队列
package queue_stack;
/**
* 使用链表来实现队列
* @author User
*
*/
public class MyQueue {
Node head;
/**
* 入队
* @param data
*/
public void add(int data) {
Node node = new Node(data);
if(isEmpty()) {
head = node;
}else {
Node temp = head;
while(temp.next != null) {
temp = temp.next;
}
// 此时temp为队列的最后一个元素
temp.next = node;
}
}
/**
* 出队
* @return
*/
public int remove() {
if(isEmpty()) {
throw new RuntimeException("队列是空的!");
}
int data = head.data;
head = head.next;
return data;
}
/**
* 打印
*/
public void print() {
Node temp = head;
while(temp != null) {
System.out.print(temp.data + " ");
temp = temp.next;
}
}
/**
* 获取队列长度
* @return
*/
public int length() {
if(head == null) {
return 0;
}
Node temp = head;
// 计数器
int count = 0;
// 从头遍历到队尾
while(temp != null) {
temp = temp.next;
count++;
}
return count;
}
/**
* 判空
* @return
*/
public boolean isEmpty() {
return head == null;
}
}
class Node{
int data;
Node next;
public Node(int data) {
this.data = data;
}
}
栈
package queue_stack;
/**
* 用两个队列模拟入栈和出栈操作
*
* @author Administrator
*
*/
public class MyStack {
MyQueue queueA = new MyQueue();// 负责入栈
MyQueue queueB = new MyQueue();// 作为一个中转站,负责出栈
/**
* 入栈
* @param data
*/
public void push(int data) {
queueA.add(data);
}
/**
* 出栈
* 出栈时,先将queueA中除了最后一个元素外依次出队,并压入队列queueB中,然后将留在queueA中的最后一个元素出队列即为出栈元素,最后还要把queueB中的元素再次压回到queueA中
* @return
*/
public int pop() {
if (queueA.isEmpty()) {
throw new RuntimeException("栈是空的!");
}
// 先将queueA中除了最后一个元素都出队,然后入队到queueB
Node temp = queueA.head;
while (temp.next != null) {
queueB.add(queueA.remove());
temp = temp.next;
}
// 到这一步出了while循环,说明temp来到了队尾,队尾元素正是要出栈的元素
int data = temp.data;
// 队尾元素出队(相当于出栈)
queueA.remove();
// 把queueB中的所有元素重新拷贝回queueA中
while (!queueB.isEmpty()) {
queueA.add(queueB.remove());
}
return data;
}
public boolean isEmpty() {
return queueA.isEmpty();
}
}
测试类
package queue_stack;
public class Test {
public static void main(String[] args) {
MyStack stack = new MyStack();
stack.push(1);
stack.push(2);
stack.push(3);
stack.pop();
stack.push(4);
stack.push(5);
stack.pop();
System.out.println(stack.pop());
System.out.println(stack.pop());
System.out.println(stack.pop());
}
}
运行结果
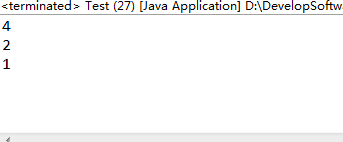