服务层结构
需要实现的功能效果图
1. Cms系统实现
1.1. 内容分类管理
1.1.1. 展示内容分类
功能分析(查询内容节点)
请求的url:/content/category/list
请求的参数:id,当前节点的id。第一次请求是没有参数,需要给默认值“0”
响应数据:List<EasyUITreeNode>(@ResponseBody)
Json数据。
[{id:1,text:节点名称,state:open(closed)},
{id:2,text:节点名称2,state:open(closed)},
{id:3,text:节点名称3,state:open(closed)}]
业务逻辑:
1、取查询参数id,parentId
2、根据parentId查询tb_content_category,查询子节点列表。
3、得到List<TbContentCategory>
4、把列表转换成List<EasyUITreeNode>
展示内容节点内容
ContentCategoryServiceImpl.java
@Override public List<EasyUITreeNode> getContentCatList(long parentId) { // 根据parentid查询子节点列表 TbContentCategoryExample example = new TbContentCategoryExample(); TbContentCategoryExample.Criteria criteria = example.createCriteria(); //设置查询条件 criteria.andParentIdEqualTo(parentId); //执行查询 List<TbContentCategory> catList = contentCategoryMapper.selectByExample(example); //转换成EasyUITreeNode的列表 List<EasyUITreeNode> nodeList = new ArrayList<>(); for (TbContentCategory tbContentCategory : catList) { EasyUITreeNode node = new EasyUITreeNode(); node.setId(tbContentCategory.getId()); node.setText(tbContentCategory.getName()); node.setState(tbContentCategory.getIsParent()?"closed":"open"); //添加到列表 nodeList.add(node); } return nodeList; }
application-context.xml配置发布服务
<!-- 使用dubbo发布服务 --> <!-- 提供方应用信息,用于计算依赖关系 --> <dubbo:application name="e3-content" /> <dubbo:registry protocol="zookeeper" address="192.168.25.128:2181" /> <!-- 用dubbo协议在20880端口暴露服务 --> <dubbo:protocol name="dubbo" port="20881" /> <!-- 声明需要暴露的服务接口 --> <dubbo:service interface="com.e3mall.content.service.ContentCategoryService" ref="contentCategoryServiceImpl" timeout="600000"/>
ContentCatController.java表示层实现代码
/** * 内容分类异步加载 * @param parentId 第一次访问时需要给默认值 0 * @return * */ @RequestMapping("/content/category/list") @ResponseBody public List<EasyUITreeNode> getContentCatList(@RequestParam(name="id", defaultValue="0")Long parentId) { List<EasyUITreeNode> list = contentCategoryService.getContentCatList(parentId); return list; }
springmvc.xml引用服务
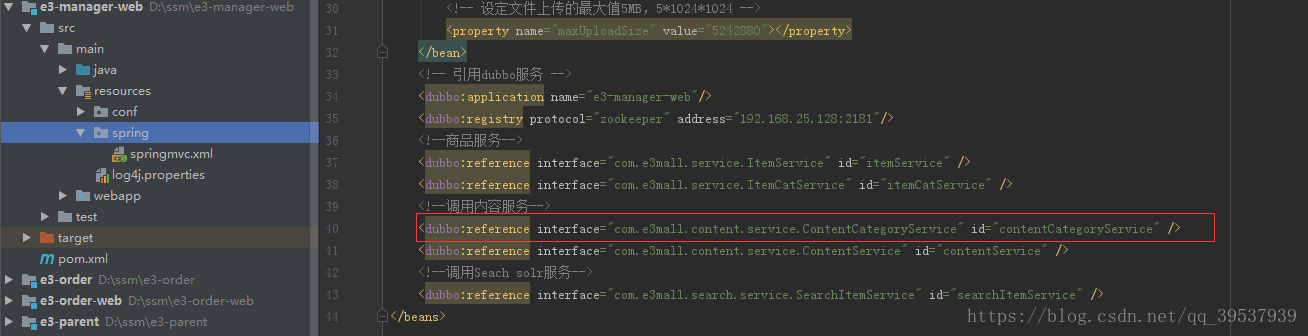
1.1.1. 新增节点
功能分析
请求的url:/content/category/create
请求的参数:
Long parentId
String name
响应的结果:
json数据,E3Result,其中包含一个对象,对象有id属性,新生产的内容分类id
业务逻辑:
1、接收两个参数:parentId、name
2、向tb_content_category表中插入数据。
a) 创建一个TbContentCategory对象
b) 补全TbContentCategory对象的属性
c) 向tb_content_category表中插入数据
3、判断父节点的isparent是否为true,不是true需要改为true。
4、需要主键返回。
5、返回E3Result,其中包装TbContentCategory对象
ContentCategoryServiceImpl.java代码
/** * 添加内容节点信息 * @param parentId * @param name * @return */
@Override public E3Result addContentCategory(long parentId, String name) { //创建tb_content_category表对应pojo对象 TbContentCategory tbContentCategory = new TbContentCategory(); //设置添加pojo对象 tbContentCategory.setParentId(parentId); tbContentCategory.setName(name); //1(正常)2(删除) tbContentCategory.setStatus(1); //排列序号,表示同级类目的展现次序,如数值相等则按名称次序排列。取值范围:大于零的整数 tbContentCategory.setSortOrder(1); //新添加的节点一定是叶子节点 tbContentCategory.setIsParent(false); tbContentCategory.setUpdated(new Date()); tbContentCategory.setCreated(new Date()); //插入到数据库 contentCategoryMapper.insert(tbContentCategory); //判断父节点的isParent属性是否为true如果不是就修改为true TbContentCategory parent = contentCategoryMapper.selectByPrimaryKey(parentId); if (!parent.getIsParent()){ parent.setIsParent(true); //更新到数据库 contentCategoryMapper.updateByPrimaryKey(parent); } //返回结果,返回E3Result,包含pojo return E3Result.ok(tbContentCategory); }
