面试题:最长公共子序列——Java
算法思路:滑动窗口(双指针)
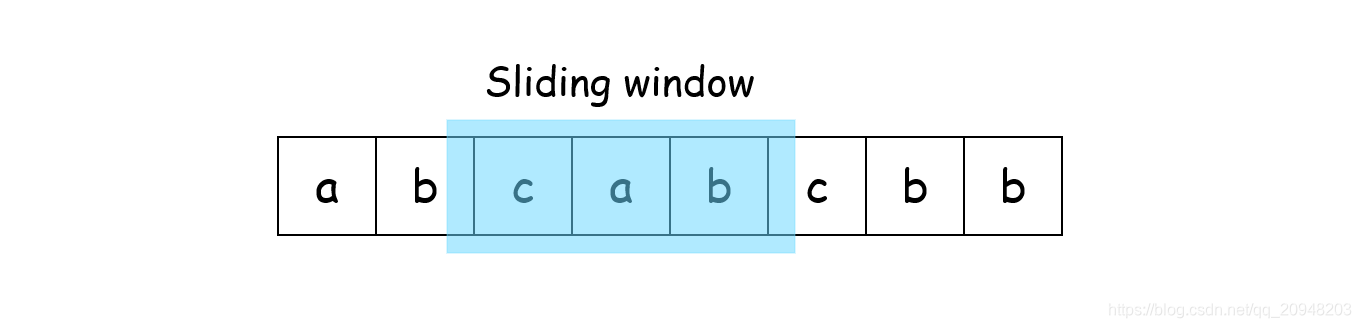
- 1.初始化头尾指针 head,tail;
- 如果窗口中没有该元素,则将该元素加入窗口,同时更新窗口长度最大值,tail 指针继续右移;
- 项目如果窗口中存在该元素,则将 head 指针右移,直到窗口中不包含该元素。
- 2.tail 指针右移,判断 tail 指向的元素是否在 [head:tail] 的窗口内;
- 3.返回窗口长度的最大值。
class Solution {
public int lengthOfLongestSubstring(String s) {
int n=s.length(),ans=0;
Map<Character,Integer>map=new HashMap<>();
for(int start=0,end=0;end<n;end++){
if(map.containsKey(s.charAt(end))){
start=Math.max(start,map.get(s.charAt(end))+1);
//重复的字符可能在start前面,也可能在后面
}
ans=Math.max(ans,end-start+1);
map.put(s.charAt(end),end);
//每次更新Value对应的位置
}
return ans;
}
}