例子
threads.start(() => {
let jgy = new JianGuoYunUtil("账户", "应用密码", "我的坚果云");
let file_path = "/sdcard/A_My_DB/sqlite.db";
let byteData = files.readBytes(file_path);
let r = jgy.putFile(byteData, "sqlite.db");
console.log(r.isSuccess);
let r2 = jgy.getFile("sqlite.db")
if (r2.isSuccess) {
console.log(r2.msg.length);
files.writeBytes(file_path, r2.msg)
}
jgy.setThisFileName("demo", "txt")
jgy.putText("哈哈哈")
console.log(jgy.getText());
console.log(jgy.getFolderCatalog());
});
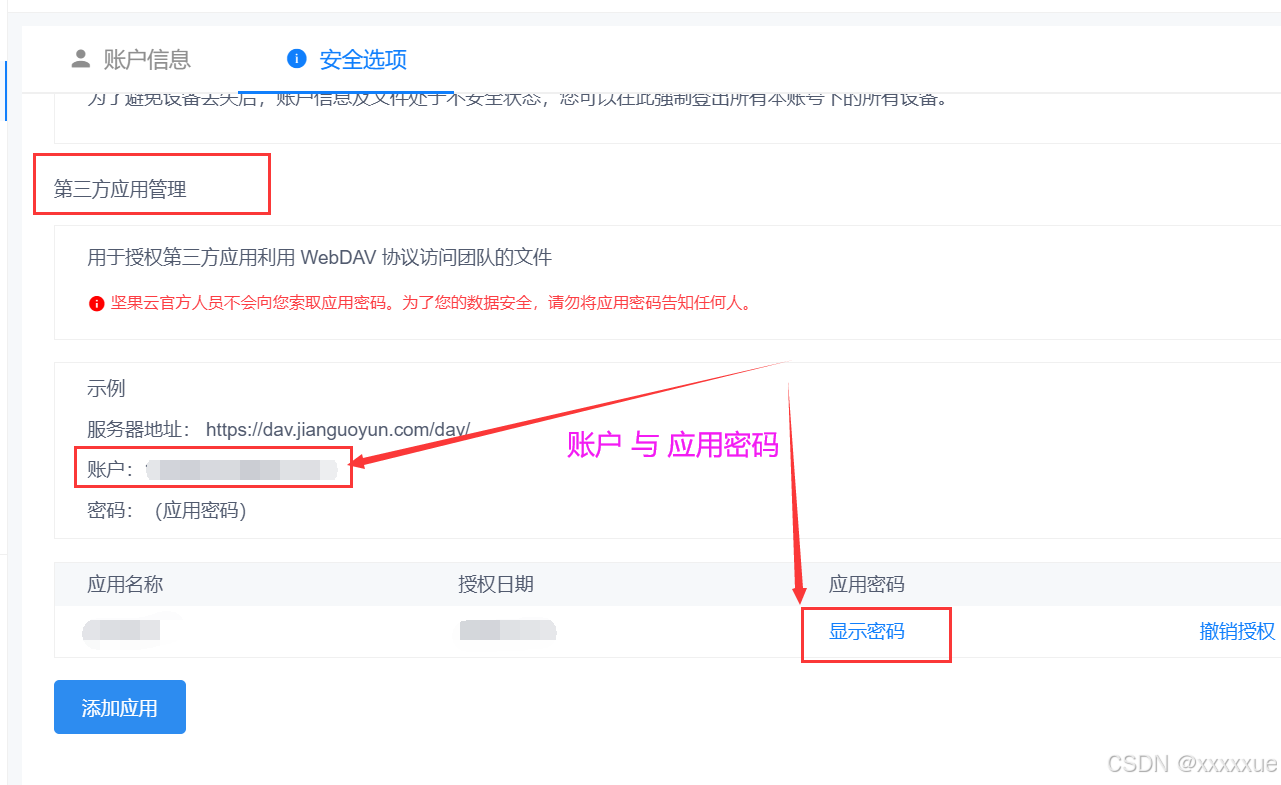
代码
var JianGuoYunUtil = (function () {
function JianGuoYunUtil(username, password, folderName) {
this.fileName = "";
this.folderName = folderName;
this.jgyHost = "https://dav.jianguoyun.com/dav/" + this.folderName + "/";
this.authorizationStr = "Basic " + this.__getBase64(username + ":" + password);
this.header = {
"Authorization": this.authorizationStr,
};
this.headerWithText = {
"Authorization": this.authorizationStr,
"Content-Type": "text/plain;charset=UTF-8",
};
this.__createFolder();
}
JianGuoYunUtil.prototype.setThisFileName = function (fileName, fileExtension) {
if (fileExtension == void 0) {
fileExtension = "txt";
}
this.fileName = fileName + "." + fileExtension;
};
JianGuoYunUtil.prototype.getFolderCatalog = function () {
var httpResp = http.request(this.jgyHost, {
method: "PROPFIND",
headers: this.header,
});
var retArr = [];
var xmlData = httpResp.body.string();
if (xmlData) {
var dataArr = xmlData.match(/<d:displayname>(.*?)<\/d:displayname>/g);
for (var _i = 0, _a = dataArr !== null && dataArr !== void 0 ? dataArr : []; _i < _a.length; _i++) {
var item = _a[_i];
item = item.replace("<d:displayname>", "").replace("</d:displayname>", "");
if (item != this.folderName) {
retArr.push(item);
}
}
}
return retArr;
};
JianGuoYunUtil.prototype.__createFolder = function () {
var httpResp = http.request(this.jgyHost, {
method: "MKCOL",
headers: this.header,
});
return httpResp.statusCode == 201;
};
JianGuoYunUtil.prototype.__getThisFileName = function (fileName) {
if (fileName == undefined) {
if (this.fileName != "") {
fileName = this.fileName;
}
else {
throw "当前必须传 fileName, 调用 setThisFileName 后,才可以不传 fileName";
}
}
return fileName;
};
JianGuoYunUtil.prototype.delete = function (fileName) {
var ret = { isSuccess: false, msg: "删除失败" };
try {
fileName = this.__getThisFileName(fileName);
var fileArr = this.getFolderCatalog();
if (fileArr.indexOf(fileName) > -1) {
http.request(this.jgyHost + fileName, {
method: "DELETE",
headers: this.header,
});
fileArr = this.getFolderCatalog();
if (fileArr.indexOf(fileName) < 0) {
ret.isSuccess = true;
