一、简单工厂
1 - 普通场景
- 场景:客户到餐厅就餐,可以选择的有东来顺的饭菜和庆丰包子铺饭菜
- UML:
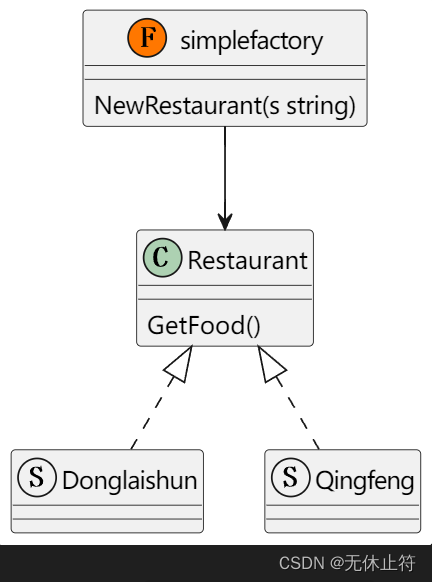
type Restaurant interface {
GetFood()
}
type Donglaishun struct {
}
func (*Donglaishun) GetFood() {
fmt.Println("东来顺的饭菜准备就绪")
}
type Qingfeng struct{}
func (*Qingfeng) GetFood() {
fmt.Println("庆丰包子铺饭菜准备就绪")
}
func NewRestaurant(s string) Restaurant {
switch s {
case "d":
return &Donglaishun{}
case "q":
return &Qingfeng{}
}
return nil
}
func main() {
factory.NewRestaurant("d").GetFood()
factory.NewRestaurant("q").GetFood()
}
2 - 游戏场景
- 游戏场景:玩家角色创建,可以创建战士职业也可以创建魔法师职业
- UML
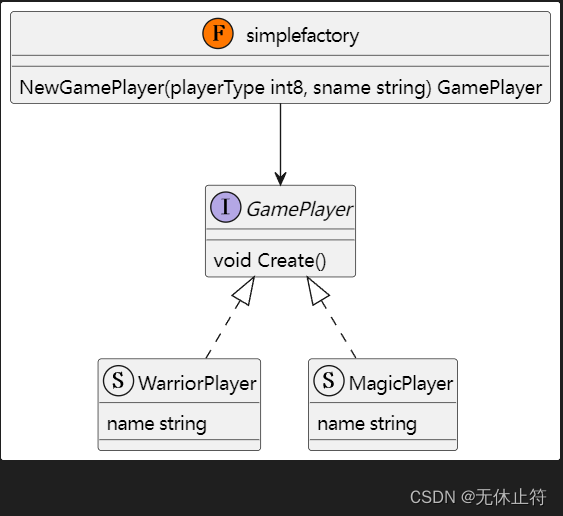
type GamePlayer interface {
Create()
}
type WarriorPlayer struct {
name string
}
func (w *WarriorPlayer) Create() {
fmt.Printf("WarriorPlayer is Created,name is %s\n", w.name)
}
type MagicPlayer struct {
name string
}
func (m *MagicPlayer) Create() {
fmt.Printf("MagicPlayer is Created,name is %s\n", m.name)
}
func NewGamePlayer(playerType int8, sname string) GamePlayer {
switch playerType {
case 1:
return &WarriorPlayer{
name: sname,
}
case 2:
return &MagicPlayer{
name: sname,
}
}
return nil
}
const (
TYPE_WARRIOR = 1
TYPE_MAGIC = 2
)
func main() {
factory.NewGamePlayer(TYPE_WARRIOR, "jack").Create()
factory.NewGamePlayer(TYPE_MAGIC, "tom").Create()
}
二、抽象工厂
- 概念:抽象工厂模式实质上对简单Factory进行再抽象,通过在AbstarctFactory中增加创建产品的接口,然后在具体子工厂中实现新加产品的创建
1 - 普通场景
- 场景:煮饭 -> 煮大米、煮番茄
- UML:
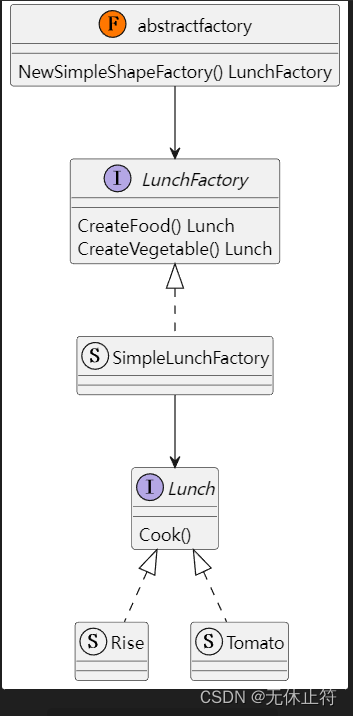
package abstractory
import "fmt"
type Lunch interface {
Cook()
}
type Rise struct {
}
func (r *Rise) Cook() {
fmt.Println("cook rise")
}
type Tomato struct {
}
func (t *Tomato) Cook() {
