目录
简介
spring boot 整合缓存服务redis 注解方式使用。整合服务请参考上篇文章Spring-boot 整合缓存服务之Redis简单集成。
注解
@Cacheable
读取缓存数据,无数据则会更新缓存中数据。配置在方法或类上。作用:本方法执行后,先去缓存看有没有数据,如果没有,从数据库中查找出来,给缓存中存一份,返回结果会将方法的返回值作为value进行缓存,下次本方法执行,在缓存未过期情况下,先在缓存中查找,有的话直接返回,没有的话从数据库查找
注解参数
参数 | 类型 | 解释 | 样例 |
---|---|---|---|
value | String[] | 缓存位置名称(可以认为其为组的概念) | @Cacheable(value = "CREATE_NUMBER_RECORDING", key = "#infoDate",unless="#result == null") |
cacheNames | String[] | 同上方value同理(具体使用请参考@CacheConfig) | @Cacheable(cacheNames= "CREATE_NUMBER_RECORDING", key = "#infoDate",unless="#result == null") |
key | String | 缓存键,可使用“:”分割,分割后为组的概念,#后为方法上的参数名称,如果参数为对象,则#对象.get对应的属性值 | @Cacheable(value = "CREATE_NUMBER_RECORDING", key = "#infoDate+':'+#companyId+':'+#type+':'+#param1") |
keyGenerator | String | 自定义生成key方式,与key()不可以共用。其值为spring注入的类,其类实现implements KeyGenerator 接口 | @Cacheable(cacheNames = "ids_cache",keyGenerator = "setKeyGrenerator") |
cacheManager | String | -- | -- |
cacheResolver | String | -- | -- |
condition | String | 使用SpEL表达式设定出发缓存的条件,在方法执行前生效 | |
unless | String | 使用SpEL设置出发缓存的条件,方法执行完生效,所以条件中可以有方法执行后的value。函数返回值符合条件的排除掉、只缓存其余不符合条件的 | @Cacheable(unless="#result == null") 排除值为null 的情况 |
sync | boolean | 是否同步,默认false |
代码案例
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.zbintel.mom.factory.dao.NumberCreateDao;
import com.zbintel.mom.factory.entity.NumberCreateEntity;
import com.zbintel.mom.factory.service.NumberCreateCacheService;
import org.springframework.cache.annotation.CachePut;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service("numberCreateCacheService")
public class NumberCreateCacheServiceImpl extends ServiceImpl<NumberCreateDao, NumberCreateEntity> implements NumberCreateCacheService {
/**
* 设置其 value : CREATE_NUMBER_RECORDING (组的概念)
* key : infoDate:companyId:type (获取请求参数中的对应值, : 号分割后给其进行组分割)
* unless : 排除result 为 null的情况
* @param companyId
* @param type
* @param infoDate
* @return
*/
@Cacheable(value = "CREATE_NUMBER_RECORDING", key = "#infoDate+':'+#companyId+':'+#type+':'+#param1",unless="#result == null")
public NumberCreateEntity queryCache(String companyId,String type, String param1, String infoDate) {
QueryWrapper<NumberCreateEntity> queryWrapper = new QueryWrapper<>();
queryWrapper.lambda().eq(NumberCreateEntity::getType,type).eq(NumberCreateEntity::getInfoDate,infoDate)
.eq(NumberCreateEntity::getCompanyId,companyId).eq(NumberCreateEntity::getParam1,param1);
return baseMapper.selectOne(queryWrapper);
}
}
