package cn.lzc.day01.nio;
import java.net.InetAddress;
public class InterAddressTest {
public static void main(String[] args) {
try {
InetAddress inet1= InetAddress.getByName("192.168.1.1");
System.out.println(inet1);
InetAddress inet2=InetAddress.getByName("www.baidu.com");
System.out.println(inet2);
InetAddress inet3=InetAddress.getLocalHost();
System.out.println(inet3);
System.out.println(inet3.getHostAddress());
System.out.println(inet3.getHostName());
} catch (Exception e) {
e.printStackTrace();
}
}
}
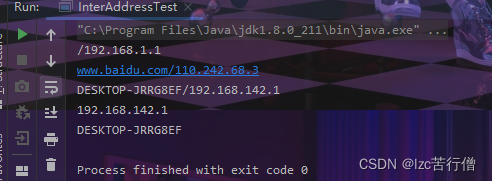
package cn.lzc.day01.nio;
import org.junit.Test;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.InetAddress;
import java.net.ServerSocket;
import java.net.Socket;
/**
* 实现TCP的网络编程
* 例子1:客户端发送信息给服务器,服务端将数据显示在控制台上
*/
public class TcpTest {
// 客户端
@Test
public void test() {
OutputStream os = null;
Socket socket = null;
try {
// 创建Socket对象,指明服务器端的ip和端口号
InetAddress inet = InetAddress.getByName("127.0.0.1");
// 获取一个输出流,用于输出数据
socket = new Socket(inet, 8899);
os = socket.getOutputStream();
// 写出数据的操作
os.write("你好,我是客户端luck".getBytes());
} catch (IOException e) {
e.printStackTrace();
} finally {
if (os != null)
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
if (socket != null)
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
// 服务端
@Test
public void test1() {
ServerSocket ss = null;
Socket socket = null;
InputStream is = null;
ByteArrayOutputStream baos = null;