主要内容
- Collection集合
- 迭代器
- 增强for
- 泛型
废话:好吧,倒腾了好久,终于开始csdn的第一篇博客了。此类文章纯属笔记整理。。。
问题:集合和数组都是容器,区别?
1.数组的长度是固定的。集合的长度是可变的。
2.数组中存储的是同一类型的元素,可以存储基本数据类型值。 集合存储的都是对象。而且对象的类型可以不一致。
集合框架的继承体系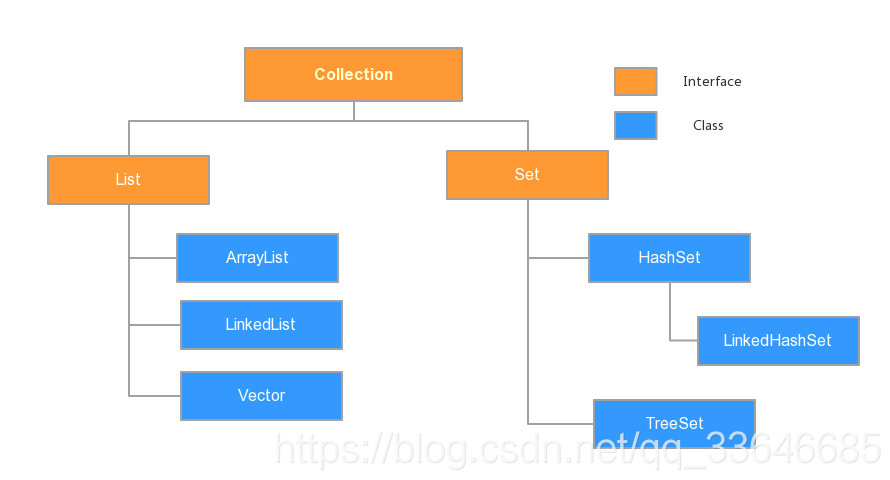
Collection与set的区别?
List接口: - 存取有序 有索引 数据可以重复
set接口: - 存取无序 没有索引 数据不可以重复
Collection
1.常用功能:
- public boolean add(E e): 把给定的对象添加到当前集合中 。
- public void clear() :清空集合中所有的元素。
- public boolean remove(E e): 把给定的对象在当前集合中删除。
- public boolean contains(E e): 判断当前集合中是否包含给定的对象。
- public boolean isEmpty(): 判断当前集合是否为空。
- public int size(): 返回集合中元素的个数。
- public Object[] toArray(): 把集合中的元素,存储到数组中。可以间接对集合进行遍历。
迭代器
public class Test01 {
public static void main(String[] args) {
// 使用多态方式 创建对象
Collection<String> coll = new ArrayList<String>();
// 添加元素到集合
coll.add("aaaa");
coll.add("bbbb");
coll.add("cccc");
//根据当前集合对象生成迭代器对象
Iterator<String> it = coll.iterator();
//获取数据
for (String s : coll) {
System.out.println("s = " + s);
}
}
}
嗯~最后加上一道面试题,关于装箱和自动装箱的:
public class Demo02 {
public static void main(String[] args) {
//创建包装类对象
Integer i1 = new Integer(50);//装箱
Integer i2 = new Integer(50);//装箱
System.out.println(i1==i2);//false
Integer i3 = new Integer(500);//装箱
Integer i4 = new Integer(500);
System.out.println(i3==i4);//false
//享元设计模式
//自动装箱Integer.valueof() 共享同一空间
//追加源代码退回上一层ctrl+alt+左键
Integer i5 = 50;
Integer i6 = 50;
System.out.println(i5==i6);//true
Integer i7 = 50;
Integer i8 = 50;
System.out.println(i7==i8);//false
}
}