ContentCatController.java
表现层
请求的url:/content/category/create
请求的参数:
Long parentId
String name
响应的结果:
json数据,E3Result
/** * 添加节点 * @param parentId 父节点 * @param name 节点名 * @return */ @RequestMapping(value = "/content/category/create",method=RequestMethod.POST) @ResponseBody public E3Result createContentCategory(Long parentId,String name){ //调用服务添加节点 E3Result result = contentCategoryService.addContentCategory(parentId, name); return result; }
重命名
请求的url:/content/category/update
参数:id,当前节点id。name,重命名后的名称。
业务逻辑:根据id更新记录。
返回值:返回E3Result.ok()
代码实现
Service实现层
/** * 修改内容节点信息 * @param id * @param name * @return */ @Override public E3Result editContentCategoryName(Long id, String name) { //通过id查询 TbContentCategory tbContentCategory = contentCategoryMapper.selectByPrimaryKey(id); //设置查询到的内容节点名称为修改名称 tbContentCategory.setName(name); //执行修改 contentCategoryMapper.updateByPrimaryKey(tbContentCategory); //返回状态 return E3Result.ok(); }
表现层
/** * 重命名节点名 * @param id 节点id * @param name 节点名 * @return */ @RequestMapping(value = "/content/category/update") @ResponseBody public E3Result editContentCategoryName(Long id ,String name){ //调用服务重命名节点 E3Result result= contentCategoryService.editContentCategoryName(id,name); return result; }
删除
请求的url:/content/category/delete/
参数:id,当前节点的id。
响应的数据:json。E3Result。
业务逻辑:
1、根据id删除记录。
2、判断父节点下是否还有子节点,如果没有需要把父节点的isparent改为false
3、如果删除的是父节点,如果判断是父节点不允许删除。
Service实现层代码
/** * 删除内容节点信息 * @param id * @return */
@Override public E3Result deleteContentCategory(Long id) { //删除节点时需要判断是否有子节点 //痛id查询内容节点并且获取到父节点 TbContentCategory tbContentCategory = contentCategoryMapper.selectByPrimaryKey(id); Long parentId = tbContentCategory.getParentId(); if (tbContentCategory.getIsParent()){ return E3Result.build(1,"失败"); }else { contentCategoryMapper.deleteByPrimaryKey(id); TbContentCategoryExample example=new TbContentCategoryExample(); TbContentCategoryExample.Criteria criteria = example.createCriteria(); criteria.andParentIdEqualTo(parentId); List<TbContentCategory> childs = contentCategoryMapper.selectByExample(example); if (childs.size()==0) { //判断父节点的isParent属性是否为true如果不是就修改为true TbContentCategory parent = contentCategoryMapper.selectByPrimaryKey(parentId); if (parent.getIsParent()) { parent.setIsParent(false); //更新到数据库 contentCategoryMapper.updateByPrimaryKey(parent); } } //返回结果,返回E3Result,包含pojo return E3Result.ok(tbContentCategory); } }
表现层Controller
/** * 删除内容节点 * @param id * @return */ @RequestMapping(value = "/content/category/delete", method=RequestMethod.POST) @ResponseBody public E3Result deleteContentCategory(Long id){ //调用服务删除节点 E3Result result=contentCategoryService.deleteContentCategory(id); return result; }