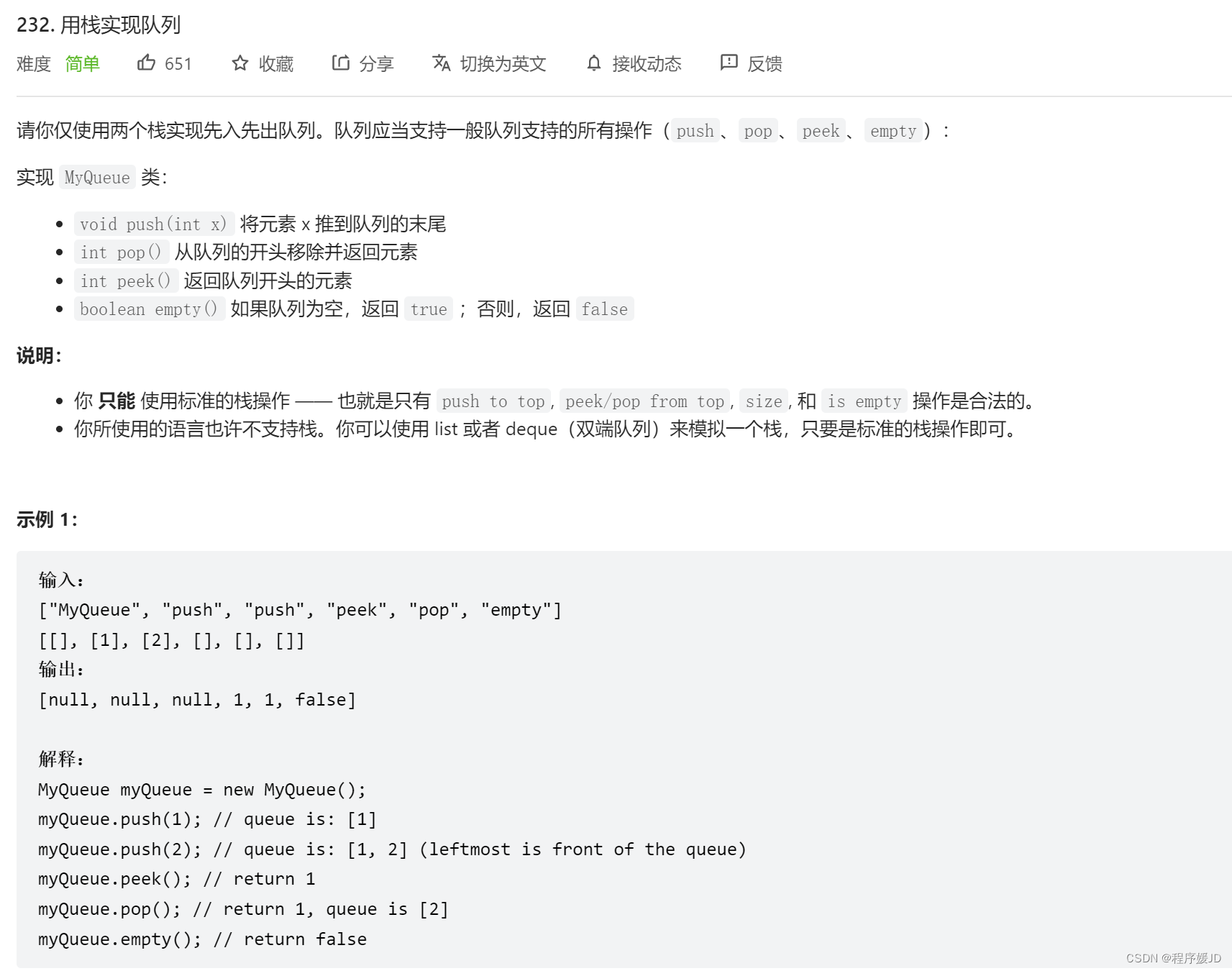
c++:
class MyQueue {
public:
stack<int>stkin;
stack<int>stkout;
MyQueue() {
}
void push(int x) {
stkin.push(x);
}
int pop() {
if(stkout.empty()){
while(!stkin.empty()){
stkout.push(stkin.top());
stkin.pop();
}
}
int res = stkout.top();
stkout.pop();
return res;
}
int peek() {
int res = this->pop();
stkout.push(res);
return res;
}
bool empty() {
return stkout.empty() && stkin.empty();
}
};
go:
type MyQueue struct {
stack []int
back []int
}
func Constructor() MyQueue {
return MyQueue{
stack:make([]int,0),
back:make([]int,0),
}
}
func (this *MyQueue) Push(x int) {
this.stack = append(this.stack,x)
}
func (this *MyQueue) Pop() int {
if(len(this.back) == 0){
for len(this.stack)!=0{
val := this.stack[len(this.stack)-1]
this.stack = this.stack[:len(this.stack)-1]
this.back = append(this.back,val)
}
}
val := this.back[len(this.back)-1]
this.back = this.back[:len(this.back)-1]
return val
}
func (this *MyQueue) Peek() int {
val := this.Pop()
if val == 0{
return 0
}
this.back = append(this.back,val)
return val
}
func (this *MyQueue) Empty() bool {
return len(this.back) == 0 && len(this.stack)==0
}