notify
- 参考:https://blog.youkuaiyun.com/weixin_44121696/article/details/109306019
public class ObjectTest {
public static void main(String[] args) {
Object objectLock = new Object();
new Thread(() -> {
synchronized (objectLock) {
System.out.println(Thread.currentThread().getName() + "--- hello t1");
try {
objectLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + "--- t1 被唤醒");
}
}, "t1").start();
new Thread(() -> {
synchronized (objectLock) {
System.out.println(Thread.currentThread().getName() + "--- hello t2");
try {
objectLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + "--- t2 被唤醒");
}
}, "t2").start();
new Thread(() -> {
synchronized (objectLock) {
System.out.println(Thread.currentThread().getName() + "--- hello t3");
try {
objectLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + "--- t3 被唤醒");
}
}, "t3").start();
new Thread(() -> {
synchronized (objectLock) {
System.out.println(Thread.currentThread().getName() + "--- hello t4");
try {
objectLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + "--- t4 被唤醒");
}
}, "t4").start();
new Thread(() -> {
synchronized (objectLock) {
objectLock.notify();
System.out.println(Thread.currentThread().getName() + "--- 唤醒别人");
}
}, "t5").start();
}
}
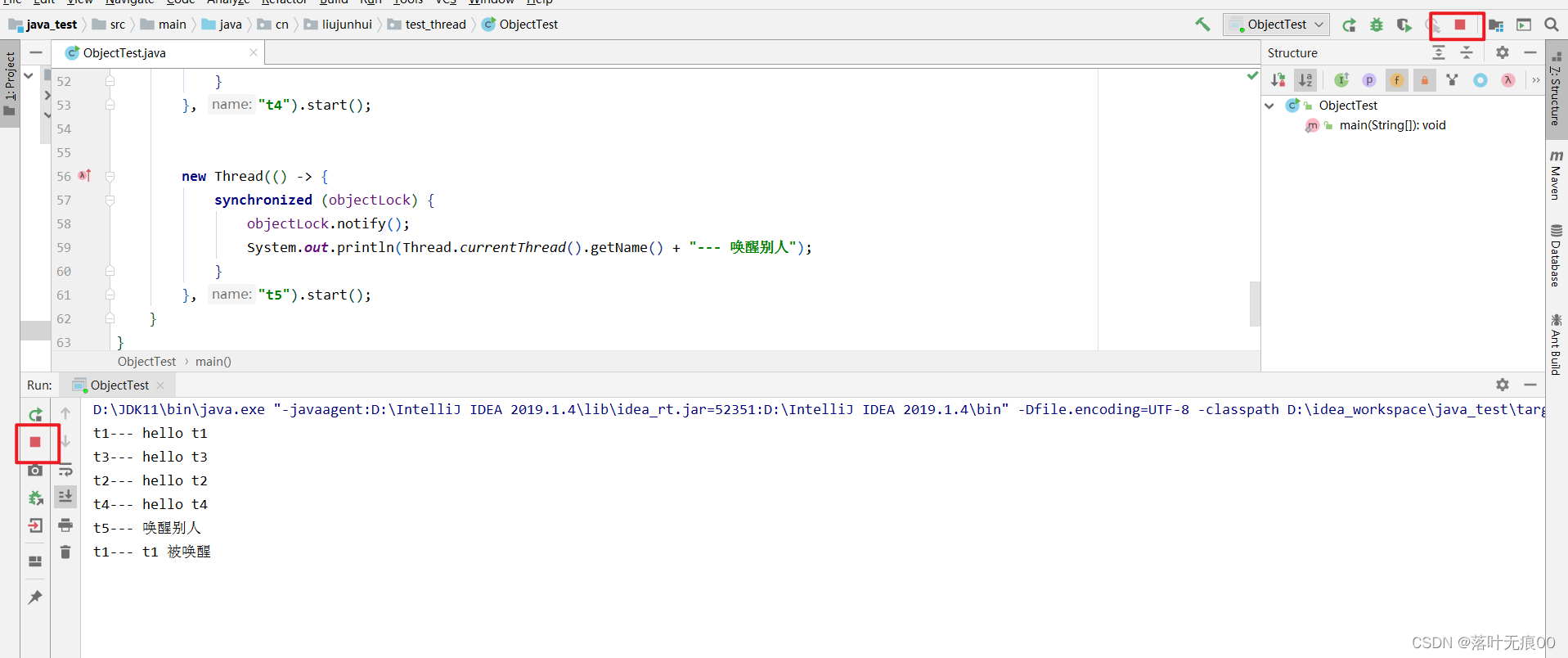
notifyAll()
public class ObjectTest {
public static void main(String[] args) {
Object objectLock = new Object();
new Thread(() -> {
synchronized (objectLock) {
System.out.println(Thread.currentThread().getName() + "--- hello t1");
try {
objectLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + "--- t1 被唤醒");
}
}, "t1").start();
new Thread(() -> {
synchronized (objectLock) {
System.out.println(Thread.currentThread().getName() + "--- hello t2");
try {
objectLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + "--- t2 被唤醒");
}
}, "t2").start();
new Thread(() -> {
synchronized (objectLock) {
System.out.println(Thread.currentThread().getName() + "--- hello t3");
try {
objectLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + "--- t3 被唤醒");
}
}, "t3").start();
new Thread(() -> {
synchronized (objectLock) {
System.out.println(Thread.currentThread().getName() + "--- hello t4");
try {
objectLock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + "--- t4 被唤醒");
}
}, "t4").start();
new Thread(() -> {
synchronized (objectLock) {
objectLock.notifyAll();
System.out.println(Thread.currentThread().getName() + "--- 唤醒别人");
}
}, "t5").start();
}
}
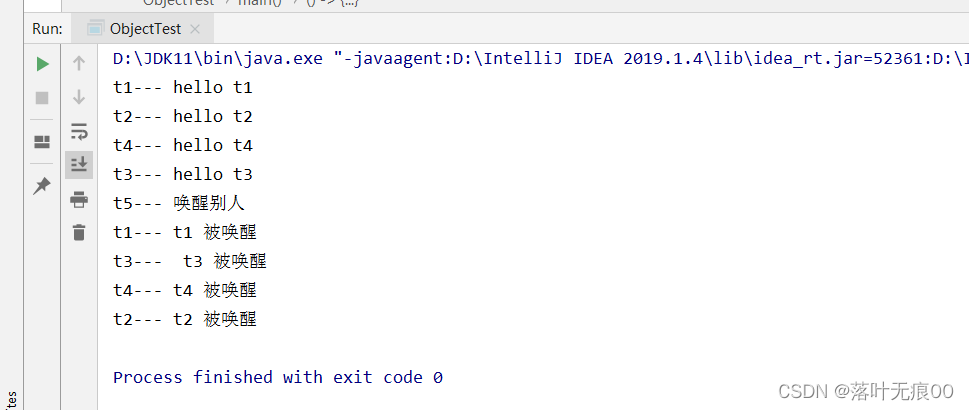