#define _CRT_SECURE_NO_WARNINGS
#include"list.h"
#include<assert.h>
void SListInit(PNode* pHead)
{
assert(pHead);
*pHead = NULL;
}
PNode BuySListNode(DataType data)
{
PNode PNewNode = (PNode )malloc(sizeof(Node));
if (PNewNode == NULL)
{
return NULL;
}
PNewNode->_pNext = NULL;
PNewNode->_data = data;
return PNewNode;
}
void SListPushBack(PNode* pHead, DataType data)
{
assert(pHead);
if (NULL == pHead)
{
return;
}
else
{
PNode pCur = NULL;
pCur = *pHead;
while (pCur->_pNext)
{
pCur = pCur->_pNext;
}
pCur->_pNext = BuySListNode(data);
}
}
void SListPopBack(PNode* pHead)
{
assert(pHead);
if (NULL == *pHead)
return ;
else if (NULL == (*pHead)->_pNext)
{
PNode TmpNode = *pHead;
free(TmpNode);
TmpNode = NULL;
*pHead = NULL;
}
else
{
PNode pCur = *pHead;
while (pCur->_pNext->_pNext)
{
pCur = pCur->_pNext;
}
pCur->_pNext = NULL;
}
}
void SListPushFront(PNode* pHead, DataType data)
{
PNode PNewNode = NULL;
assert(pHead);
PNewNode = BuySListNode(data);
if (NULL == PNewNode)
return;
PNewNode->_pNext = *pHead;
*pHead = PNewNode;
}
void SListPopFront(PNode* pHead)
{
PNode pDelNode = NULL;
assert(pHead);
if (NULL == pHead)
return ;
pDelNode = *pHead;
*pHead = pDelNode->_pNext;
free(pDelNode);
}
PNode SListFind(PNode pHead, DataType data)
{
PNode pCur = pHead;
while (pCur)
{
if (pCur->_data == data)
{
return pCur;
}
pCur = pCur->_pNext;
}
return NULL;
}
void SListInsert(PNode* pHead, PNode pos, DataType data)
{
PNode PNewNode = NULL;
assert(pHead);
if (NULL == *pHead || NULL == pos)
return;
PNewNode = BuySListNode(data);
PNewNode->_pNext = pos->_pNext;
pos->_pNext = PNewNode;
}
void SListErase(PNode* pHead, PNode pos)
{
assert(pHead);
if (NULL == *pHead || NULL == pos)
return;
if (pos == *pHead)
{
SListPopFront(pHead);
}
else
{
PNode pCur = *pHead;
while (pCur && pCur->_pNext != pos)
{
pCur = pCur->_pNext;
}
if (pCur)
{
pCur->_pNext = pos->_pNext;
free(pos);
}
}
}
void SListClear(PNode* pHead)
{
PNode pDelNode = NULL;
assert(pHead);
while (*pHead)
{
pDelNode = *pHead;
*pHead = pDelNode->_pNext;
free(pDelNode);
}
}
void SListDestroy(PNode* pHead)
{
SListClear(pHead);
}
int SListSize(PNode pHead)
{
int count = 0;
PNode pCur = pHead;
assert(pHead);
if (NULL == pHead)
return 0;
pCur = pHead;
while (pCur)
{
count++;
pCur = pCur->_pNext;
}
return count;
}
PNode SListBack(PNode pHead)
{
PNode pCur = pHead;
assert(pHead);
while (pCur->_pNext)
{
pCur = pCur->_pNext;
}
return pCur;
}
void PrintList(PNode* pHead)
{
PNode pCur = *pHead;
while (pCur)
{
printf("%d->", pCur->_data);
pCur = pCur->_pNext;
}
printf("NULL\n");
}
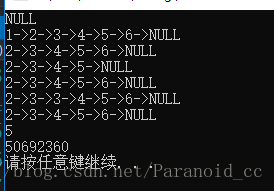
以下为链表面试题
void PrintListFromTail2Head(PNode pHead)
{
if (pHead)
{
PrintListFromTail2Head(pHead->_pNext);
printf("%d--", pHead->_data);
}
}
void DeleteNotTailNode(PNode pos)
{
PNode pDelNode = NULL;
if (NULL == pos&&NULL == pos->_pNext)
return;
pDelNode = pos->_pNext;
pos->_data = pDelNode->_data;
pos->_pNext = pDelNode->_pNext;
free(pDelNode);
}
void InsertPosFront(PNode pos, DataType data)
{
PNode pNewNode = NULL;
if (NULL == pos)
return;
pNewNode = BuySListNode(pos->_data);
pNewNode->_pNext = pos->_pNext;
pos->_pNext = pNewNode;
pos->_data = data;
}
void JosephCircle(PNode* pHead, const int M)
{
assert(pHead);
PNode pCur = *pHead;
if (NULL == pHead)
return;
while (pCur->_pNext != pCur)
{
int count = M;
while (--count)
{
pCur = pCur->_pNext;
}
PNode pDelNode = pCur->_pNext;
pCur->_data = pDelNode->_data;
pCur->_pNext = pDelNode->_pNext;
free(pDelNode);
}
*pHead = pCur;
}