fmt.Println("cook tomato")
}
type LunchFactory interface {
CreateFood() Lunch
CreateVegetable() Lunch
}
type SimpleLunchFactory struct {
}
func (s *SimpleLunchFactory) CreateFood() Lunch {
return &Rise{}
}
func (s *SimpleLunchFactory) CreateVegetable() Lunch {
return &Tomato{}
}
func NewSimpleShapeFactory() LunchFactory {
return &SimpleLunchFactory{}
}
func main() {
abfactory := abstractory.NewSimpleShapeFactory()
food := abfactory.CreateFood()
food.Cook()
vegetable := abfactory.CreateVegetable()
vegetable.Cook()
}
2 - 游戏场景
- 场景:根据任务类型处理业务,我们在处理不同类型任务的时候,需要初始化以及交接等情况
- UML:
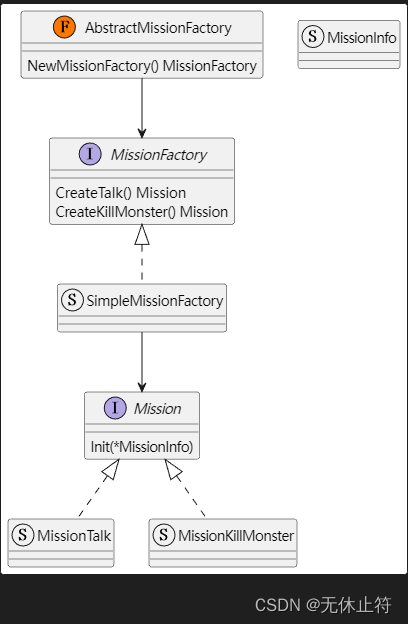
type MissionInfo struct {
ID uint64
}
type MissionFactory interface {
CreateTalk() Mission
CreateKillMonster() Mission
}
type SimpleMissionFactory struct {
}
func (s *SimpleMissionFactory) CreateTalk() Mission {
return &MissionTalk{}
}
func (s *SimpleMissionFactory) CreateKillMonster() Mission {
return &MissionKillMonster{}
}
type Mission interface {
Init(*MissionInfo)
}
type MissionTalk struct {
}
func (m *MissionTalk) Init(s *MissionInfo) {
fmt.Printf("MissionTalk init id=%d\n", s.ID)
}
type MissionKillMonster struct {
}
func (m *MissionKillMonster) Init(s *MissionInfo) {
fmt.Printf("MissionKillMonster init id=%d\n", s.ID)
}
func NewMissionFactory() MissionFactory {
return &SimpleMissionFactory{}
}
func main() {
minfotalk := &abstractory.MissionInfo{
ID: 100,
}
minfokill := &abstractory.MissionInfo{
ID: 101,
}
mission := abstractory.NewMissionFactory()
talk := mission.CreateTalk()
talk.Init(minfotalk)
killmonster := mission.CreateKillMonster()
killmonster.Init(minfokill)
}