#ifndef __RTC_H_
#define __RTC_H_
#include <stdint.h>
#if 0
用外部低速时钟作为时钟源
#endif
#define RTC_CLOCK_SOURCE_LSE
#ifdef RTC_CLOCK_SOURCE_LSI
#define RTC_ASYNCH_PREDIV 0x7C
#define RTC_SYNCH_PREDIV 0xF9
#endif
#ifdef RTC_CLOCK_SOURCE_LSE
#define RTC_ASYNCH_PREDIV 0x7F
#define RTC_SYNCH_PREDIV 0x00FF
#endif
void RTC_Init(void);
void RTC_CalendarShow(void);
#endif
#include <string.h>
#include <stdio.h>
#include "Rtc.h"
#include "stm32wbxx_hal.h"
#include "app_conf.h"
#include "ble_dbg_conf.h"
#include "dbg_trace.h"
RTC_HandleTypeDef hrtc;
#define RTC_DBG APP_DBG_MSG
void RTC_Init(void)
{
RTC_TimeTypeDef sTime = {0};
RTC_DateTypeDef sDate = {0};
hrtc.Instance = RTC;
hrtc.Init.HourFormat = RTC_HOURFORMAT_24;
hrtc.Init.AsynchPrediv = RTC_ASYNCH_PREDIV;
hrtc.Init.SynchPrediv = RTC_SYNCH_PREDIV;
if (HAL_RTC_Init(&hrtc) != HAL_OK)
{
}
#if 0
通过读备份寄存器的值判断是不是上电后RTC首次初始化
#endif
if (HAL_RTCEx_BKUPRead(&hrtc, RTC_BKP_DR0) != 0x32F2)
{
#if 0
初始化RTC时钟模块 设置时间和日期
#endif
sTime.Hours = 0x0D;
sTime.Minutes = 0x0;
sTime.Seconds = 0x0;
sTime.SubSeconds = 0x0;
sTime.DayLightSaving = RTC_DAYLIGHTSAVING_NONE;
sTime.StoreOperation = RTC_STOREOPERATION_RESET;
if (HAL_RTC_SetTime(&hrtc, &sTime, RTC_FORMAT_BCD) != HAL_OK)
{
}
sDate.WeekDay = RTC_WEEKDAY_TUESDAY;
sDate.Month = RTC_MONTH_AUGUST;
sDate.Date = 0x18;
sDate.Year = 0x20;
if (HAL_RTC_SetDate(&hrtc, &sDate, RTC_FORMAT_BCD) != HAL_OK)
{
}
#if 0
给备份寄存器写入一个值,用于软复位判断
#endif
HAL_RTCEx_BKUPWrite(&hrtc, RTC_BKP_DR0, 0x32F2);
}
else
{
if (__HAL_RCC_GET_FLAG(RCC_FLAG_BORRST) != RESET)
{
}
if (__HAL_RCC_GET_FLAG(RCC_FLAG_PINRST) != RESET)
{
}
}
__HAL_RCC_CLEAR_RESET_FLAGS();
}
void RTC_CalendarShow(void)
{
RTC_DateTypeDef sdatestructureget;
RTC_TimeTypeDef stimestructureget;
HAL_RTC_GetTime(&hrtc, &stimestructureget, RTC_FORMAT_BIN);
HAL_RTC_GetDate(&hrtc, &sdatestructureget, RTC_FORMAT_BIN);
RTC_DBG("\nTime %02d:%02d:%02d.\t", stimestructureget.Hours, stimestructureget.Minutes, stimestructureget.Seconds);
RTC_DBG("Data %2d-%02d-%02d.\n\n", 2000 + sdatestructureget.Year, sdatestructureget.Month, sdatestructureget.Date);
}
void StartDefaultTask(void *argument)
{
MAIN_DBG("2020/08/18 proj run!\r\n");
for(;;)
{
osDelay(1000);
RTC_CalendarShow();
}
}
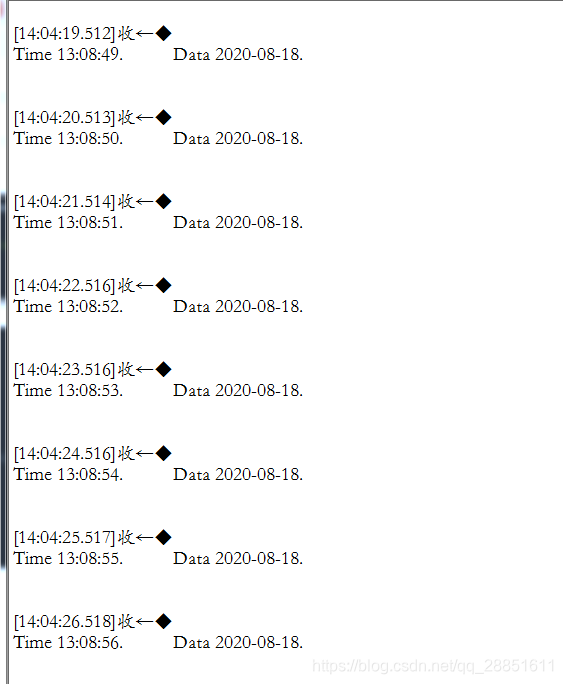