前言
这个项目的应用场景便是当某个公司要制作一个或者多个项目时,就需要针对性的将不同的员工分配到一个团队里。作为计算机开发团队,则架构师、设计师,程序员这三种人员是必不可少。所以此项目就是能做到将公司的人员添加到不同的团队中,并将团队在分配到项目里。
这个项目就是根据以往所学内容开发起来的,需要用到面向对象的封装、继承、多态,以及数组和集合,还需要熟悉使用接口和异常处理。我在做此项目的过程中也是源源不断的出现bug,所以在做这项目的小伙伴们,千万不要害怕,先从这个项目开始联手,咱一个一个解决它。这个项目是一个以文本的形式展示,存在菜单选项。下面就来看一下代码和过程吧。具体所学的内容可查看以往文章。
一.项目分析
1.1需要熟悉掌握的知识点
(1)首先对于面向对象中的封装、继承、多态要熟悉并且掌握
(2)接着对于对象、数据、值传递等要能够运用
(3)接口和类、类和类之间的关系,以及使用
(4)修饰符的运用:static、final
(5)java程序中异常的处理
(6)对于数组、列表的熟练掌握
1.2项目功能模块分析
我们来具体看一下系统总功能图
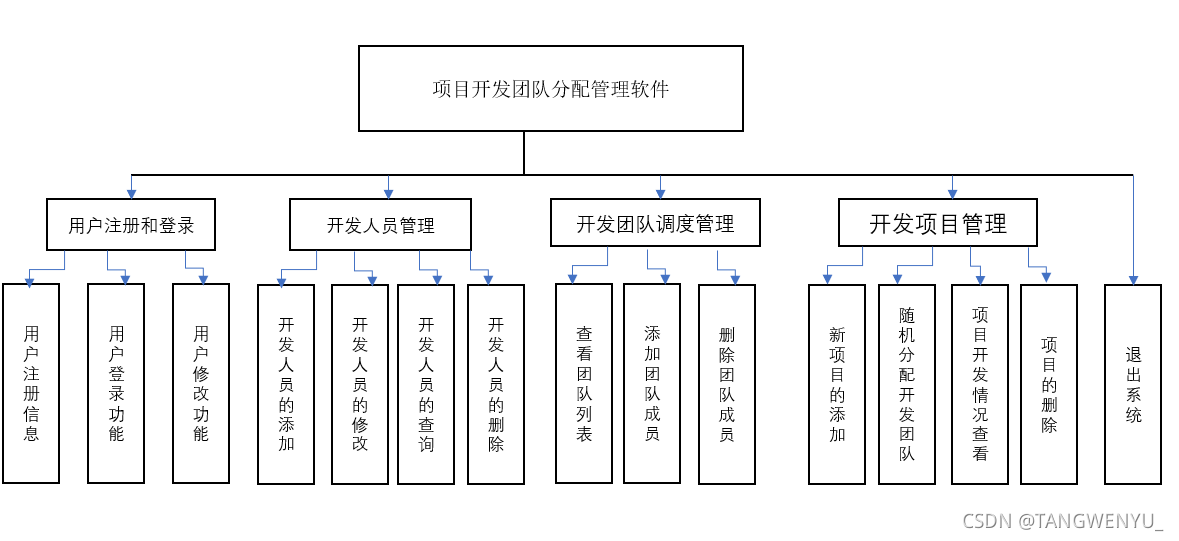
此项目分为四个模块:用户注册登录,开发人员管理、开发团队配置、开发项目管理。
首先在用户注册登录模块中 :当程序开启时,就会进入注册和登录界面,要先进行注册,再进行登录。在此我采用了ArrayList存放用户名及密码。
登陆成功后,剩余的三个模块以及用户修改模块就会以菜单的形式展现,用户们可以根据需要进行操作。
将项目代码分成三部分:实体类、操作类、界面展示类。即domain、serive,view。
具体结构为:
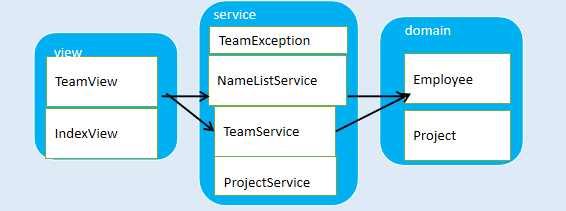
二.项目程序
2.1实体类分析
我先从实体类开始讲。公司需要有员工,所以需要有一个员工的实体类
这个实体类要有着员工最基础的四个熟悉
-id:int 作为员工的工号
-name:String 作为员工的名字
-age:int 作为员工的年龄
-salary:double 作为员工的月工资
public class Employee {
private int id;//工号
private String name;//姓名
private int age;//年龄
private double salary;//工资
public Employee() {
}
public Employee(int id, String name, int age, double salary) {
this.id = id;
this.name = name;
this.age = age;
this.salary = salary;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
protected String getDetails() { 输出形式
return id + "\t" + name + "\t" + age+ "\t\t" +salary;
}
@Override
public String toString() {//重写toString方法
return getDetails();
}
}
接着it公司最需要的就是程序员们,此时我们创建一个Programmer类
public class Programmer extends Employee{//程序员Programmer
private int memberId;
private boolean status = true;//员工状态
private Equipment equipment;//设备
public Programmer() {
}
public Programmer(int id, String name, int age, double salary, Equipment equipment) {
super(id, name, age, salary);
this.equipment = equipment;
}
public int getMemberId() {
return memberId;
}
public void setMemberId(int memberId) {
this.memberId = memberId;
}
public boolean getStatus() {
return status;
}
public void setStatus(boolean status) {
this.status = status;
}
public Equipment getEquipment() {
return equipment;
}
public void setEquipment(Equipment equipment) {
this.equipment = equipment;
}
protected String getMemberDetails(){
return getMemberId()+"/"+getDetails();
}
public String getDetailsForTeam(){
return getMemberDetails()+"\t程序员";
}
@Override
public String toString() {
return getDetails()+"\t程序员\t"+status+"\t\t\t\t\t"+equipment.getDescription();
}
}
公司有了程序员后,必不可免的也需要设计师的存在,才能带领程序员们开发出好的程序来,所以设计师会在程序员的基础上有着公司奖金。
public class Designer extends Programmer{
private double bonus;//奖金
public Designer() {
}
public Designer(int id, String name, int age, double salary, Equipment equipment, double bonus) {
super(id, name, age, salary, equipment);
this.bonus = bonus;
}
public double getBonus() {
return bonus;
}
public void setBonus(double bonus) {
this.bonus = bonus;
}
public String getDetailsForTeam(){
return getMemberDetails()+"\t设计师\t"+getBonus();
}
@Override
public String toString() {
return getDetails() + "\t设计师\t" + getStatus() + "\t" +
getBonus() +"\t\t\t" + getEquipment().getDescription();
}
}
一家it公司可不止要有设计师和程序员,在他们上面也需要有架构师领着做项目,所以架构师呀,程序员有的,设计师有的他都有,而且比设计师多出了一份公司的股票数量。所以接下来就是架构师类
public class Architect extends Designer{
private int stock;//股票数量
public Architect() {
}
public Architect(int id, String name, int age, double salary,
Equipment equipment, double bonus, int stock) {
super(id, name, age, salary, equipment, bonus);
this.stock = stock;
}
public int getStock() {
return stock;
}
public void setStock(int stock) {
this.stock = stock;
}
@Override
public String getDetailsForTeam() {
return getMemberDetails() + "\t架构师\t" +
getBonus() + "\t" + getStock();
}
@Override
public String toString() {
return getDetails() + "\t架构师\t" + getStatus() + "\t" +
getBonus() + "\t" + getStock() + "\t" + getEquipment().getDescription();
}
}
噢!对,身为it行业的人物,是必须要准备好设备,如笔记本电脑,台式电脑以及打印机。所以接下来就是设备
首先这个“设备” 是不是很抽象?它会是什么设备呢?真是不够具体,烦人。那我们把他设成一个接口吧!