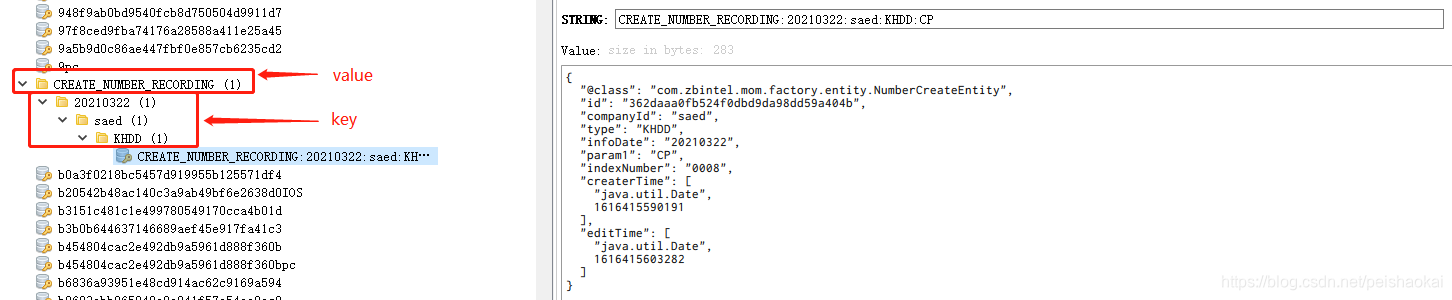
@CachePut
更新缓存中的值。配置到方法上。 类似于更新操作,即每次不管缓存中有没有结果,都从数据库查找结果,并将结果更新到缓存,并返回结果。
注解参数
参数 | 类型 | 解释 | 样例 |
---|---|---|---|
value | String[] | 缓存位置名称(可以认为其为组的概念) | @Cacheable(value = "CREATE_NUMBER_RECORDING", key = "#infoDate",unless="#result == null") |
cacheNames | String[] | 同上方value同理(具体使用请参考@CacheConfig) | @Cacheable(cacheNames= "CREATE_NUMBER_RECORDING", key = "#infoDate",unless="#result == null") |
key | String | 缓存键,可使用“:”分割,分割后为组的概念,#后为方法上的参数名称,如果参数为对象,则#对象.get对应的属性值 | @Cacheable(value = "CREATE_NUMBER_RECORDING", key = "#infoDate+':'+#companyId+':'+#type+':'+#param1") |
keyGenerator | String | 自定义生成key方式,与key()不可以共用。其值为spring注入的类,其类实现implements KeyGenerator 接口 | @Cacheable(cacheNames = "ids_cache",keyGenerator = "setKeyGrenerator") |
cacheManager | String | -- | -- |
cacheResolver | String | -- | -- |
condition | String | 使用SpEL表达式设定出发缓存的条件,在方法执行前生效 | |
unless | String | 使用SpEL设置出发缓存的条件,方法执行完生效,所以条件中可以有方法执行后的value。函数返回值符合条件的排除掉、只缓存其余不符合条件的 | @Cacheable(unless="#result == null") 排除值为null 的情况 |
代码案例
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.zbintel.mom.factory.dao.NumberCreateDao;
import com.zbintel.mom.factory.entity.NumberCreateEntity;
import com.zbintel.mom.factory.service.NumberCreateCacheService;
import org.springframework.cache.annotation.CachePut;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service("numberCreateCacheService")
public class NumberCreateCacheServiceImpl extends ServiceImpl<NumberCreateDao, NumberCreateEntity> implements NumberCreateCacheService {
/**
* 设置其 value : CREATE_NUMBER_RECORDING (组的概念)
* key : infoDate:companyId:type (获取请求参数中的对应值, : 号分割后给其进行组分割)
* @return
*/
@CachePut(value = "CREATE_NUMBER_RECORDING", key = "#entity.getInfoDate()+':'+#entity.getCompanyId()+':'+#entity.getType()")
public NumberCreateEntity saveOrUpdateCaCheParamIsNull(NumberCreateEntity entity){
saveOrUpdate(entity);
return entity;
}
}
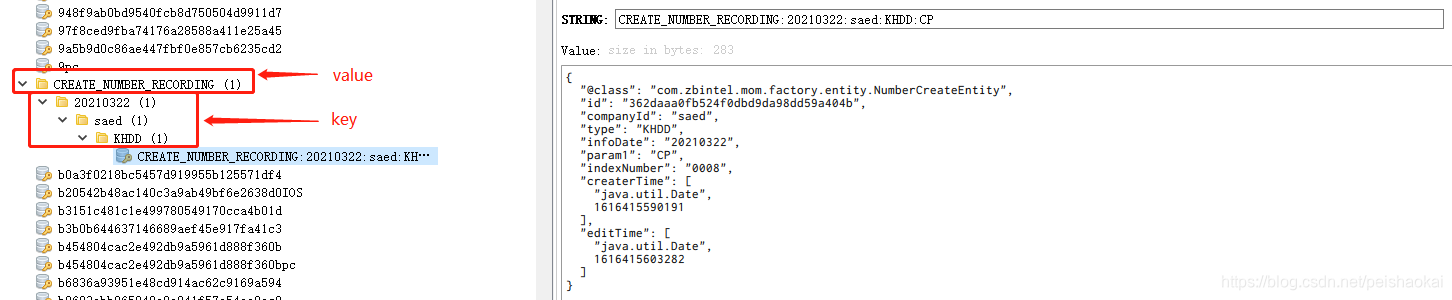
@CacheEvict
删除缓存数据,配置到方法上。用来清除用在本方法或者类上的缓存数据。
注解参数
参数 | 类型 | 解释 | 样例 |
---|---|---|---|
value | String[] | 缓存位置名称(可以认为其为组的概念) | @Cacheable(value = "CREATE_NUMBER_RECORDING", key = "#infoDate") |
cacheNames | String[] | 同上方value同理(具体使用请参考@CacheConfig) | @Cacheable(cacheNames= "CREATE_NUMBER_RECORDING", key = "#infoDate") |
key | String | 缓存键,可使用“:”分割,分割后为组的概念,#后为方法上的参数名称,如果参数为对象,则#对象.get对应的属性值 | @Cacheable(value = "CREATE_NUMBER_RECORDING", key = "#infoDate+':'+#companyId+':'+#type+':'+#param1") |
