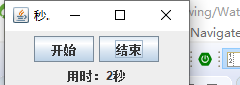
package com.swing;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Calendar;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.SwingUtilities;
public class Watch implements ActionListener{
JLabel jl;
long start=0;//必须定义为全局变量,如果定义在监听事件内部,每一次都会被刷新
Watch(){
JFrame jfame=new JFrame("秒表");
jfame.setLayout(new FlowLayout());
JButton start=new JButton("开始");
JButton end =new JButton("结束");
jl=new JLabel("点击开始按钮开始计时");
start.addActionListener(this);
end.addActionListener(this);
jfame.add(start);
jfame.add(end);
jfame.add(jl);
jfame.setSize(200, 200);
jfame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jfame.setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
// TODO Auto-generated method stub
Calendar cla=Calendar.getInstance();
if(e.getActionCommand().equals("开始")) {
start=cla.getTimeInMillis();
System.out.println(start);
jl.setText("正在计时。。。");
}
else {
jl.setText("用时:"+(cla.getTimeInMillis()-start)/1000+"秒");
System.out.println(cla.getTimeInMillis());
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
// TODO Auto-generated method stub
new Watch();
}
});
}
}