public interface Equipment {
String getDescription();
}
它好像啥都没得,又好像啥都有,那设备是什么?接下来我们说一下笔记本电脑,说不定我就有所了解了。
public class NoteBook implements Equipment {
private String model;//机器的型号
private double price;//价格
public NoteBook() {
super();
}
public NoteBook(String model, double price) {
super();
this.model = model;
this.price = price;
}
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public NoteBook addNoteBook()
{
System.out.println("请输入需要配置的笔记本电脑的型号:");
String model = TSUtility.readKeyBoard(10, false);
System.out.println("请输入需要配置的笔记本电脑的价格:");
Double price = TSUtility.readDouble();
System.out.println("设备添加成功!");
return new NoteBook(model, price);
}
@Override
public String getDescription() {
return model + "(" + price + ")";
}
}
噢!原来如此,笔记本电脑实现了设备,使得设备不在那么抽象,而变成一种具体的设备,并且重写了方法。那接下来的台式电脑和打印机也一样吧
public class PC implements Equipment {
private String model;//机器型号
private String display;//显示器名称
public PC(){
super();
}
public PC(String model, String display) {
super();
this.model = model;
this.display = display;
}
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
public String getDisplay() {
return display;
}
public void setDisplay(String display) {
this.display = display;
}
//输入添加电脑的相关信息
public PC addPC()
{
System.out.println("请输入需要配置的台式电脑的型号:");
String model = TSUtility.readKeyBoard(10, false);
System.out.println("请输入需要配置的台式电脑的显示器名称:");
String display = TSUtility.readKeyBoard(10, false);
System.out.println("设备添加成功!");
return new PC(model, display);
}
@Override
public String getDescription() {
return model + "(" + display + ")";
}
}
public class Printer implements Equipment {
private String name;
private String type;
public Printer() {
}
public Printer(String name, String type) {
this.name = name;
this.type = type;
}
@Override
public String getDescription() {
return name + "(" + type + ")";
}
public Printer addPrinter() {
System.out.println("请输入需要配置的打印机的品牌:");
String name = TSUtility.readKeyBoard(10, false);
System.out.println("请输入需要配置的打印机的型号:");
String type = TSUtility.readKeyBoard(10, false);
System.out.println("设备添加成功!");
return new Printer(name,type);
}
}
果然如此!!!好像实体讲完了?不对,还有一个项目的实体
public class Project {
private int proId;//项目号
private String projectName;//项目名称
private String desName;//项目描述
private Programmer[] team;//开发团队
private String teamName;//开发团队名称
private boolean status=false;//true为开发中,false为未开发中
public Project() {
}
public Project(int proId, String projectName, String desName,
Programmer[] team, String teamName, boolean status) {
this.proId = proId;
this.projectName = projectName;
this.desName = desName;
this.team = team;
this.teamName = teamName;
this.status = status;
}
public int getProId() {
return proId;
}
public void setProId(int proId) {
this.proId = proId;
}
public String getProjectName() {
return projectName;
}
public void setProjectName(String projectName) {
this.projectName = projectName;
}
public String getDesName() {
return desName;
}
public void setDesName(String desName) {
this.desName = desName;
}
public Programmer[] getTeam() {
return team;
}
public void setTeam(Programmer[] team) {
this.team = team;
}
public String getTeamName() {
return teamName;
}
public void setTeamName(String teamName) {
this.teamName = teamName;
}
public boolean getStatus() {
return status;
}
public void setStatus(boolean status) {
this.status = status;
}
@Override
public String toString() {
return "项目{" +
"项目号='" + proId + '\'' +
"项目名='" + projectName + '\'' +
", 项目描述='" + desName + '\'' +
", 开发团队名称='" + teamName + '\'' +
", 开发状态=" + status +
'}' + "\n";
}
}
总算说完了实体了。接着说注册登录呢,这里我采用了ArrayList列表存储数据
先说一下登录吧
public class LoginView {
//定义登录用户名及密码
private String userName="";
private String passWord="";
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getPassWord() {
return passWord;
}
public void setPassWord(String passWord) {
this.passWord = passWord;
}
/**
这里我偷了个懒,其实上面也是应该单独作为一个实体类,大家千万不要这样学我啊!
