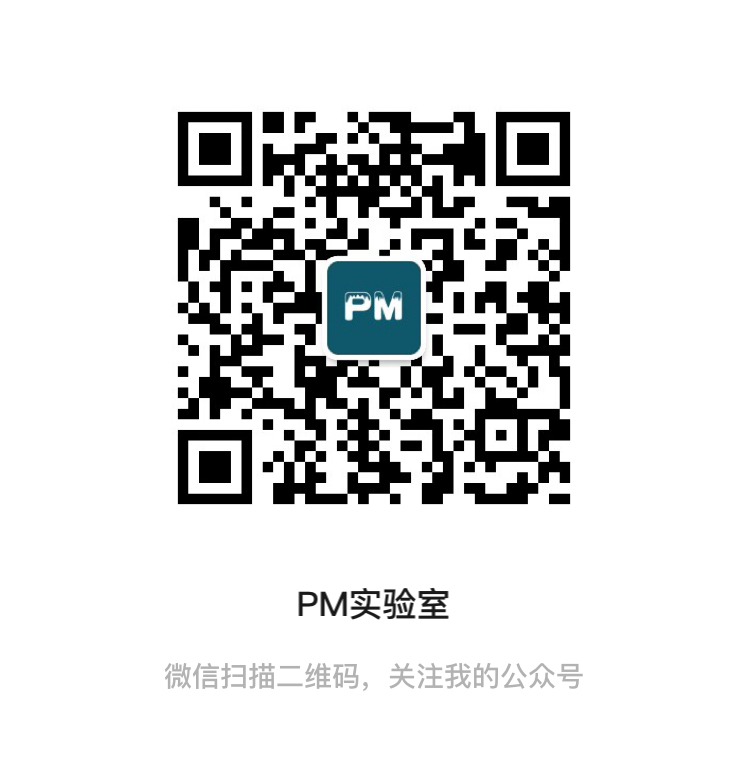
我编写工具类所参考的文档
pache HttpClient:
http://hc.apache.org/httpcomponents-client-ga/index.html
中文翻译教程:
https://www.yiibai.com/apache_httpclient
stackoverflow 关于SSL的讨论:
https://stackoverflow.com/questions/19517538/ignoring-ssl-certificate-in-apache-httpclient-4-3
代码要点
1、基于httpclient 4.5.8,代码无deprecated
2、GET请求 + POST请求
3、ResponseHandler处理响应,自动释放所有HTTP连接
4、忽略验证SSL证书来处理Https请求
5、请求携带CookieStore
参考代码(java)
public class HttpClientUtils {
private static final Logger log = LoggerFactory.getLogger(HttpClientUtils.class);
/**
* POST 请求
*
* @param uri URI
* @return response
* @throws IOException
*/
public static String doPost(String uri) throws IOException {
CloseableHttpClient httpClient = createHttpClient("", true, 10);
//创建post方式请求对象
HttpPost httpPost = new HttpPost(uri);
httpPost.setHeader("Content-type", "application/x-www-form-urlencoded");
httpPost.setHeader("User-Agent", "Mozilla/5.0 (Windows NT 6.1; rv:6.0.2) Gecko/20100101 Firefox/6.0.2");
ResponseHandler<String> responseHandler = new ResponseHandler<String>() {
@Override
public String handleResponse(HttpResponse response) throws ClientProtocolException, IOException {
int status = response.getStatusLine().getStatusCode();
if (status >= 200 && status < 300) {
HttpEntity entity = response.getEntity();
return entity != null ? EntityUtils.toString(entity, "UTF-8") : null;
} else {
log.error("Unexpected response status: " + status);
throw new ClientProtocolException("Unexpected response status: " + status);
}
}
};
String response = httpClient.execute(httpPost, responseHandler);
return response;
}
/**
* Get 请求
*
* @param uri URI
* @return response
* @throws IOException
*/
public static String doGet(String uri) throws IOException {
CloseableHttpClient httpClient = createHttpClient("", true, 10);
HttpGet httpGet = new HttpGet(uri);
httpGet.setHeader("Content-type", "application/x-www-form-urlencoded");
httpGet.setHeader("User-Agent", "Mozilla/5.0 (Windows NT 6.1; rv:6.0.2) Gecko/20100101 Firefox/6.0.2");
ResponseHandler<String> responseHandler = new ResponseHandler<String>() {
@Override
public String handleResponse(HttpResponse response) throws ClientProtocolException, IOException {
int status = response.getStatusLine().getStatusCode();
if (status >= 200 && status < 300) {
HttpEntity entity = response.getEntity();
return entity != null ? EntityUtils.toString(entity, "UTF-8") : null;
} else {
log.error("Unexpected response status: " + status);
throw new ClientProtocolException("Unexpected response status: " + status);
}
}
};
String response = httpClient.execute(httpGet, responseHandler);
return response;
}
/**
* @param sessionId 会话ID
* @param automaticRedirect 自动重定向
* @param timeOut 超时时间
* @return CloseableHttpClient
*/
private static CloseableHttpClient createHttpClient(String sessionId, boolean automaticRedirect, int timeOut) {
//忽略校验HTTPS服务器证书, 将hostname校验和CA证书校验同时关闭
SSLContext sslContext = null;
try {
sslContext = SSLContexts.custom()
.loadTrustMaterial(TrustSelfSignedStrategy.INSTANCE)
.build();
} catch (NoSuchAlgorithmException | KeyManagementException | KeyStoreException e) {
log.error("HttpClientUtils.createHttpClient: " + e);
}
//设置http和https协议对应的处理socket链接工厂的对象
Registry<ConnectionSocketFactory> registry = RegistryBuilder.<ConnectionSocketFactory>create()
.register("http", PlainConnectionSocketFactory.INSTANCE)
.register("https", new SSLConnectionSocketFactory(sslContext, NoopHostnameVerifier.INSTANCE))
.build();
PoolingHttpClientConnectionManager connectionManager = new PoolingHttpClientConnectionManager(registry);
//构建请求配置信息
timeOut = timeOut * 1000;
RequestConfig requestConfig = RequestConfig.custom()
.setSocketTimeout(timeOut) //获取数据的超时时间
.setConnectTimeout(timeOut) //建立连接的超时时间
.setConnectionRequestTimeout(timeOut) //连接池获取到连接的超时时间
.build();
//创建自定义httpclient对象
HttpClientBuilder builder = HttpClients.custom();
builder.setDefaultRequestConfig(requestConfig); //设置默认请求配置
builder.setConnectionManager(connectionManager); //设置连接管理器
if (!automaticRedirect) { //设置自动重定向配置
builder.disableRedirectHandling();
}
//配置CookieStore
CookieStore cookieStore = null;
if (StringUtils.isNotBlank(sessionId)) {
//TODO...redis查找cookieStore
if (cookieStore == null) {
cookieStore = new BasicCookieStore();
}
builder.setDefaultCookieStore(cookieStore);
}
CloseableHttpClient httpClient = builder.build();
return httpClient;
}
}