递归分割生成迷宫
运行效果
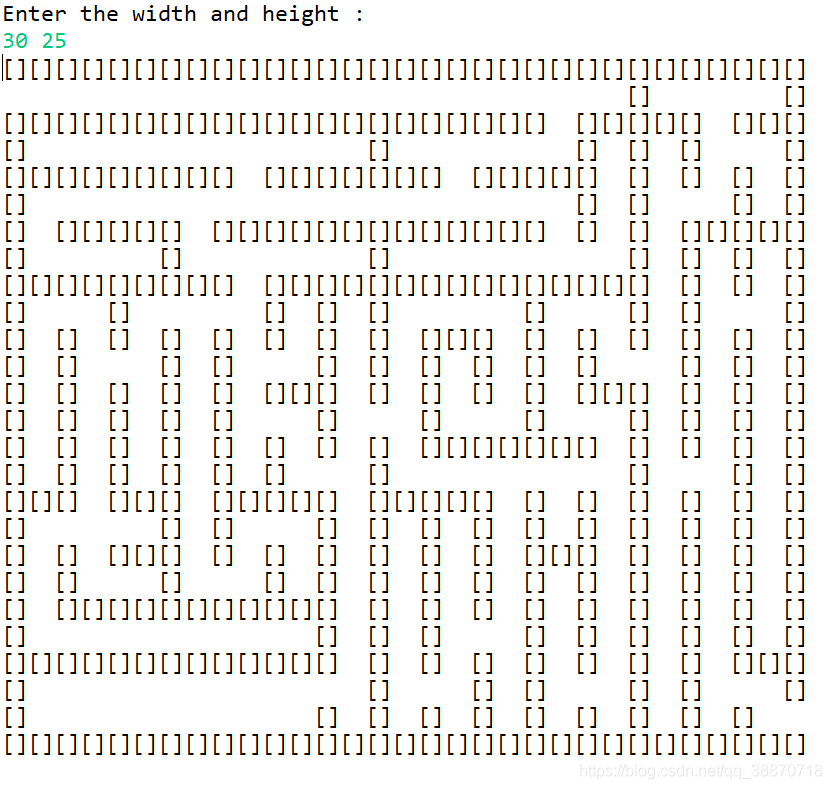
实现代码
package cn.edu.Maze;
import java.util.Random;
import java.util.Scanner;
public class Text {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.println("Enter the width and height :");
int width = in.nextInt(), height = in.nextInt();
RecursiveDivision maze = new RecursiveDivision(width, height);
maze.printMaze(maze.createMazeData());
in.close();
}
}
class RecursiveDivision {
private int[][] mazeData;
private final int WALL = 0;
private final int ROUND = 1;
private int width;
private int height;
public RecursiveDivision(int width, int height) {
this.width = width % 2 + 1 == 0 ? width : width + 1;
this.height = height % 2 + 1 == 0 ? height : height + 1;
}
public int[][] createMazeData() {
mazeData = new int[height][width];
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
if (x == 0 || x == width - 1 || y == 0 || y == height - 1)
mazeData[y][x] = WALL;
else
mazeData[y][x] = ROUND;
}
}
division(1, 1, width - 2, height - 2);
mazeData[1][0] = ROUND;
mazeData[height - 2][width - 1] = ROUND;
return mazeData;
}
private void division(int startX, int startY, int endX, int endY) {
Random random = new Random();
if (endX - startX < 2 || endY - startY < 2)
return;
int posX = startX + 1 + random.nextInt((endX - startX) / 2) * 2;
int posY = startY + 1 + random.nextInt((endY - startY) / 2) * 2;
for (int i = startX; i <= endX; i++)
mazeData[posY][i] = WALL;
for (int i = startY; i <= endY; i++)
mazeData[i][posX] = WALL;
division(startX, startY, posX - 1, posY - 1);
division(startX, posY + 1, posX - 1, endY);
division(posX + 1, posY + 1, endX, endY);
division(posX + 1, startY, endX, posY - 1);
switch (random.nextInt(4)) {
case 0:
openDoor(startX, posY, posX - 1, posY);
openDoor(posX, posY + 1, posX, endY);
openDoor(posX + 1, posY, endX, posY);
break;
case 1:
openDoor(posX, posY + 1, posX, endY);
openDoor(posX + 1, posY, endX, posY);
openDoor(posX, startY, posX, posY - 1);
break;
case 2:
openDoor(posX + 1, posY, endX, posY);
openDoor(posX, startY, posX, posY - 1);
openDoor(startX, posY, posX - 1, posY);
break;
case 3:
openDoor(posX, startY, posX, posY - 1);
openDoor(startX, posY, posX - 1, posY);
openDoor(posX, posY + 1, posX, endY);
break;
}
}
private void openDoor(int startX, int startY, int endX, int endY) {
Random random = new Random();
int x;
int y;
if (startY == endY) {
x = startX + random.nextInt((endX - startX) / 2 + 1) * 2;
mazeData[startY][x] = ROUND;
}
if (startX == endX) {
y = startY + random.nextInt((endY - startY) / 2 + 1) * 2;
mazeData[y][startX] = ROUND;
}
}
public void setWidth(int width) {
this.width = width % 2 + 1 == 0 ? width : width + 1;
}
public void setHeight(int height) {
this.height = height % 2 + 1 == 0 ? height : height + 1;
}
public static void printMaze(int[][] data) {
for (int i = 0; i < data.length; i++) {
for (int j = 0; j < data[0].length; j++) {
if (data[i][j] == 0)
System.out.print("[]");
else
System.out.print(" ");
}
System.out.println();
}
}
}