**/
static ArrayList<LoginView> list = new ArrayList<>();
static LoginView lv = new LoginView();
static {
lv.setUserName("root");
lv.setPassWord("root");
list.add(lv);
}
/**这里我将list列表和lv新对象设为了静态,是为了方便static代码块中初始化数据*/
//注册功能
public void regist() throws InterruptedException {
TSUtility.loadSpecialEffects();
System.out.println("开始注册");
Scanner sc = new Scanner(System.in);
System.out.println("请输入你的注册账户名");
String userName=TSUtility.readKeyBoard(4,false);
//this.userName 指向全局变量 而赋值“=”右边为主方法体内的变量
lv.setUserName(userName);
// this.userName=userName;
System.out.println("请输入你的注册密码");
String passWord=TSUtility.readKeyBoard(8,false);
lv.setPassWord(passWord);
// this.passWord=passWord;
list.add(lv);
System.out.println("注册成功,请登录!");
}
//登录功能
public void login() throws InterruptedException {
int count=5;
boolean flag=true;
boolean flag1=true;
while (flag){//死循环
System.out.println("********************🐱");
System.out.println("*** <登录界面> ***");
System.out.println("*** (: ***🐱");
System.out.println("********************🐱");
System.out.println("请输入你的登录账户名称:");
String userName = TSUtility.readKeyBoard(4, false);
System.out.println("请输入你的登录密码:");
String password = TSUtility.readKeyBoard(8, false);
//检测列表的长度是否为1,和当前输入的账号密码是否为初始化的数据
if (list.size()==1 && (userName.equals("root")==false&&password.equals("root")==false)) {//未注册
System.out.println("未检测到您的账号,请您先注册!");
regist();//进入注册
}else if (userName.equals("root")&&password.equals("root")){
TSUtility.loadSpecialEffects();
System.out.println("登陆成功!欢迎您:" + userName);
flag = false;
break;
}else{
//已注册
for (int i = 0; i < list.size(); i++) {
if ((list.get(i).getUserName().equals(userName)) && list.get(i).getPassWord().equals(password)) {
TSUtility.loadSpecialEffects();
System.out.println("登陆成功!欢迎您:" + userName);
flag = false;
break;
}
//登录次数
else {
if (count <= 0) {
System.out.println("登录次数不足!退出!");
return;
} else {
count--;
System.out.println("登录失败!用户名或密码不匹配!");
System.out.println("登录次数还剩" + count + "次,请重新输入:");
break;
}
}
}
}
}
}
//修改功能
public void update() throws InterruptedException {
boolean flag = true;
while (flag) {
System.out.println(ANSI_RESET + ANSI_YELLOW);
System.out.println("********************🐱");
System.out.println("*** <修改界面> ***");
System.out.println("*** (: ***🐱");
System.out.println("********************🐱");
System.out.println("请输入你需要修改的类型:");
System.out.println("1(修改用户名)");
System.out.println("2(修改密码名)");
System.out.println("3(修改用户名和密码名)");
System.out.println("4(不修改,退出)");
Scanner sc = new Scanner(System.in);
String options = sc.next();
if (options.equals("1")) {
System.out.println("请输入你的修改的账户名称:");
String userName = TSUtility.readKeyBoard(4, false);
lv.setUserName(userName);
System.out.println("修改成功!");
} else if (options.equals("2")) {
System.out.println("请输入你的修改密码:");
String passWord = TSUtility.readKeyBoard(8, false);
lv.setPassWord(passWord);
System.out.println("修改成功!");
} else if (options.equals("3")) {
System.out.println("请输入你的修改的账户名称:");
String userName = TSUtility.readKeyBoard(4, false);
lv.setUserName(userName);
System.out.println("请输入你的修改密码:");
String passWord = TSUtility.readKeyBoard(8, false);
lv.setPassWord(passWord);
System.out.println("修改成功!");
}
else if (options.equals("4")) {
System.out.println("退出中");
TSUtility.loadSpecialEffects();
flag = false;
}
else {
System.out.println("输入错误!请输入“1”或者“2”或者“3”或者“4”:");
}
}
}
}
差点忘了给大家看看此项目的工具类了哈哈,这工具类在此项目中发挥了无与伦比的重要,大家可以参考参考
public class TSUtility {
private static Scanner scanner = new Scanner(System.in);
public static char readMenuSelection() {
char c;
for (; ; ) {
String str = readKeyBoard(1, false);
c = str.charAt(0);
if (c != '1' && c != '2' &&
c != '3' && c != '4' && c!='5') {
System.out.print("选择错误,请重新输入:");
} else break;
}
return c;
}
public static char readMenuSelectionPro() {
char c;
for (; ; ) {
String str = readKeyBoard(1, false);
c = str.charAt(0);
if (c != '1' && c != '2' &&
c != '3' && c != '4' && c != '5') {
System.out.print("选择错误,请重新输入:");
} else break;
}
return c;
}
public static void readReturn() {
