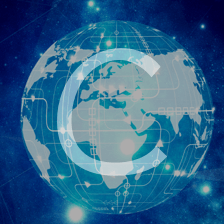
LeetCode
零分分分分
00
展开
-
LeetCodeNo.162寻找峰值(二分查找)
注意题目中假定的是nums[-1]==nums[n]=负无穷,解法见注释class Solution { public int findPeakElement(int[] nums) { if(nums.length==0) return 0; int left=0,right=nums.length-1; int...原创 2020-03-05 22:00:01 · 168 阅读 · 0 评论 -
LeetCode No.81搜索旋转排序数组II
class Solution { public boolean search(int[] nums, int target) { if(nums==null||nums.length==0) return false; return search(nums,0,nums.length-1,target); } priv...原创 2020-03-05 19:11:21 · 136 阅读 · 0 评论 -
LeetCode No.74搜索二维矩阵
class Solution { public boolean searchMatrix(int[][] matrix, int target) { if(matrix==null||matrix.length==0||matrix[0].length==0) return false;//注意这里要判断列的数目是否为0 int le...原创 2020-03-05 11:53:00 · 248 阅读 · 1 评论 -
LeetCodeNo.29两数相除
class Solution { public int divide(int dividend, int divisor) { if (dividend == Integer.MIN_VALUE && Math.abs(divisor) == 1) { return divisor>0?Integer.MIN_VALUE:Int...原创 2020-03-05 10:54:44 · 135 阅读 · 0 评论 -
LeetCode No.300 最长上升子序列(动态规划)
class Solution { public int lengthOfLIS(int[] nums) { if(nums==null||nums.length==0) return 0; int[] beforeCounts=new int[nums.length]; for(int i=0;i<nums.length;i++){ ...原创 2020-03-05 00:50:52 · 153 阅读 · 0 评论 -
LeetCode No.287 寻找重复数
class Solution { public int findDuplicate(int[] nums) { int left=1,right=nums.length-1; int mid=0; while(left<right){ int count=0;//这个count一定要放这里面,重置,不能放到whi...原创 2020-03-05 00:12:07 · 121 阅读 · 0 评论 -
LeetCodeNo.139单词拆分
class Solution { public boolean wordBreak(String s, List<String> wordDict) { boolean[] dp=new boolean[s.length()+1];//表示 s 中以 i - 1 结尾的字符串是否可被 wordDict 拆分 dp[0]=true; ...原创 2020-02-04 19:53:14 · 128 阅读 · 0 评论 -
leetcode No.120三角形最小路径和
//自底向上的解法class Solution { public int minimumTotal(List<List<Integer>> triangle) { //加一行是为了方便dp数组最后一行的赋值(dp最后一行就是三角形列表最后一行) int[][] dp=new int[triangle.size()+1][triangle...原创 2020-02-03 20:38:16 · 153 阅读 · 0 评论 -
LeetCode No.64最小路径和
class Solution { public int minPathSum(int[][] grid) { int m=grid.length; int n=grid[0].length; int[][] dp=new int[m][n]; //dp[i][j]代表到当前位置的最小路径和 for(int i=0;i<m...原创 2020-02-01 19:03:49 · 137 阅读 · 0 评论 -
LeetCode No.63不同路径2
class Solution { public int uniquePathsWithObstacles(int[][] obstacleGrid) { if(obstacleGrid[0][0]==1) return 0; //入口为1 则结果为0 int m=obstacleGrid.length; int n=obstacleGrid[...原创 2020-02-01 18:43:31 · 108 阅读 · 0 评论 -
LeetCodeNo.4两个有序数组的中位数
思路就是合并两个有序数组,然后再在新数组上进行计算中位数time:98.38内存:27.87class Solution { public double findMedianSortedArrays(int[] nums1, int[] nums2) { int len=nums1.length+nums2.length; int[] nums3=n...原创 2020-01-30 12:25:45 · 164 阅读 · 0 评论 -
leetcode No.61旋转链表
/** * Definition for singly-linked list. * public class ListNode { * int val; * ListNode next; * ListNode(int x) { val = x; } * } */ 思路就是 先将链表首尾相接变成循环列表,然后按照k值找到切断的点切开,返回新的头部1ms ...原创 2019-12-23 13:49:20 · 86 阅读 · 0 评论 -
LeetCodeNo.60第k个
参考评论,我的思路和高赞评论基本一样,也是先确定第一个数,然后第二个数..也是采用列表,计算索引,计算阶乘,更新k值,更新列表。但是我的不足很多:1、打算采用递归来做,递归函数参数选用(不断更新的list,n-1,变化的k值)但发现不需要采用递归,应该类似for循环递推2、求解阶乘;打算写一个阶乘函数,一直调用函数,却发现可以直接一次计算所有阶乘,放入数组,需要的时候直接拿3、确定索引...原创 2019-12-23 12:01:11 · 135 阅读 · 0 评论 -
