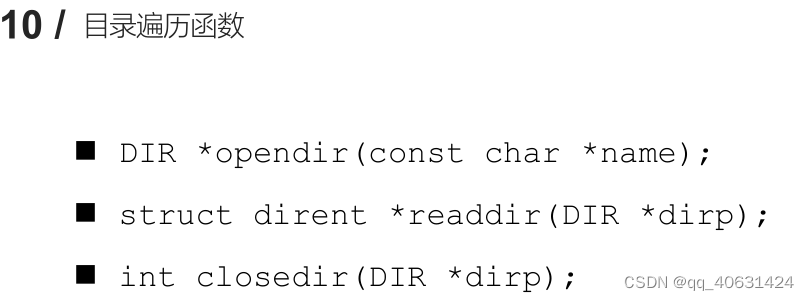
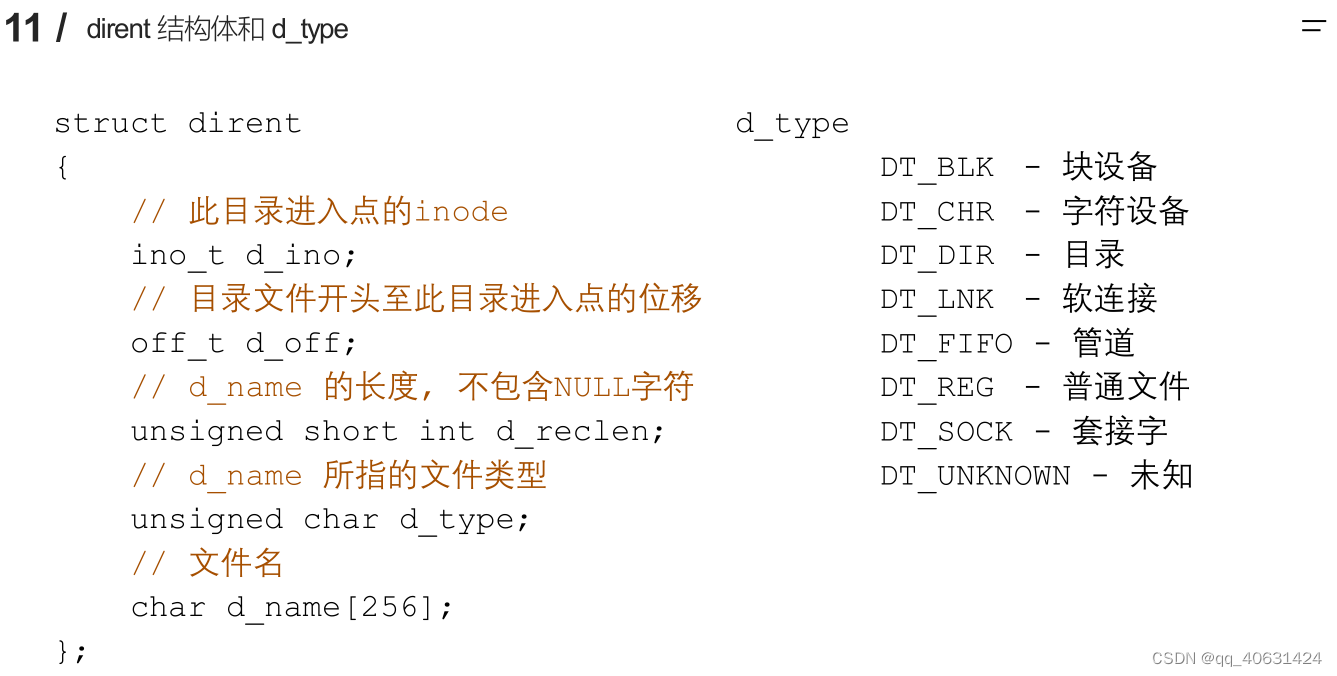
#include <sys/types.h>
#include <dirent.h>
DIR *opendir(const char *name);
参数:
- name: 需要打开的目录的名称
返回值:
DIR * 类型,理解为目录流
错误返回NULL
#include <dirent.h>
struct dirent *readdir(DIR *dirp);
- 参数:dirp是opendir返回的结果
- 返回值:
struct dirent,代表读取到的文件的信息
读取到了末尾或者失败了,返回NULL
#include <sys/types.h>
#include <dirent.h>
int closedir(DIR *dirp);
*/
#include <sys/types.h>
#include <dirent.h>
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int getFileNum(const char * path);
int main(int argc, char * argv[]){
if(argc < 2){
printf("%s path\n",argv[0]);
return -1;
}
int num = getFileNum(argv[1]);
printf("普通文件的个数为:%d\n", num);
return 0;
}
int getFileNum(const char * path){
DIR * dir = opendir(path);
if(dir == NULL){
perror("opendir");
exit(0);
}
struct dirent * ptr;
int total = 0;
while ((ptr = (readdir(dir))) != NULL)
{
char * dname = ptr->d_name;
if(strcmp(dname,".") == 0 || strcmp(dname,"..") == 0){
continue;
}
if(ptr->d_type == DT_DIR){
char newPath[256];
sprintf(newPath, "%s/%s", path, dname);
total += getFileNum(newPath);
}
if(ptr->d_type == DT_REG){
total++;
}
}
closedir(dir);
return total;
}