System.out.print("按回车键继续...");
readKeyBoard(100, true);
}
public static int readInt() {
int n;
for (; ; ) {
String str = readKeyBoard(2, false);
try {
n = Integer.parseInt(str);
break;
} catch (NumberFormatException e) {
System.out.print("数字输入错误,请重新输入:");
}
}
return n;
}
public static int readstock() {
int n;
for (; ; ) {
String str = readKeyBoard(6, false);
try {
n = Integer.parseInt(str);
break;
} catch (NumberFormatException e) {
System.out.print("数字输入错误,请重新输入:");
}
}
return n;
}
public static Double readDouble() {
Double n;
for (; ; ) {
String str = readKeyBoard(6, false);
try {
n = Double.parseDouble(str);
break;
} catch (NumberFormatException e) {
System.out.print("数字输入错误,请重新输入:");
}
}
return n;
}
public static char readConfirmSelection() {
char c;
for (; ; ) {
String str = readKeyBoard(1, false).toUpperCase();
c = str.charAt(0);
if (c == 'Y' || c == 'N') {
break;
} else {
System.out.print("选择错误,请重新输入:");
}
}
return c;
}
public static String readString(int limit, String defaultValue) {
String str = readKeyBoard(limit, true);
return str.equals("")? defaultValue : str;
}
//可用于账号注册
public static String readKeyBoard(int limit, boolean blankReturn) {
String line = "";
while (scanner.hasNextLine()) {
line = scanner.nextLine();
if (line.length() == 0) {
if (blankReturn) return line;
else continue;
}
if (line.length() < 1 || line.length() > limit) {
System.out.print("输入长度(不大于" + limit + ")错误,请重新输入:");
continue;
}
break;
}
return line;
}
public static void loadSpecialEffects() throws InterruptedException {
System.out.println("请稍等:");
for (int i1 = 1; i1 <= 100; i1++) {
System.out.print("加载中:" + i1 + "%");
Thread.sleep(new Random().nextInt(25) + 1);
if (i1 == 100) {
Thread.sleep(50);
}
System.out.print("\r");
}
}
}
接下来我们看一下主界面显示
public class IndexView {
/**创建对象,再根据对象去调用里面的方法*/
private static LoginView lv = new LoginView();
private static NameListService nlv = new NameListService();
public static TeamView tv = new TeamView();
private static ProjectService pv = new ProjectService();
private ArrayList<Programmer[]> manyTeam=null;
/*
颜色特效
*/
public static final String ANSI_RESET = "\u001B[0m";
public static final String ANSI_YELLOW = "\u001B[33m";
public static final String ANSI_PURPLE = "\u001B[35m";
//项目的主菜单
private void MainMenu() throws TeamException, InterruptedException {
System.out.println(ANSI_PURPLE);
System.out.println("*******************************************");
System.out.println("* *");
System.out.println("* 欢迎来到项目开发团队分配管理软件 *");
System.out.println("* *");
System.out.println("*******************************************");
System.out.println("-----------<请您先进行登录>-------------");
System.out.println("");
System.out.println("");
System.out.println("");
TSUtility.readReturn();
try {
System.out.println(ANSI_PURPLE);
lv.login();
} catch (InterruptedException e) {
e.printStackTrace();
}
while (true) {//死循环
System.out.println(ANSI_RESET + ANSI_YELLOW);
System.out.println("~~~~~~~~~~~~~~软件主菜单~~~~~~~~~~~~~~~");
System.out.println(" 1. <用户信息修改> ");
System.out.println(" 2. <开发人员管理> ");
System.out.println(" 3. <开发团队调度管理> ");
System.out.println(" 4. <开发项目管理> ");
System.out.println(" 5. <退出软件> ");
System.out.println("请选择: ");
System.out.print(ANSI_RESET);
switch (TSUtility.readMenuSelectionPro()) {
case '1':
lv.update();//修改信息的入口
break;
case '2':
nlv.init();//人员管理入口
break;
case '3':
manyTeam=tv.getManyTeam(nlv);//开发团队配置入口
break;
case '4':
pv.init();//开发项目入口
break;
case '5':
System.out.print("确认是否退出(Y/N):");
if (TSUtility.readConfirmSelection() == 'Y') {
System.exit(0);
}
break;
}
}
}
public static Programmer[] dy(){//接收tv对象传过来的参数
return tv.pro1();
}
public static void main(String[] args) throws InterruptedException, TeamException {
IndexView iv = new IndexView();
iv.MainMenu();//程序的第一入口
}
}
根据功能图,接下来就是开发人员管理
public class NameListService {
private ArrayList<Employee> employees = new ArrayList<>();//存人员信息
private int count = 1;//记录序号
static NameListService listSvc = new NameListService();
//初始化人员名单
{
