;
public char charAt(int index)返回指定索引处的 字符;
public int indexOf(int ch)返回指定字符在此字符串中第一次出现处的索引;
public int indexOf(int ch,int fromIndex)返回在此字符串中第一次出现指定字符处的索引,从指定的索引开始搜索。
public int indexOf(String str)返回指定子字符串在此字符串中第一次出现处的索引;
public int indexOf(String str,int fromIndex)回在此字符串中第一次出现指定字符串处的索引,从指定的索引开始搜索。
public char charAt(int index)返回指定索引处的 字符;
public int indexOf(int ch)返回指定字符在此字符串中第一次出现处的索引;
public int indexOf(int ch,int fromIndex)返回在此字符串中第一次出现指定字符处的索引,从指定的索引开始搜索。
public int indexOf(String str)返回指定子字符串在此字符串中第一次出现处的索引;
public int indexOf(String str,int fromIndex)回在此字符串中第一次出现指定字符串处的索引,从指定的索引开始搜索。
6.截取功能:
public String substring(int beginIndex):从指定位置开始截取,默认截取到末尾,返回新的字符串;
public String substring(int beginIndex, int endIndex):从指定位置开始到指定位置末尾结束,包前不包含.
public String substring(int beginIndex, int endIndex):从指定位置开始到指定位置末尾结束,包前不包含.

7.转换功能:
public byte[] getBytes() :将字符串转换为字节数组;
public char[] toCharArray() :将字符串转换成字符数组(重点);
public static String valueOf(int i):将int类型的数据转换成字符串(重点);
这个方法可以将任何类型的数据转化成String类型
public String toLowerCase():转成小写;
public String toUpperCase():字符串中所有的字符变成大写。

8.其他功能:
public String replace(char oldChar,char newChar):将大字符串中的某个字符替换掉成新的字符;
public String replace(String oldStr,String newStr):将大串中的某个子字符串替换掉;
public String trim():去除字符串两端空格;
public int compareTo(String anotherString)按字典顺序比较两个字符串。
public String replace(String oldStr,String newStr):将大串中的某个子字符串替换掉;
public String trim():去除字符串两端空格;
public int compareTo(String anotherString)按字典顺序比较两个字符串。
二、StringBuffer:
1.概念:
线程程安全的可变字符序列。
2.构造方法:
StringBuffer() :无参构造的形式,初始容量16;
StringBuffer(int capacity) :指定容量构造一个字符串缓冲区;
StringBuffer(String str) 构造一个字符串缓冲区,并将其内容初始化为指定的字符串内容.
StringBuffer(int capacity) :指定容量构造一个字符串缓冲区;
StringBuffer(String str) 构造一个字符串缓冲区,并将其内容初始化为指定的字符串内容.
3.面试题:
StringBuffer和String的区别?
答: 前者是一个可变的字符序列,后者是不可变的字符序列;
如果从内存角度考虑,String定义的时候,会在常量池中开辟空间,比较耗费内存;
而StringBuffer,字符串缓冲区(里面存储的全部都是字符串),它会释放掉。
答: 前者是一个可变的字符序列,后者是不可变的字符序列;
如果从内存角度考虑,String定义的时候,会在常量池中开辟空间,比较耗费内存;
而StringBuffer,字符串缓冲区(里面存储的全部都是字符串),它会释放掉。
4.获取功能:
public int length()返回长度;
public int capacity()返回当前容量 (如果超过容量,系统自动分配(存储字符串的时候,英文的))。
public int capacity()返回当前容量 (如果超过容量,系统自动分配(存储字符串的时候,英文的))。
5.功能:
1)添加功能:
public StringBuffer append(String/boolean....) :在字符串缓冲区中追加数据(在末尾追加),并且返回字符串缓冲区本身;
public StringBuffer insert(int offset,String str):将当前str字符串添加到指定位置处,它返回字符串缓冲区本身。
public StringBuffer insert(int offset,String str):将当前str字符串添加到指定位置处,它返回字符串缓冲区本身。

2)反转功能:
public StringBuffer reverse() :将缓冲区中的字符序列反转取代,返回它(字符串冲)本身。
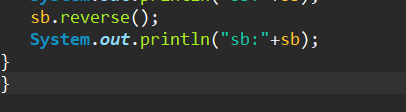
3)删除功能:
public StringBuffer deleteCharAt(int index):移除指定位置处的字符..
public StringBuffer delete(int start,int end):移除从指定位置处到end-1处的子字符串...
4)截取功能:
public StringBuffer delete(int start,int end):移除从指定位置处到end-1处的子字符串...

4)截取功能:
public String substring(int start):从指定位置开始截取,默认截取到末尾,返回值不在是缓冲区本身,而是一个新的字符串
public String substring(int start,int end) :从指定位置开始到指定位置结束截取,包前不包后,返回值不在是缓冲区本身,而是一个新的字符串。
public String substring(int start,int end) :从指定位置开始到指定位置结束截取,包前不包后,返回值不在是缓冲区本身,而是一个新的字符串。

5)替换功能:
public StringBuffer replace(int start,int end,String str)
从指定位置到指定位置结束,用新的str字符串去替换,返回值是字符串缓冲区本身。
从指定位置到指定位置结束,用新的str字符串去替换,返回值是字符串缓冲区本身。

/*
StringBuffer,String,StringBuilder的区别?
StringBuffer和StringBuilder都是一个可变的字符序列,提供一个缓冲区.(两者都看做容器)
StringBuffer:线程安全的,同步的,执行效率低
StringBuilder:线程不安全的,不同步的,执行效率高,并且单线程中优先采用StringBuilder
StringBuffer 执行效率虽然低,但是由于String类型,并且他可变的字符序列,提供了缓冲区
StringBuffer:线程安全的,同步的,执行效率低
StringBuilder:线程不安全的,不同步的,执行效率高,并且单线程中优先采用StringBuilder
StringBuffer 执行效率虽然低,但是由于String类型,并且他可变的字符序列,提供了缓冲区
*/
/*
StringBuffer和数组的区别?
数组:它是可以存储多个数据的容器,并且多个数据的类型必须一致
数组长度功能:length属性
StringBuffer:它是容器,它在缓冲区始终存储的只能字符串类型的数据
获取缓冲区的长度:length()
数组长度功能:length属性
StringBuffer:它是容器,它在缓冲区始终存储的只能字符串类型的数据
获取缓冲区的长度:length()
*/