LeetCode No.59螺旋矩阵2
思路和螺旋矩阵一样,小小的改动class Solution { public int[][] generateMatrix(int n) { int[][] result=new int[n][n]; int i=0,j=0,di=0,dj=1; for(int k=1;k<n*n+1;k++){ resul...原创 2019-12-23 10:00:48 · 107 阅读 · 0 评论 -
LeetCode No.56 合并区间
参考评论区java解答,这里对二维数组的排序,排序规则是按照里面数组第一个值从小到大排序.然后每一次for循环会得到一个区间,也就是每次while循环结束会得到一个区间,关键在于while里面判断下一个数组是否和前一个有交集,若是有则扩增区间,没有的话就结束while循环,那么当前的区间一定是结果集中的(因为已经排序过) 另外注意这里有两次i++class Solution {...原创 2019-12-22 11:06:05 · 104 阅读 · 0 评论 -
LeetCodeNo.55 跳跃游戏
比较简单,有点类似于以前有道赌石头游戏的题?思路就是:从数组最后开始判断,如果索引n可以到达终点,那么将索引n作为终点,判断前面的可不可以到达索引n,如此一直将终点前移。需要注意,该方法不会丢失解:也就是不会出现到不了索引n,但是可以到终点的走法,可证明。class Solution { public boolean canJump(int[] nums) { in...原创 2019-12-21 15:41:29 · 119 阅读 · 0 评论 -
LeetCode No.54螺旋矩阵
将已经走过的地方置0,然后拐弯的时候判断一下是不是已经走过了,如果走过了就计算一下新的方向class Solution { public List<Integer> spiralOrder(int[][] matrix) { List<Integer> result = new ArrayList<>(); int i...原创 2019-12-21 11:46:12 · 144 阅读 · 0 评论 -
LeetCode No.49字母异位词分组
参考解法:在美版leetcode上看到大神的思路,用质数表示26个字母,把字符串的各个字母相乘,这样可保证字母异位词的乘积必定是相等的。其余步骤就是用map存储注意这里直接将List 作为值放入到map中,后续用add方法,更新map,此外注意更新map的写法以及遍历map值的写法beat 99.52% 98.98%class Solution { public List<...原创 2019-11-29 22:56:04 · 143 阅读 · 0 评论 -
leetcode No.48旋转图像
找规律找了挺久的,主要就是从外层四条边,再一层层到最内部,直到最里面只剩一个元素或者没有元素。class Solution { public void rotate(int[][] matrix) { int len=matrix.length; for(int x=0;len-2*x>1;x++){ //x记录层数,最外层为0 ...原创 2019-11-25 22:56:58 · 82 阅读 · 0 评论 -
LeetCode No.39组合总和 No.46全排列(回溯算法)
要特别注意在for循环里的递归,相当于每一次递归又包含了n次递归。因此递归return的位置,要注意别搞错。class Solution { public List<List<Integer>> combinationSum(int[] candidates, int target) { List<List<Integer>>...原创 2019-11-21 16:40:21 · 148 阅读 · 0 评论 -
leetcode No.36有效的数独
class Solution { public boolean isValidSudoku(char[][] board) { boolean[][] rows=new boolean[9][9]; boolean[][] columns=new boolean[9][9]; boolean[][] grids=new boolean[9][...原创 2019-11-21 11:17:31 · 110 阅读 · 0 评论 -
LeetCode No.34在排序数组中查找元素的第一个和最后一个位置
//参考评论区解答,还要再消化消化,主要是边界的选择class Solution { public int[] searchRange(int[] nums, int target) { int[] result={-1,-1}; if(nums.length==0) return result; int l=0,r=nums.length...原创 2019-11-20 22:04:39 · 106 阅读 · 0 评论 -
leetcode No.33搜索旋转排序数组
class Solution { public int search(int[] nums, int target) { //take arrays to two part,one part is sorted,another part is rotated sorted; return search(nums,0,nums.length-1,target)...原创 2019-11-18 11:14:39 · 123 阅读 · 0 评论 -
LeetCode No.31 下一个排列
题干的意思是:找出这个数组排序出的所有数中,刚好比当前数大的那个数比如当前 nums = [1,2,3]。这个数是123,找出1,2,3这3个数字排序可能的所有数,排序后,比123大的那个数 也就是132如果当前 nums = [3,2,1]。这就是1,2,3所有排序中最大的那个数,那么就返回1,2,3排序后所有数中最小的那个,也就是1,2,3 -> [1,2,3]解题思路就是:从最...原创 2019-11-15 17:54:03 · 114 阅读 · 0 评论 -
LeetCodeNo.19删除链表倒数第N个节点
使用两个指针,一个是fast一个是slow先让fast走n步,然后再让fastslow同时往前走当fast走到头时,slow即是倒数第n+1个节点了。这里注意如果要删除的是第一个节点的话,fast走的过程中就会到头,所以判断是否出现这种情况,若是直接删除头结点```java/** * Definition for singly-linked list. * public clas...原创 2019-11-15 15:44:23 · 104 阅读 · 0 评论 -
LeetCode No.532数组中的k-diff对