employees.add(new Employee(count, "马云 ", 22, 3000));
employees.add(new Architect(++count, "马化腾", 32, 18000, new NoteBook("联想T4", 6000), 60000, 5000));
employees.add(new Programmer(++count, "李彦宏", 23, 7000, new PC("戴尔", "NEC 17寸")));
employees.add(new Programmer(++count, "刘强东", 24, 7300, new PC("戴尔", "三星 17寸")));
employees.add(new Designer(++count, "雷军 ", 50, 10000, new Printer("激光", "佳能2900"), 5000));
employees.add(new Programmer(++count, "任志强", 30, 16800, new PC("华硕", "三星 17寸")));
employees.add(new Designer(++count, "柳传志", 45, 35500, new PC("华硕", "三星 17寸"), 8000));
employees.add(new Architect(++count, "杨元庆", 35, 6500, new Printer("针式", "爱普生20k"), 15500, 1200));
employees.add(new Designer(++count, "史玉柱", 27, 7800, new NoteBook("惠普m6", 5800), 1500));
employees.add(new Programmer(++count, "丁磊 ", 26, 6600, new PC("戴尔", "NEC17寸")));
employees.add(new Programmer(++count, "张朝阳 ", 35, 7100, new PC("华硕", "三星 17寸")));
employees.add(new Designer(++count, "杨致远", 38, 9600, new NoteBook("惠普m6", 5800), 3000));
}
//界面展示
public void init() {
while (true) {
System.out.println(ANSI_RESET + ANSI_YELLOW);
System.out.println("---------------开发人员管理菜单--------------");
System.out.println(" 1.<开发人员的添加> ");
System.out.println(" 2.<开发人员的查看> ");
System.out.println(" 3.<开发人员的修改> ");
System.out.println(" 4.<开发人员的删除> ");
System.out.println(" 5.<退出当前菜单> ");
System.out.println("请选择: ");
char c = TSUtility.readMenuSelectionPro();
switch (c) {
case '1':
addEmployee();
break;
case '2':
showEmployees();
break;
case '3':
updateEmployees();
break;
case '4':
deleteEmployee();
break;
case '5':
System.out.print("确认是否退出(Y/N):");
char b = TSUtility.readConfirmSelection();
if (b == 'Y') {
return;
}
}
}
}
//得到所有员工
public ArrayList<Employee> getAllEmployees() {
return employees;
}
//展示
public void showEmployees() {
System.out.println("ID\t姓名\t\t年龄\t\t工资\t\t 职位\t状态\t\t奖金\t\t股票\t\t领用设备");
for (int i = 0; i < employees.size(); i++) {
System.out.println(employees.get(i));
}
}
//查询指定员工
public Employee getEmployee(int id) throws TeamException {
for (int i = 0; i < employees.size(); i++) {
if (employees.get(i).getId() == id) {
return employees.get(i);
}
}
throw new TeamException("该员工不存在");
}
//增加员工
public void addEmployee() {
System.out.println("-----------------增加界面-----------------");
System.out.println(" 1 无职位 ");
System.out.println(" 2 程序员 ");
System.out.println(" 3 设计师 ");
System.out.println(" 4 架构师 ");
System.out.println(" 5 退出 ");
System.out.println("请选择:");
char a = TSUtility.readMenuSelectionPro();
String name;
int age;
double bonus;
switch (a) {
case '1':
System.out.print("请输入姓名:");
name = TSUtility.readKeyBoard(4, false);
System.out.print("请输入年龄:");
age = TSUtility.readInt();
System.out.print("请输入工资:");
double salary = TSUtility.readDouble();
employees.add(new Employee(++count, name, age, salary));
System.out.println("添加成功!!!");
break;
case '2':
System.out.print("请输入姓名:");
name = TSUtility.readKeyBoard(4, false);
System.out.print("请输入年龄:");
age = TSUtility.readInt();
System.out.print("请输入工资:");
salary = TSUtility.readDouble();
System.out.println("请为当前程序员配一台好的台式电脑:");
PC pc = new PC().addPC();
Programmer programmer = new Programmer(++count, name, age, salary, pc);
employees.add(programmer);
System.out.println("人员添加成功!");
break;
case '3':
System.out.print("请输入姓名:");
name = TSUtility.readKeyBoard(4, false);
System.out.print("请输入年龄:");
age = TSUtility.readInt();
System.out.print("请输入工资:");
salary = TSUtility.readDouble();
System.out.println("请为当前设计师配一台好的笔记本电脑:");
NoteBook noteBook = new NoteBook().addNoteBook();
System.out.println("请输入当前设计师的奖金:");
bonus = TSUtility.readDouble();
Designer designer = new Designer(++count, name, age, salary, noteBook, bonus);
employees.add(designer);
System.out.println("人员添加成功!");
break;
case '4':
System.out.print("请输入名字:");
name = TSUtility.readKeyBoard(4, false);
System.out.print("请输入年龄:");
age = TSUtility.readInt();
System.out.print("请输入工资:");
salary = TSUtility.readDouble();
System.out.println("请为当前架构师配一台好的打印设备:");