keyGenerator | String | 自定义生成key方式,与key()不可以共用。其值为spring注入的类,其类实现implements KeyGenerator 接口 | @Cacheable(cacheNames = "ids_cache",keyGenerator = "setKeyGrenerator") |
cacheManager | String | -- | -- |
cacheResolver | String | -- | -- |
condition | String | 使用SpEL表达式设定出发缓存的条件,在方法执行前生效 | |
allEntries | boolean | allEntries是boolean类型,表示是否需要清除缓存中的所有元素。默认为false,表示不需要。当指定了allEntries为true时,Spring Cache将忽略指定的key。有的时候我们需要Cache一下清除所有的元素,这比一个一个清除元素更有效率。 | |
beforeInvocation | boolean | 清除操作默认是在对应方法成功执行之后触发的,即方法如果因为抛出异常而未能成功返回时也不会触发清除操作。使用beforeInvocation可以改变触发清除操作的时间,当我们指定该属性值为true时,Spring会在调用该方法之前清除缓存中的指定元素。 |
代码案例
@CacheEvict(value="users", beforeInvocation=true)
public void delete(Integer id) {
System.out.println("delete user by id: " + id);
}
@Cacheing
同时指定多个Spring Cache相关的注解,配置到方法或者类上。其拥有三个属性:cacheable、put和evict,分别用于指定@Cacheable、@CachePut和@CacheEvict。
注解参数
参数 | 类型 | 解释 | 样例 |
---|---|---|---|
cacheable | Cacheable[] | 配置多个Cacheable | @Caching(cacheable = @Cacheable("users"), evict = { @CacheEvict("cache2"),@CacheEvict(value = "cache3", allEntries = true) }) |
put | CachePut[] | 配置多个CachePut | @Cacheable(cacheNames= "CREATE_NUMBER_RECORDING", key = "#infoDate",unless="#result == null") |
evict | CacheEvict[] | 配置多个CacheEvict | @Cacheable(value = "CREATE_NUMBER_RECORDING", key = "#infoDate+':'+#companyId+':'+#type+':'+#param1") |
代码案例
@Caching(cacheable = @Cacheable("users"), evict = { @CacheEvict("cache2"),
@CacheEvict(value = "cache3", allEntries = true) })
public User find(Integer id) {
return null;
}
@CacheConfig
公共配置。配置在类上。cacheNames即定义了本类中所有用到缓存的地方,都去找这个库。只要使用了这个注解,在方法上@Cacheable @CachePut @CacheEvict就可以不用写value去找具体库名了。
注解参数
参数 | 类型 | 解释 | 样例 |
---|---|---|---|
cacheNames | String[] | 同上方value同理(具体使用请参考@CacheConfig) | @Cacheable(cacheNames= "CREATE_NUMBER_RECORDING", key = "#infoDate",unless="#result == null") |
keyGenerator | String | 自定义生成key方式,与key()不可以共用。其值为spring注入的类,其类实现implements KeyGenerator 接口 | @Cacheable(cacheNames = "ids_cache",keyGenerator = "setKeyGrenerator") |
cacheManager | String | -- | -- |
cacheResolver | String | -- | -- |
使用自定义注解
Spring允许我们在配置可缓存的方法时使用自定义的注解,前提是自定义的注解上必须使用对应的注解进行标注。(使用场景:可以配置公共的注解, 进行分组统一管理)
创建自定义注解
@Target({ElementType.TYPE, ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
@Cacheable(value="selInfo")
public @interface SelCacheable {
}
使用
@SelCacheable
public Number findById(String id) {
Number n = new Number();
return n;
}
参考博客
Spring Boot缓存注解@Cacheable、@CacheEvict、@CachePut使用