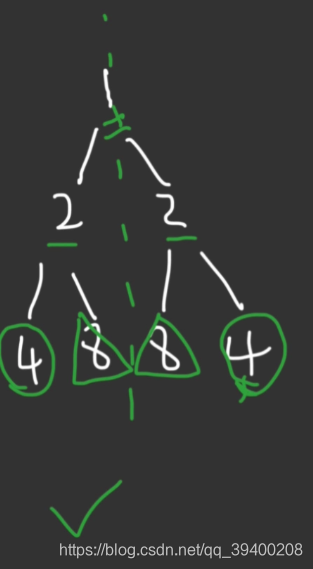
上图是对称的二叉树示例
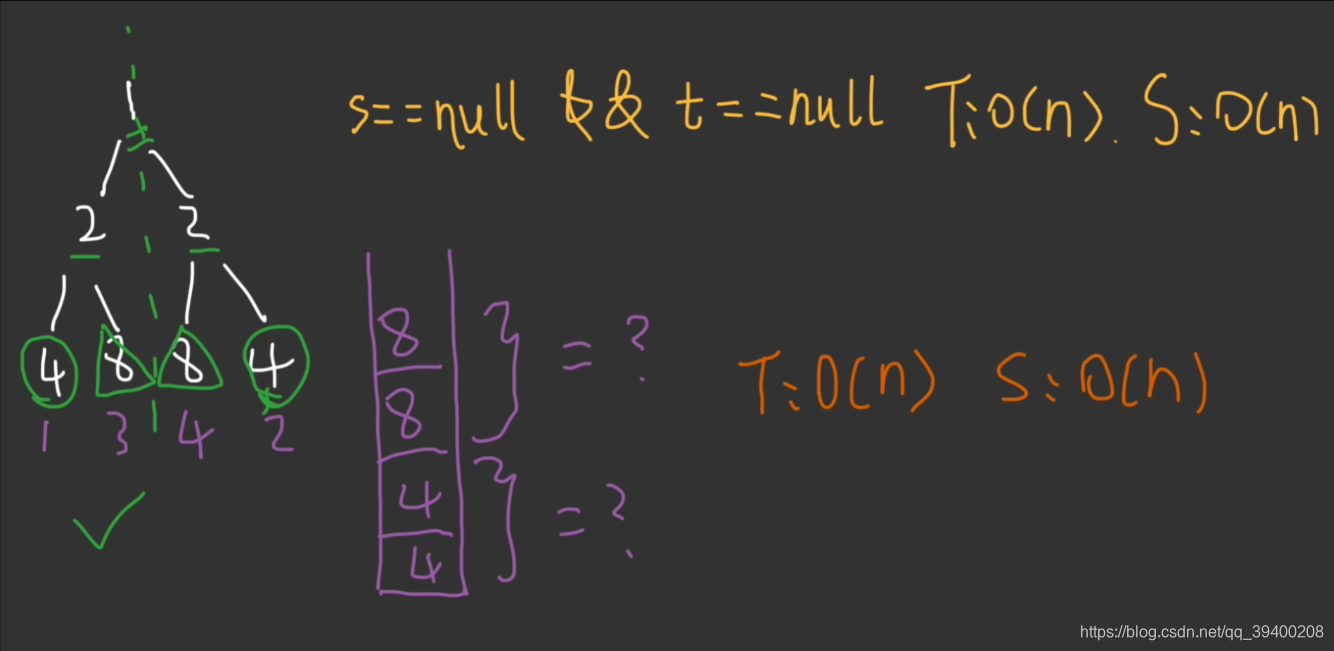
左节点的左指数,右节点的右指数
左节点的右指数,右节点的左指数
下面的代码用了两种做法
import java.util.Stack;
public class Fourth {
public class TreeNode{
int val;
TreeNode left;
TreeNode right;
TreeNode(int x) { val = x; }
}
boolean isSymmetric(TreeNode s, TreeNode t) {
if(s != null && t != null)
return s.val == t.val &&
isSymmetric(s.left, t.right) && isSymmetric(s.right, t.right);
else return s == null && t == null;
}
public boolean isSymmetricTreeRecursive(TreeNode root) {
if (root == null) return true;
return isSymmetric(root.left, root.right);
}
public boolean isSymmetricTreeIterative(TreeNode root) {
if(root == null) return true;
Stack<TreeNode> stack = new Stack<>();
stack.push(root.left);
stack.push(root.right);
while(!stack.empty()) {
TreeNode s = stack.pop(), t = stack.pop();
if(s == null && t == null) continue;
if(s == null || t == null) return false;
if(s.val != t.val) return false;
stack.push(s.left);
stack.push(t.right);
stack.push(s.right);
stack.push(t.left);
}
return false;
}
}