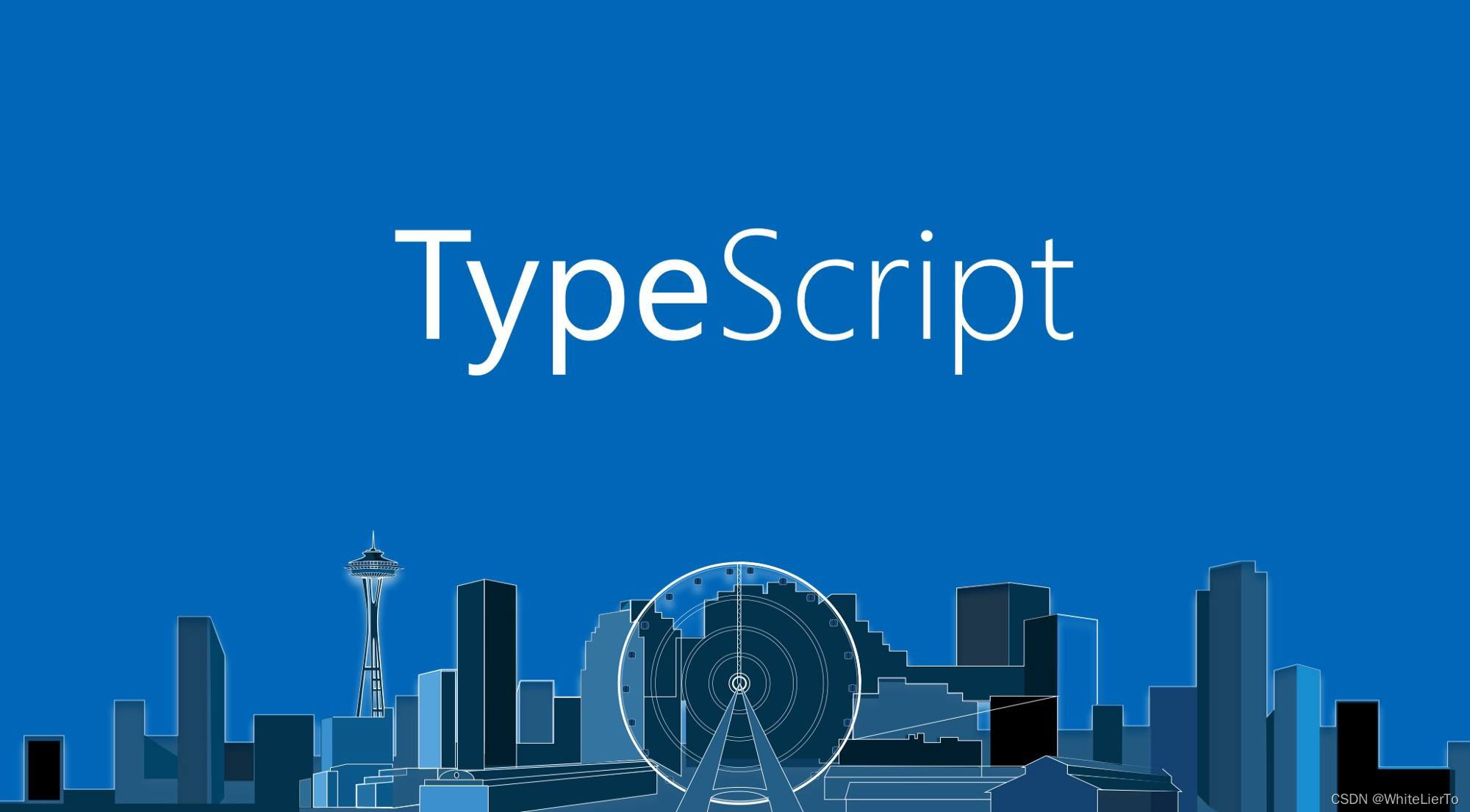
基本用法
// 普通形式
const getFullName = ({ firstName, lastName }: { firstName: string, lastName: string }) => {
return `${firstName} ${lastName}`
}
console.log(
getFullName({
firstName: 'hhh',
lastName: 'Li'
})
)
// 接口定义
interface NameInfo{
firstName:string,
lastName:string
}
const getFullName = ({ firstName, lastName }: NameInfo) => {
return `${firstName} ${lastName}`
}
console.log(
getFullName({
firstName: 'hhh',
lastName: 'Li'
})
)
可选属性
// color?:string ? 表示可选属性
interface Vegetable{
color?:string,
type:string,
}
const getVegetable = ({color,type}:Vegetable):string=>{
return `A ${color ? (color + ' ') : ''}${type} `
}
getVegetable({
type:'tomato',
})
绕开多余属性检查
const getVegetable = ({color,type}:Vegetable):string=>{
return `A ${color ? (color + ' ') : ''}${type} `
}
// 索引签名 [prop:string]:any
interface Vegetable{
color?:string,
readonly type:string,
[prop:string]:any
}
getVegetable({
color:'red',
type:'tomato',
size:2
})
// 或者使用类型断言 as Vegetable
getVegetable({
color:'red',
type:'tomato',
size:2
} as Vegetable)
// 或者使用类型兼容性
const vagetableInfo = {
color:'red',
type:'tomato',
size:2
}
getVegetable(vagetableInfo)
只读属性
// readonly 只读属性
interface Vegetable{
color?:string,
readonly type:string,
}
let vagetableObj : Vegetable={
color:'red',
type:'tomato',
}
vagetableObj.type='carrot' // 报错
函数类型
type AddFnc = (num1:number,num2:number) =>number
const add: AddFnc=(n1,n2)=>n1+n2
索引类型
// 索引类型
interface RoleDic{
[id:number]:string
}
const role1:RoleDic={
0:'super_admin'
}
// 如果属性名是字符串类型,即使设置属性名为数字,也是可以的,因为会被转成string类型
interface RoleDic{
[id:string]:string
}
const role2:RoleDic={
a:'admin'
0:'super_admin'
}
继承接口
// 接口继承
interface Vegetables{
color:string
}
interface Tomato extends Vegetables{
radius:number
}
interface Carrot{
length:number
}
const tomato:Tomato={
radius:1,
color:'red'
}
const carrot:Carrot={
length:2
}
混合类型接口
// 混合类型接口
interface Counter{
():void,
count:number
}
const getCount =():Counter=>{
const c =()=>{c.count++}
c.count=0
return c
}
const counter :Counter=getCount()