#include <iostream>
#include <event2/event.h>
#include <event2/listener.h>
using namespace std;
#define SPORT 5001
void listen_cb(struct evconnlistener *e, evutil_socket_t s, struct sockaddr *a, int socklen, void *arg)
{
cout << "listen_cb" << endl;
}
int main()
{
#ifdef _WIN32
WSADATA wver;
WSAStartup(MAKEWORD(2, 2), &wver);
#else
if (signal(SIGPIPE, SIG_IGN) == SIG_ERR)
return 1;
#endif
std::cout << "test libevent!\n";
event_base* base = event_base_new();
if (base)
{
std::cout << "event_base_new success!" << std::endl;
}
sockaddr_in sin;
memset(&sin, 0, sizeof(sin));
sin.sin_family = AF_INET;
sin.sin_port = htons(SPORT);
evconnlistener *ev = evconnlistener_new_bind(
base,
listen_cb,
base,
LEV_OPT_REUSEABLE|LEV_OPT_CLOSE_ON_FREE,
10,
(sockaddr*) &sin,
sizeof(sin)
);
if(base)
event_base_dispatch(base);
if(ev)
evconnlistener_free(ev);
if(base)
event_base_free(base);
#ifdef _WIN32
WSACleanup();
#endif
return 0;
}
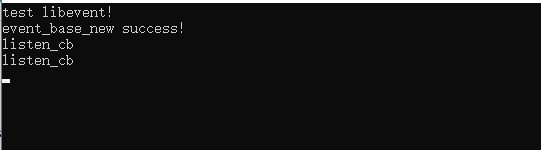