Printer printer = new Printer().addPrinter();
System.out.println("请输入当前架构师的奖金:");
bonus = TSUtility.readDouble();
System.out.println("请输入当前架构师的股票:");
Integer stock = TSUtility.readstock();
Architect architect = new Architect(++count, name, age, salary, printer, bonus, stock);
employees.add(architect);
System.out.println("人员添加成功!");
break;
case '5':
System.out.println("是否确认退出(输入Y/N):");
char b = TSUtility.readConfirmSelection();
if (b == 'Y') {
return;
}
}
}
//删除员工
public void deleteEmployee() {
System.out.print("请输入需要删除的员工id:");
int id = TSUtility.readInt();
boolean flag = false;
for (int i = 0; i < employees.size(); i++) {
if (employees.get(i).getId() == id) {
employees.remove(i);
for (i = id; i <= employees.size(); i++) {
employees.get(i - 1).setId(employees.get(i - 1).getId() - 1);
}
flag = true;
}
}
if (flag) {
System.out.println("删除成功!");
count--;
} else {
try {
throw new TeamException("该员工不存在");
} catch (TeamException e) {
e.printStackTrace();
}
}
}
public void updateEmployees() {
System.out.print("请输入需要修改的ID:");
int a = TSUtility.readInt();
if (a > count) {
try {
throw new TeamException("该员工不存在!!!");
} catch (TeamException e) {
e.printStackTrace();
}
return;
}
Employee b = employees.get(a - 1);
System.out.print("请输入新的姓名(" + employees.get(a - 1).getName() + "):");
String name = TSUtility.readString(4, b.getName());
System.out.print("请输入新的年龄(" + b.getAge() + "):");
int age = Integer.parseInt(TSUtility.readString(2, b.getAge() + ""));
System.out.print("请输入新的工资(" + b.getSalary() + "):");
double salary = Double.parseDouble(TSUtility.readString(10, b.getSalary() + ""));
b.setName(name);
b.setAge(age);
b.setSalary(salary);
employees.set(a - 1, b);
System.out.println("修改成功!!!");
}
}
接着是开发团队功能模块
//团队的操作
public class TeamService {
private static int counter = 1;//用于自动生成团队成员的memberId
private final int MAX_MEMBER = 5;//限制开发团队人数上限
private Programmer[] team = new Programmer[MAX_MEMBER];//保存当前团队成员
private int total = 0;//记录开发团队实际人数
public TeamService() {
}
//返回team中所有程序员构成的数组
public Programmer[] getTeam() {
Programmer[] team = new Programmer[total];
for (int i = 0; i < total; i++) {
team[i] = this.team[i];
}
return team;
}
//初始化当前团队成员数组
public void clearTeam() {
team = new Programmer[MAX_MEMBER];
counter=1;
total=0;
this.team = team;
}
//增加团队成员
public void addMember(Employee e) throws TeamException {
if (total>=MAX_MEMBER){
throw new TeamException("团队成员已满,无法添加!");
}
if (!(e instanceof Programmer)){
throw new TeamException("该成员不是开发人员,无法添加!");
}
Programmer p=(Programmer) e;//向下转型,以便于调用子类的成员变量
if (isExist(p)){
throw new TeamException("该成员已在本团队中");
}
if(!p.getStatus()) {
throw new TeamException("该员工已是某团队成员");
}
//添加团队
int numOfA=0,numOfDe=0,numOfPro=0;
for (int i = 0; i < total; i++) {
if (team[i] instanceof Architect){numOfA++;}
else if (team[i] instanceof Designer){numOfDe++;}
else if (team[i] instanceof Programmer){numOfPro++;}
}
if (p instanceof Architect){
if (numOfA>=1){
throw new TeamException("团队中至多只能有一名架构师");}}
else if (p instanceof Designer){
if (numOfDe>=2){
throw new TeamException("团队中至多只能有两名设计师");}}
else if (p instanceof Programmer){
if (numOfPro>=3){
throw new TeamException("团队中至多只能有三名程序员");}}
p.setStatus(false);
p.setMemberId(counter++);
team[total++]=p;
}
//判断该成员是否已经在团队里
public boolean isExist(Programmer p) {
for (int i = 0; i < total; i++) {
if (team[i].getId() == p.getId()){return true;}
}
return false;
}
//删除指定memberId的程序员
public void removeMember(int memberId) throws TeamException {
int n = 0;
//找到指定memberId的员工,并删除
for (; n < total; n++) {
if (team[n].getMemberId() == memberId) {
team[n].setStatus(true);
break;
}
}
//如果遍历一遍,都找不到,则报异常
if (n == total)
throw new TeamException("找不到指定的该成员,无法删除");
//后面的一个元素覆盖前面一个元素,实现删除操作
for (int i = n + 1; i < total; i++) {
team[i - 1] = team[i];
}
team[--total] = null;
}
}
//团队的界面展示
public class TeamView {
private NameListService lis = new NameListService();
private TeamService teamSvc = new TeamService();
private ArrayList<Programmer[]> team = new ArrayList<>();
