文章目录
Reactor模式及NIO
1.引子
Redis服务器: Redis服务器是一个事件驱动程序,主要处理文件事件(file event)和时间事件(time event)
Redis基于Reactor模式开发了网络事件处理器
2.Reactor模式
2.1Reactor模式定义
The reactor design pattern is an event handling pattern for handling service requests delivered concurrently to a service handler by one or more inputs. The service handler then demultiplexes the incoming requests and dispatches them synchronously to the associated request handlers
1.reactor模式是事件驱动模式
2.并发服务处理一个或多个输入
3.实现分离多个到达的请求,并同步分发给相关处理器
2.2网络编程模型
Reactor, Proactor, Asynchronous, Completion Token, and Acceptor-Connector
基础的socket编程实现见附录
2.3Reactor常用组件
- Dispatcher,分发器,根据Event类型来调用EventHandler
- Handle,句柄,操作系统管理的资源
- EventHandler,事件处理器
- Synchronous Event Demultiplexes,同步事件分离器
- Reactor模式时序图
- Reactor调用流程
initiate => receive => demultiplex => dispatch => process events
1.Dispatcher初始化
2.注册EventHandler到Dispatcher中,每个EventHanler包含对响应Handle的引用
3.启动EventLoop事件循环,调用select()方法,Synchronous Event Demultiplexes 阻塞等待 Event 发生
4.当某个或某些Handle的Event发生后,select()方法返回,Dispatcher根据返回的Event找到注册的EventHandler
并调用EventHandler的hand_event()方法
5.EventHandler的hand_event()方法还可以向Diapatcher注册新的EventHandler,用于处理下一个Event
- Reactor事件管理的接口
class Reactor {
public:
int register_handler(EventHandler *pHandler, int event);
int remove_handler(EventHandler *pHandler, int event);
void handle_events(timeval *ptv);
}
- EventHandler事件处理程序
class Event_Handler {
public:
// events maybe read/write/timeout/close .etc
virtual void handle_events(int events) = 0;
virtual HANDLE get_handle() = 0;
}
3.Reactor模式应用
3.1多线程IO模型
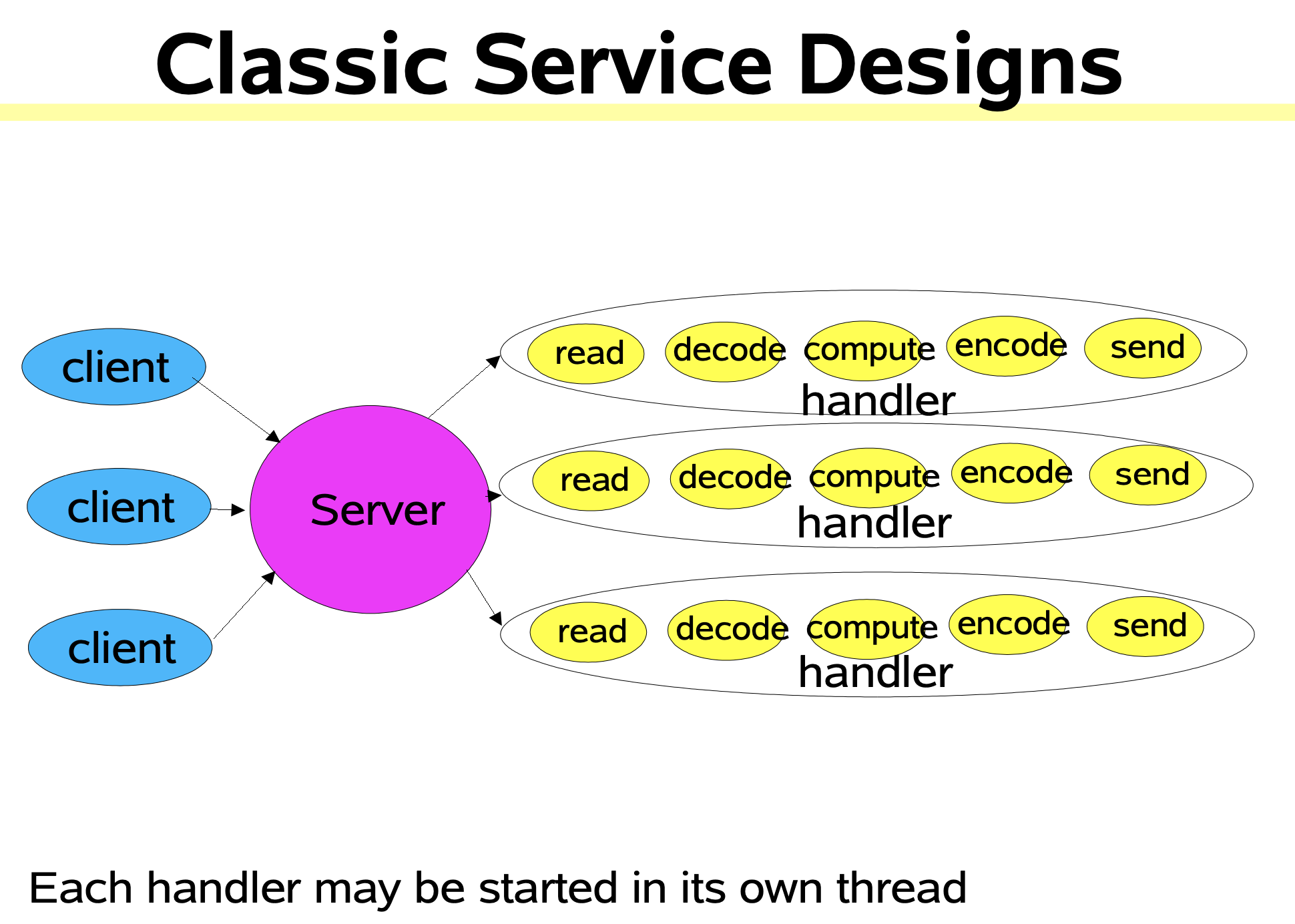
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
/**
* Classic ServerSocket Loop
*
* 传统的多线程IO,每个线程处理一个请求
*
* @Author deng shuo
* @Date 5/26/21 23:09
* @Version 1.0
*/
public class ClassicServerModel implements Runnable{
private static final int PORT = 8085;
private static final int MAX_INPUT = 1024 *1024;
@Override
public void run() {
try{
ServerSocket ss = new ServerSocket(PORT);
while(!Thread.interrupted()){
/*或者使用线程池*/
new Thread(new Handler(ss.accept())).start();;
}
}catch (IOException ex){
/* Handler exception*/
ex.printStackTrace();
}
}
static class Handler implements Runnable{
final Socket socket;
Handler(Socket s){
socket = s;}
@Override
public void run(