class Solution { public int findPairs(int[] nums, int k) //当k!=0时,当K=0时,脑子疼 想不出来 Arrays.sort(nums); int result=0; Set<Integer> set=new HashSet<Integer>...原创 2019-11-04 10:48:20 · 88 阅读 · 0 评论 -
LeetCode No.520检测大写字母
class Solution { public boolean detectCapitalUse(String word) { char[] a=word.toCharArray(); int upNums=0,lowNums=0; for(int i=0;i<a.length;i++){ if(a[i]<...原创 2019-11-01 17:51:42 · 188 阅读 · 0 评论 -
leetcode No.509斐波那契数
class Solution { public int fib(int N) { if(N==0||N==1) return N; else return fib(N-1)+fib(N-2); }}原创 2020-01-05 15:59:58 · 120 阅读 · 0 评论 -
LeetCodeNo.507完美数
class Solution { public boolean checkPerfectNumber(int num) { if(num==0) return false; int a=num,i=1; while(i<=a/2){ if(a%i==0) num-=i; ...原创 2019-11-01 16:22:50 · 180 阅读 · 0 评论 -
leetcodeNo.506 相对名次
class Solution { public String[] findRelativeRanks(int[] nums) { //思路是先用map记录每个数字出现的位置,然后将数组排序,排序完名次也就出现了,根据map找到该数字的位置,放入名次就可以了 String[] result=new String[nums.length]; Ma...原创 2019-11-01 15:32:21 · 102 阅读 · 0 评论 -
LeetCodeNo.504七进制
class Solution { public String convertToBase7(int num) { if(num==0) return "0"; StringBuilder sb=new StringBuilder(); int absNum=Math.abs(num); while(absNum>0){...原创 2020-01-05 16:00:31 · 96 阅读 · 0 评论 -
LeetCode No.496下一个更大元素I
class Solution { public int[] nextGreaterElement(int[] nums1, int[] nums2) { int[] result=new int[nums1.length]; Map<Integer,Integer> map=new HashMap<>(); for(i...原创 2019-11-01 10:06:37 · 100 阅读 · 0 评论 -
leetcode No.492构造矩形
class Solution { public int[] constructRectangle(int area) { int n=(int)Math.sqrt(area); while(area%n!=0){ n--; } return new int[]{area/n,n}; }}一...原创 2020-01-05 16:00:52 · 122 阅读 · 0 评论 -
leetcode No.485最大连续1的个数
class Solution { public int findMaxConsecutiveOnes(int[] nums) { int result=0,curLength=0; for(int i=0;i<nums.length;i++){ if(nums[i]==1){ curLength+...原创 2019-10-31 17:03:54 · 102 阅读 · 0 评论 -
LeetCode No.476数字的补数
class Solution { public int findComplement(int num) { int i=0,result=0; while(num>0){ if(num%2==0){ result+=Math.pow(2,i); } ...原创 2019-10-31 16:14:21 · 102 阅读 · 0 评论 -
LeetCode No.475供暖器
参考解答class Solution { public int findRadius(int[] houses, int[] heaters) { Arrays.sort(houses); Arrays.sort(heaters); int result=0; int j=0; for(int i=0;i&...原创 2020-01-05 16:01:04 · 152 阅读 · 0 评论 -
LeetCode No.463 岛屿的周长
class Solution { public int islandPerimeter(int[][] grid) { int result=0; for(int i=0;i<grid.length;i++){ for(int j=0;j<grid[i].length;j++){ if(gr...原创 2019-10-30 16:14:05 · 83 阅读 · 0 评论 -
No.461汉明距离
class Solution { public int hammingDistance(int x, int y) { int result=0; while(x!=0||y!=0){ result+=(x%2)^(y%2); //除2取余 x/=2; y/=2; }...原创 2019-10-30 15:27:52 · 116 阅读 · 0 评论 -
leetcode No.459重复的子字符串
class Solution { public boolean repeatedSubstringPattern(String s) { //1、若字符串S从头取一段放到尾部,仍能组成原字符串S,那么S是重复的。 //证明:设字符串由ax组成,a代表一段字符串,x中包含了位置的字符串以及未知长度 //若ax=xa,则x=a或aa或aaa......原创 2019-10-30 14:45:01 · 91 阅读 · 0 评论 -
LeetCodeNo.455 分发饼干
class Solution { public int findContentChildren(int[] g, int[] s) { Arrays.sort(g); Arrays.sort(s); int i=0,j=0; while(i<g.length&&j<s.length){ ...原创 2019-10-30 11:34:43 · 100 阅读 · 0 评论