private static IndexView I = new IndexView();
//主菜单
public void init() {
boolean loopFlag = true;
char key = 0;
do {
System.out.println(ANSI_RESET + ANSI_YELLOW);
if (key != '1') {
listAllEmployees();
}
System.out.println("1-团队成员列表 2-添加团队成员 3-删除团队成员 4-退出 请选择");
key = TSUtility.readMenuSelection();
System.out.println();
switch (key) {
case '1':
listTeam();
break;
case '2':
addMember();
break;
case '3':
deleteMember();
break;
case '4':
System.out.print("确认是否退出(Y/N):");
char yn = TSUtility.readConfirmSelection();
if (yn == 'Y') {
if (teamSvc.getTeam().length == 0) {
loopFlag = false;
} else {
team.add(teamSvc.getTeam());//获取存入team中所有程序员构成的数组,每调用一次,就在集合team里存入了一个团队
teamSvc.clearTeam();//每次退出都会初始化当前团队的成员数组 teamSvc,以便于下一次加入新的团队
loopFlag = false;
}
}
break;
default:
break;
}
} while (loopFlag);
}//分别调用了增删改查功能
public Programmer[] pro1(){//在TeamService取到数据并返回
return teamSvc.getTeam();
}
//显示所有的员工成员
public void listAllEmployees() {
System.out.println("~~~~~~~~~~~~~~欢迎来到团队调度软件~~~~~~~~~~~~~~~");
ArrayList<Employee> emps = lis.getAllEmployees();
if (emps.size() == 0) {
System.out.println("没有客户记录");
} else {
System.out.println("ID\t姓名\t\t工资\t\t职位\t\t状态\t\t奖金\t\t股票\t\t领用设备");
}
for (int i = 0; i < emps.size(); i++) {
System.out.println(" " + emps.get(i));
}
System.out.println("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~");
}
//显示开发团队成员列表
public void listTeam() {
System.out.println("~~~~~~~~~~~~~~~~团队成员列表~~~~~~~~~~~~~~~~");
Programmer[] team = teamSvc.getTeam();//获取team中所有程序员构成的数组
if (team.length == 0) {
System.out.println("开发团队目前没有成员!");
} else {
System.out.println("TID/ID\t姓名\t\t工资\t\t职位\t\t奖金\t\t股票");
}
for (int i = 0; i < team.length; i++) {//打印输出
System.out.println(" " + team[i].getDetailsForTeam());
}
System.out.println("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~");
}
// 添加成员到团队
private void addMember() {
System.out.println("---------------------添加成员---------------------");
System.out.print("请输入要添加的员工ID:");
int id = TSUtility.readInt();
try {
Employee e = lis.getEmployee(id);
teamSvc.addMember(e);//添加成员到团队
System.out.println("添加成功");
} catch (TeamException e) {
System.out.println("添加失败,原因:" + e.getMessage());
}
TSUtility.readReturn();
}
// 从团队中删除指定id的成员
private void deleteMember() {
System.out.println("---------------------删除成员---------------------");
System.out.print("请输入要删除员工的TID:");
int id = TSUtility.readInt();
System.out.print("确认是否删除(Y/N):");
char yn = TSUtility.readConfirmSelection();
if (yn == 'N')
return;
try {
teamSvc.removeMember(id);//改变员工的状态,表示将其移除团队
System.out.println("删除成功");
} catch (TeamException e) {
System.out.println("删除失败,原因:" + e.getMessage());
}
// 按回车键继续...
TSUtility.readReturn();
}
// 加入并得到更多的团队
public ArrayList<Programmer[]> getManyTeam(NameListService nameListSer) {
boolean flag = true;
char key = 0;
lis = nameListSer;//将传入的对象,赋值给lis ,使得lis可以用到参数对象里的 数据
do {
System.out.println(ANSI_RESET + ANSI_YELLOW);
System.out.println("※※※※※※※※※※※");
System.out.println("※ 团队调度界面 ※");
System.out.println("※※※※※※※※※※※");
System.out.print("1-添加团队 2-查看团队 3-删除团队 4-退出 请选择(1-4):");
key = TSUtility.readMenuSelection();
System.out.println();
switch (key) {
case '1':
init();//
break;
case '2':
System.out.println("~~~~~~~~~~~~~~~~~~团队列表~~~~~~~~~~~~~~~~~~~~~~");
for (Programmer[] team : team) {
for (int i = 0; i < team.length; i++) {
System.out.println(team[i]);
}
System.out.println("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~");
}
break;
case '3':
System.out.println("请输入想要删除第几个团队");
int num = TSUtility.readInt();
if (num <= team.size()) {
System.out.print("确认是否删除(Y/N):");
char de = TSUtility.readConfirmSelection();
if (de == 'Y') {
team.remove(num - 1);
} else {
System.out.println("请确认!");
}
} else {
System.out.println("没有该团队,请正常输入!" + "目前团队只有" + team.size() + "个");
}
break;
case '4':
System.out.print("确认是否退出(Y/N):");
char yn = TSUtility.readConfirmSelection();
if (yn == 'Y') {
flag = false;
}
break;
default:
break;
}
} while (flag);
return team;
}//调用了主菜单的功能
}
最后是项目的展示及操作
