1. 写法不同
const arrayFn = (a, b) => a + b;
function add(a, b) => {
return a + b;
}
2. 内部this的指向不同
window.a = 1;
const loga = () => {
const a = 2;
return this.a;
}
function fn() {
const a = 3;
return this.a;
}
loga();
fn();
const obj = {
loga,
fn,
a: "obj"
}
obj.loga();
obj.fn();
import React, { Component } from "react";
class Header extends Component {
constructor() {
super();
this.data = {
a: 1
}
}
log() {
console.log(this.data)
}
_log = () => { console.log(this.data) }
render() {
return (
<div>
<button onClick={this.log.bind(this)}>normal</button>
<button onClick={this._log}>array</button>
</div>
)
}
}
3. 普通函数可以当作构造函数来实例化,而箭头函数不行,因为箭头函数没有constructor
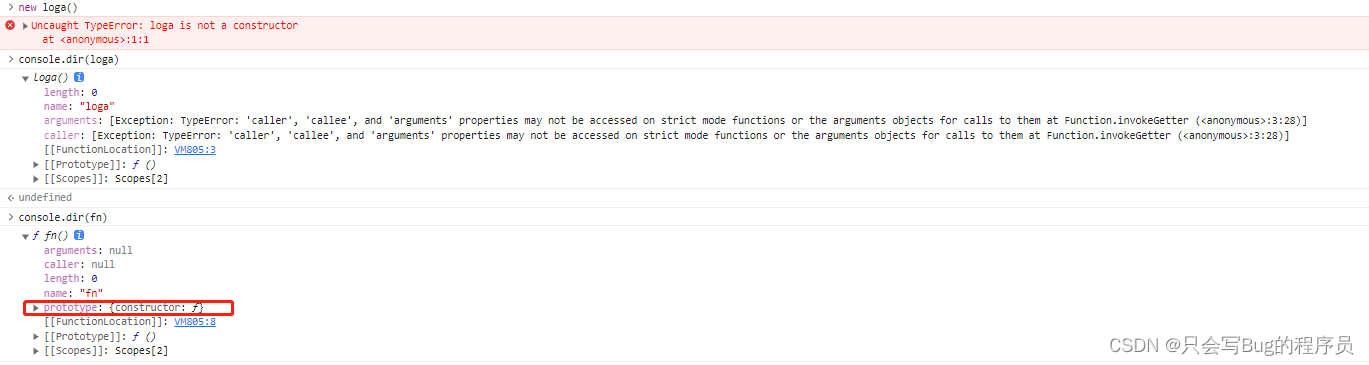
4. 普通函数可以通过bind,call,apply来指定this的指向,而箭头函数不行
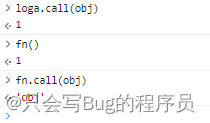
5. 在class中,定义普通函数和箭头函数的区别
class Father {
constructor(state) {
this.state = state
}
arrowMethod = () => {}
generalMethod() {}
};
class Son extends Father {
constructor(state) {
super(state)
}
};
const instance1 = new Father();
const instance2 = new Father();
const instance3 = new Son();
6. 普通函数中可以使用arguments变量获取所有的参数,而箭头函数中没有该变量,所以需要配合es6的扩展运算符取到所有参数
function add() {
let result = 0;
for(let i = 0; i < arguments.length; i++) {
result += arguments[i]
}
return result;
}
const arrayAdd = (...args) => args.reduce((prev, curr) => prev + curr, 0);
add(1,2,3,4,5)
arrayAdd(1,2,3,4,5)