Bean标签的常用属性
1.Id:属性值名称随便起,但是不能有特殊符号
2.Class:创建类对象的所在类的全路径
3.Name:与Id相似,但是名称可以用特殊符号(遗留的一个问题,不经常用)
4.scope:设置Bean的作用范围
Bean的作用范围
1.Singleton:单例(默认方式),只能创建一个对象
2.Prototype:多例的
3.request:在web项目中,将spring创建的对象存放在request域中
4.session:在web项目中,将spring创建的对象存放在sessiont域中
5.globalSession:将spring创建的对象存放在globalSession域中
属性注入
- 创建对象的时候,向类里面的成员变量设置值
- 注入方式
· set方法注入(spring中最常用)
配置文件:
实体类:
<bean id="demo" class="edu.slxy.property.PropertyDemo1">
<property name="username" value="地方"></property>
<!-- <constructor-arg name="username" value="小孩"></constructor-arg> --><!-- name代表成员标量名,value:值 -->
</bean>
实体类:
package edu.slxy.property;
public class PropertyDemo1 {
private String username;
/*
* 采用set方法注入这个需要注释掉,否者会报错
* public PropertyDemo1(String username) {
this.username=username;
}*/
public void setUsername(String username) {
this.username = username;
}
public void test (){
System.out.println("username : "+username);
}
}
注入对象
配置文件:
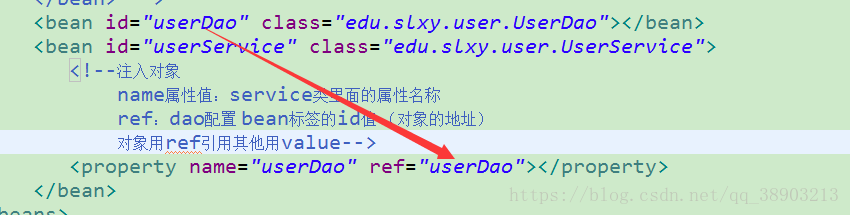
注入复杂类型属性值
配置文件
实体类:
· 有参数构造注入
· p名称空间注入
配置文件:
<bean id="demo" class="edu.slxy.property.PropertyDemo1">
<constructor-arg name="username" value="小孩"></constructor-arg><!-- name代表成员标量名,value:值 -->
</bean>
实体类:
public class PropertyDemo1 {
private String username;
public PropertyDemo1(String username) {
this.username=username;
}
public void test (){
System.out.println("username : "+username);
}
}
结果:
· 使用接口注入
Bean的实例化方式
1.使用类的无参构造函数创建(重点)
2.使用静态的工厂创建
(1)创建静态的方法,返回类的对象
xml文件
工厂类
package edu.slxy.bean;
public class Bean2Factory{
public static Bean2 getBean2(){
return new Bean2();
}
}
实体类
package edu.slxy.bean;
public class Bean2 {
public void add(){
System.out.println("add----------");
}
}
测试类
package text;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import edu.slxy.bean.Bean2;
import edu.slxy.user.User;
public class TextDemo {
public static void main(String[] args) {
ApplicationContext context=new ClassPathXmlApplicationContext("bean.xml");
Bean2 bean2=(Bean2) context.getBean("bean2");
System.out.println(bean2);
bean2.add();
//user.add();
}
}
3.使用实例工厂创建
1.配置文件
<bean id="bean3Factory" class="edu.slxy.bean.Bean3Factory"> </bean>
<bean id="bean3" factory-bean="bean3Factory" factory-method="getBean3"> </bean>
2.实体类
package edu.slxy.bean;
public class Bean3 {
public void add(){
System.out.println("add----------");
}
}
3.工厂类
package edu.slxy.bean;
public class Bean3Factory{
public Bean3 getBean3(){
return new Bean3();
}
}
4.测试类
package text;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import edu.slxy.bean.Bean2;
import edu.slxy.bean.Bean3;
import edu.slxy.user.User;
public class TextDemo {
public static void main(String[] args) {
ApplicationContext context=new ClassPathXmlApplicationContext("bean.xml");
Bean3 bean3=(Bean3) context.getBean("bean3");
System.out.println(bean3);
bean3.add();
//user.add();
}
}