public class ProjectService {
//用来存项目的集合
private ArrayList<Project> pro = new ArrayList<>();
// TeamView tv1= IndexView.tv;
//添加项目的标号
private int count = 1;
public void init() throws InterruptedException {
while (true) {
System.out.println("~~~~~~~开发项目管理主菜单~~~~~~~~~ ");
System.out.println("1. <项目的添加> ");
System.out.println("2. <项目分配开发团队> ");
System.out.println("3. <项目的查看> ");
System.out.println("4. <项目的删除> ");
System.out.println("5. <退出当前菜单> ");
System.out.print("请选择:");
switch (TSUtility.readMenuSelectionPro()) {
case '1':
addProjectView();
break;
case '2':
dealingProView(IndexView.dy());
break;
case '3':
showPro();
break;
case '4':
delProView();
break;
case '5':
System.out.print("确认是否退出(Y/N):");
if (TSUtility.readConfirmSelection() == 'Y') {
return;
}
break;
}
}
}
private void dealingProView() {
// dealingProView();
}
//项目的添加交互界面
public void addProjectView() throws InterruptedException {
System.out.println("项目参考:");
System.out.println("1.小米官网:开发完成类似于小米官网的web项目.");
System.out.println("2.公益在线商城:猫宁Morning公益商城是中国公益性在线电子商城.");
System.out.println("3.博客系统:Java博客系统,让每一个有故事的人更好的表达想法!");
System.out.println("4.在线协作文档编辑系统:一个很常用的功能,适合小组内的文档编辑。");
System.out.println("------------------------------------------------------------");
TSUtility.readReturn();
System.out.println("请输入你想添加的项目名: ");
char c = TSUtility.readMenuSelection();
switch (c) {
case '1':
Project p1 = new Project();
p1.setProId(count++);
p1.setProjectName("小米官网");
p1.setDesName("开发完成类似于小米官网的web项目.");
pro.add(p1);
TSUtility.loadSpecialEffects();
System.out.println("已添加项目:" + p1.getProjectName());
break;
case '2':
Project p2 = new Project();
p2.setProId(count++);
p2.setProjectName("公益在线商城");
p2.setDesName("猫宁Morning公益商城是中国公益性在线电子商城.");
pro.add(p2);
TSUtility.loadSpecialEffects();
System.out.println("已添加项目:"+p2.getProjectName());
break;
case '3':
Project p3 = new Project();
p3.setProId(count++);
p3.setProjectName("博客系统");
p3.setDesName("Java博客系统,让每一个有故事的人更好的表达想法!");
pro.add(p3);
TSUtility.loadSpecialEffects();
System.out.println("已添加项目:"+p3.getProjectName());
break;
case '4':
Project p4 = new Project();
p4.setProId(count++);
p4.setProjectName("在线协作文档编辑系统");
p4.setDesName("一个很常用的功能,适合小组内的文档编辑。");
pro.add(p4);
TSUtility.loadSpecialEffects();
System.out.println("已添加项目:"+p4.getProjectName());
break;
default:
System.out.println("项目不存在");
break;
}
}
//项目分配开发团队交互界面
public void dealingProView(Programmer[] team) {
//dealingProView
if (pro.size()==0){
System.out.println("请先添加项目");
}
else {
System.out.println("当前团队有人员:");
for (int i = 0; i < team.length; i++) {
System.out.println(team[i]);
}
System.out.println("请为当前团队创建一个团队名称:");
String teamName = TSUtility.readKeyBoard(6, false);
//随机分配项目
Random ra = new Random();
int ranNum = ra.nextInt(pro.size());
Project project = this.pro.get(ranNum);
// while (project.getStatus()){ //使用while循环,判断如果项目已经开发,重新读取
// ranNum= ra.nextInt(pro.size());//重新获取随机数
// project=this.pro.get(ranNum);//集合重新取出随机项目
// }
project.setTeamName(teamName);
project.setTeam(team);
project.setStatus(true);
pro.set(ranNum, project);
System.out.println();
}
}
//项目的查看
private void showPro() {
for (Project p : pro) {
if (p.getStatus()) {
System.out.println("项目【" + p.getProjectName() + "】---->正在被团队【" + p.getTeamName() + "】开发中!");
} else {
System.out.println(p + "\n项目【" + p.getProjectName() + "】---->未被开发!");
}
}
}
//项目的删除交互界面
private void delProView() {
boolean flag = false;
System.out.print("请输入需要删除的项目id:");
int id = TSUtility.readInt();
for (int i = 0; i < pro.size(); i++) {
if (pro.get(i).getProId() == id) {
pro.remove(i);
for (i = id; i <= pro.size(); i++) {
pro.get(i - 1).setProId(pro.get(i - 1).getProId() - 1);
}
flag = true;
}
}
if (flag) {
System.out.println("删除成功!");
count--;
} else {
try {
throw new TeamException("该项目不存在");
} catch (TeamException e) {
e.printStackTrace();
}
}
}
//得到所有项目数据集合
public ArrayList<Project> getAllPro() {
return pro;
}
}
最后的最后哈,大家千万别忘了自定义异常抛出了!!!感谢各位的观看,点个赞再走呗
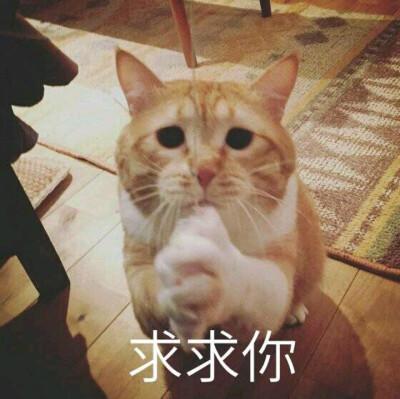
以上均是个人学习心得,若是有什么地方写的不对,也欢迎大家也可以多指教、谢谢大家的观看