ret.msg = "删除成功";
}
else {
ret.msg = "删除失败,文件依然在目录中";
}
}
else {
ret.isSuccess = true;
ret.msg = "文件不存在,无需删除";
}
}
catch (error) {
ret.msg = error;
}
return ret;
};
JianGuoYunUtil.prototype.getText = function (fileName) {
return this.downLoadFile(false, fileName);
};
JianGuoYunUtil.prototype.getFile = function (fileName) {
return this.downLoadFile(true, fileName);
};
JianGuoYunUtil.prototype.putText = function (data, fileName) {
var ret = { isSuccess: false, msg: "上传失败" };
try {
fileName = this.__getThisFileName(fileName);
var httpResp = http.request(this.jgyHost + fileName, {
method: "PUT",
headers: this.headerWithText,
body: data,
});
if (httpResp.statusCode == 201 || httpResp.statusCode == 204) {
ret.isSuccess = true;
ret.msg = "本地数据 上传到 坚果云 成功";
}
}
catch (error) {
ret.msg = error;
}
return ret;
};
JianGuoYunUtil.prototype.downLoadFile = function (isByteData, fileName) {
var ret = { isSuccess: false, msg: "获取失败" };
try {
fileName = this.__getThisFileName(fileName);
var httpResp = http.get(this.jgyHost + fileName, {
headers: this.header,
});
if (httpResp.statusCode == 404) {
var strRes = httpResp.body.string();
if (strRes.indexOf("doesn't exist") > -1 || strRes.indexOf("The file was deleted") > -1) {
ret.msg = "没有文件:" + fileName;
}
}
else if (httpResp.statusCode == 200) {
ret.isSuccess = true;
if (isByteData) {
ret.msg = httpResp.body.bytes();
}
else {
ret.msg = httpResp.body.string();
}
}
else {
ret.msg = "未知的状态码:" + httpResp.statusCode;
}
}
catch (error) {
ret.msg = "异常信息:" + JSON.stringify(error);
}
return ret;
};
JianGuoYunUtil.prototype.putFile = function (byteData, fileName) {
var ret = { isSuccess: false, msg: "上传失败" };
try {
fileName = this.__getThisFileName(fileName);
importPackage(Packages["okhttp3"]);
importClass(com.stardust.autojs.core.http.MutableOkHttp);
var client = new MutableOkHttp();
var mediaType = MediaType.parse("application/octet-stream");
var requestBody = RequestBody.create(mediaType, byteData);
var request = new Request.Builder()
.url(this.jgyHost + fileName)
.put(requestBody)
.header("Authorization", this.authorizationStr)
.header("Content-Type", "application/octet-stream")
.header("Content-Length", byteData.length)
.build();
var response = client.newCall(request).execute();
if (response.code() == "201" || response.code() == "204") {
ret.isSuccess = true;
ret.msg = "本地数据 上传到 坚果云 成功";
}
}
catch (error) {
ret.msg = error;
}
return ret;
};
JianGuoYunUtil.prototype.__getBase64 = function (str) {
return java.lang.String(android.util.Base64.encode(java.lang.String(str).getBytes(), 2));
};
return JianGuoYunUtil;
}());