栈:
源代码:
#include<stdio.h>
#include<malloc.h>
#define max 50
typedef struct
{
char data[max];
int top;
}sqStack;
void InitStack(sqStack *&s)
{
s=(sqStack*)malloc(sizeof(sqStack));
s->top=-1;
printf("初始化成功!\n");
}
void sqStackEmpty(sqStack *s)
{
if(s->top==-1)
printf("栈中为空\n");
else
printf("栈不为空\n");
}
int push(sqStack *&s,char e)
{
if(s->top==max-1)
{
printf("栈满,进栈失败\n");
return 0;
}
s->top++;
s->data[s->top]=e;
printf("进栈成功\n");
return 0;
}
int pop(sqStack *&s)
{
if(s->top==-1)
{
printf("栈为空\n");
return 0;
}
printf("%3c出栈成功\n",s->data[s->top]);
s->top--;
return 0;
}
void Destroy(sqStack *& s)
{
free(s);
printf("释放栈成功!\n");
}
int pop_Stacksequence(sqStack *s)
{
int p;
if(s->top==-1)
{
printf("栈为空\n");
return 0;
}
printf("栈顶到栈底元素为 ");
for(p=s->top;s->data[p]!=NULL;p--)
{
printf("%4c",s->data[p]);
}
printf("\n");
}
int length(sqStack *s)
{
int p;int k=0;
if(s->top==-1)
{
printf("栈为空\n");
return 0;
}
for(p=s->top;s->data[p]!=NULL;p--)
{
k++;
}
printf("共有%d个元素\n",k);
return 0;
}
int main()
{
sqStack *s;int i=5;
char e;
InitStack( s);
sqStackEmpty(s);
for(int j=0;j<i;j++)
{
printf("请输入要插入的元素 : ");
scanf("%c",&e);
push(s,e);
fflush(stdin);
}
sqStackEmpty(s);
length(s);
pop_Stacksequence(s);
for(int j=0;j<i;j++)
{
pop(s);
}
sqStackEmpty(s);
Destroy(s);
return 0;
}
实验截图:
队列:
源代码:
#include<stdio.h>
#include<malloc.h>
typedef struct qnode
{
char data;
struct qnode *next;
}Qnode;
typedef struct
{
Qnode *front;
Qnode *rear;
}LiQueue;
void InitQueue(LiQueue *&q)
{
q=(LiQueue*)malloc(sizeof(LiQueue));
q->front=q->rear=NULL;
printf("初始化...成功\n");
}
void DestroyQueue(LiQueue *&q)
{
Qnode *p=q->front,*r;
if(p!=NULL)
{
r=p->next;
while(r!=NULL)
{
free(p);
p=r;r=p->next;
}
free(p);
free(q);
printf("释放队列成功!\n");
}
}
void QueueEmpty(LiQueue *q)
{
if(q->rear==NULL)
printf("队列为空\n");
else
printf("队列不为空 \n");
}
void enQueue(LiQueue *&q,int n)
{
Qnode *p;
int i=0;
char e[50];
char enter;
for(i=0;i<n;i++)
{
printf("请输入要插入的元素:\n");
fflush(stdin);
scanf("%c",&e[i]);
p=(Qnode*)malloc(sizeof(Qnode));
p->data=e[i];
p->next=NULL;
if(q->rear==NULL)
{
q->front=q->rear=p;
}
else
{
q->rear->next=p;
q->rear=p;
}
}
}
int deQueue(LiQueue *& q)
{
if(q->rear==NULL)
return 0;
printf("删除元素为%c\n",q->front->data);
if(q->front==q->rear)
q->front=q->rear=NULL;
else
q->front=q->front->next;
return 0;
}
void length(LiQueue * q)
{
Qnode *p;
int i=0;
p=q->front;
while(p!=NULL)
{
i++;
p=p->next;
}
printf("队列长度为 %d\n",i);
}
void disDequeue(LiQueue * q)
{
Qnode *p;
p=q->front;
printf("出队序列为: ");
while(p!=NULL)
{
printf("%4c",p->data);
p=p->next;
}
printf("\n");
}
int main()
{
int n=3;
LiQueue *q;
InitQueue(q);
QueueEmpty(q);
enQueue(q,n);
deQueue(q);
length(q);
enQueue(q,n);
disDequeue(q);
QueueEmpty(q);
length(q);
DestroyQueue(q) ;
return 0;
}
/*编译问题:键盘缓冲区问题,字符类型读取是把回车也读取了*/
实验截图:
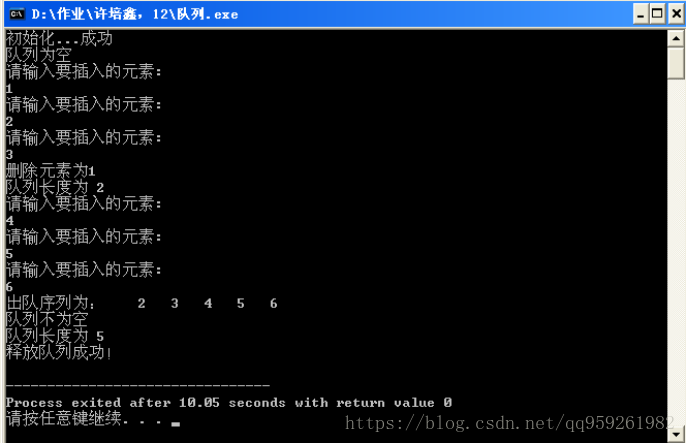