概念:通过建造者接口可以将一个复杂对象的构建过程与对象的表现相分离,使同样的构建过程可以创建出不同的表现。
例子: 摩拜工厂(框架、轮胎、gps)、 ofo工厂(框架、轮胎、gps),用户通过调用建造者封装一个方法调用(框架、轮胎、gps)方法生产自行车,用户在调用获取方法获取生产好的自行车。
我们可以试着将车的组装和零部件生产分离开来:让一个类似“导演”的角色负责车子组装,而具体造什么样的车需要什么样的零部件让具体的“构造者”去实现,“导演”知道什么样的车怎么造,需要的零部件则让“构造者”去建造,何时完成由“导演”来控制并最终返回给客户端。
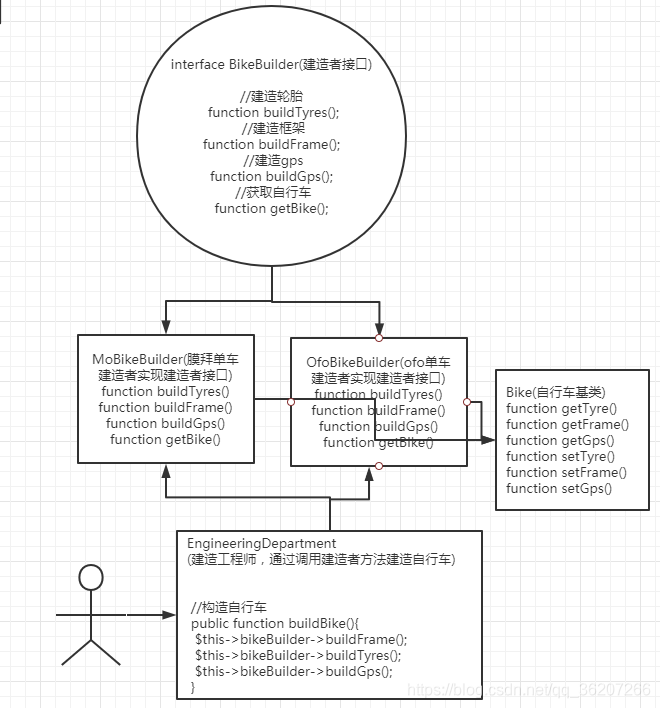
<?php
/**
* 自行车基类
*/
class Bike
{
protected $tyre;
protected $frame;
protected $gps;
public function getTyre(){
return $tyre;
}
public function getFrame(){
return $frame;
}
public function getGps(){
return $gps;
}
public function setTyre(string $tyre){
return $this->tyre = $tyre;
}
public function setFrame(string $frame){
return $this->frame = $frame;
}
public function setGps(string $gps){
return $this->gps = $gps;
}
}
//建造者接口
interface BikeBuilder
{
//建造轮胎
public function buildTyres();
//建造框架
public function buildFrame();
//建造gps
public function buildGps();
//获取自行车
public function getBike();
}
/**
* 膜拜单车建造者 实现 建造者接口
*/
class MoBikeBuilder implements BikeBuilder
{
public $bike;
public function __construct(){
$this->bike = new Bike();
}
public function buildTyres(){
return $this->bike->setTyre('橙色轮胎');
}
public function buildFrame(){
return $this->bike->setFrame('橙色框架');
}
public function buildGps(){
return $this->bike->setGps('mobike gps');
}
public function getBike(){
return $this->bike;
}
}
/**
* ofo单车建造者 实现 建造者接口
*/
class OfoBikeBuilder implements BikeBuilder
{
public $bike;
public function __construct(){
$this->bike = new Bike();
}
public function buildTyres(){
return $this->bike->setTyre('黑色轮胎');
}
public function buildFrame(){
return $this->bike->setFrame('黑色框架');
}
public function buildGps(){
return $this->bike->setGps('ofo gps');
}
public function getBike(){
return $this->bike;
}
}
//建造工程师,通过调用不同组件建造自行车
class EngineeringDepartment
{
protected $bikeBuilder;
public function __construct(BikeBuilder $bikeBuilder){
$this->bikeBuilder = $bikeBuilder;
}
//构造自行车
public function buildBike(){
$this->bikeBuilder->buildFrame();
$this->bikeBuilder->buildTyres();
$this->bikeBuilder->buildGps();
}
}
//==========================================================================================================
//建造者模式
//
class BuilderTest
{
public static function main(){
//建造膜拜自行车
$moBikeBuilder = new MoBikeBuilder();
$enginner = new EngineeringDepartment($moBikeBuilder);
$enginner->buildBike();
$moBike = $moBikeBuilder->getBike();
var_dump($moBike) . "</br>";
//建造ofo自行车
$ofoBikeBuilder = new OfoBikeBuilder();
$enginner = new EngineeringDepartment($ofoBikeBuilder);
$enginner->buildBike();
$ofoBike = $ofoBikeBuilder->getBike();
var_dump($ofoBike) . "</br>";
}
}
require 'builder.php';
BuilderTest::main();