void BubbleSort(PNode pHead)
{
PNode pPreCur = NULL;
PNode pCur = NULL;
PNode pTail = NULL;
if (NULL == pHead || NULL == pHead->_pNext)
return;
while (pHead != pTail)
{
int IsChange = 0;
pPreCur = pHead;
pCur = pPreCur->_pNext;
while (pCur != pTail)
{
if (pPreCur->_data > pCur->_data)
{
int tmp = pPreCur->_data;
pPreCur->_data = pCur->_data;
pCur->_data = tmp;
IsChange = 1;
}
pPreCur = pCur;
pCur = pPreCur->_pNext;
}
if (!IsChange)
return;
pTail = pPreCur;
}
}
void ReverseSList(PNode* pHead)
{
PNode pPre = NULL;
PNode pCur = NULL;
PNode pNext = NULL;
assert(pHead);
if (NULL == *pHead || NULL == (*pHead)->_pNext)
return;
pCur = *pHead;
while (pCur)
{
pNext = pCur->_pNext;
pCur->_pNext = pPre;
pPre = pCur;
pCur = pNext;
}
*pHead = pPre;
}
PNode ReverseSListOP(PNode pHead)
{
PNode pNewHead = NULL;
PNode pCur = pHead;
PNode pNext = NULL;
if (NULL == pHead || NULL == pHead->_pNext)
return pHead;
while (pCur)
{
pNext = pCur->_pNext;
pCur->_pNext = pNewHead;
pNewHead = pCur;
pCur = pNext;
}
return pNewHead;
}
PNode MergeSList(PNode pHead1, PNode pHead2)
{
PNode pNewHead = NULL;
PNode pTailNode = NULL;
PNode pL1 = pHead1;
PNode pL2 = pHead2;
if (NULL == pHead1)
return pHead2;
if (NULL == pHead2)
return pHead1;
if (pL1->_data < pL2->_data)
{
pNewHead = pL1;
pL1 = pL1->_pNext;
}
else
{
pNewHead = pL2;
pL2 = pL2->_pNext;
}
pTailNode = pNewHead;
while (pL1 && pL2)
{
if (pL1->_data < pL2->_data)
{
pTailNode->_pNext = pL1;
pL1 = pL1->_pNext;
}
else
{
pTailNode->_pNext = pL2;
pL2 = pL2->_pNext;
}
pTailNode = pTailNode->_pNext;
}
if (pL1)
pTailNode->_pNext = pL1;
else
pTailNode->_pNext = pL2;
return pNewHead;
}
PNode FindMiddleNode(PNode pHead)
{
PNode pFast = pHead;
PNode pSlow = pHead;
while (pFast && pFast->_pNext)
{
pFast = pFast->_pNext->_pNext;
pSlow = pSlow->_pNext;
}
return pSlow;
}
PNode FindLastKNode(PNode pHead, int K)
{
PNode pFast = pHead;
PNode pSlow = pHead;
if (NULL == pHead || K < 0)
return NULL;
while (K--)
{
if (NULL == pFast)
return NULL;
pFast = pFast->_pNext;
}
while (pFast)
{
pFast = pFast->_pNext;
pSlow = pSlow->_pNext;
}
return pSlow;
}
PNode DeleteLastKNode(PNode pHead, int K)
{
PNode pFast = pHead;
PNode pSlow = pHead;
PNode pPre = NULL;
if (NULL == pHead || K <= 0)
return NULL;
int count = K;
while (count--)
{
if (NULL == pFast)
return NULL;
pFast = pFast->_pNext;
}
while (pFast)
{
pPre = pSlow;
pFast = pFast->_pNext;
pSlow = pSlow->_pNext;
}
pPre->_pNext = pSlow->_pNext;
free(pSlow);
return pHead;
}
int IsCrossWithoutCircle(PNode pHead1, PNode pHead2)
{
PNode pTail1 = pHead1;
PNode pTail2 = pHead2;
if (NULL == pHead1 || NULL == pHead2)
return 0;
while (pTail1)
pTail1 = pTail1->_pNext;
while (pTail2)
pTail2 = pTail2->_pNext;
return pTail1 == pTail2;
}
PNode GetCrossNode(PNode pHead1, PNode pHead2)
{
int size1 = 0, size2 = 0, gap;
PNode pCur1 = pHead1, pCur2 = pHead2;
if (!(IsCrossWithoutCircle(pHead1, pHead2)))
return NULL;
while (pCur1)
{
size1++;
pCur1 = pCur1->_pNext;
}
while (pCur2)
{
size2++;
pCur2 = pCur2->_pNext;
}
gap = size1 - size2;
pCur1 = pHead1;
pCur2 = pHead2;
if (gap > 0)
{
while (gap--)
pCur1 = pCur1->_pNext;
}
else
{
while (gap++)
pCur2 = pCur2->_pNext;
}
while (pCur1 != pCur2)
{
pCur1 = pCur1->_pNext;
pCur2 = pCur2->_pNext;
}
return pCur1;
}
PNode IsCircle(PNode pHead)
{
PNode pFast = pHead;
PNode pSlow = pHead;
while (pFast&&pFast->_pNext)
{
pFast = pFast->_pNext->_pNext;
pSlow = pSlow->_pNext;
if (pFast == pSlow)
return pFast;
}
return 0;
}
int GetCircleLen(PNode pHead)
{
PNode pMeetNode = IsCircle(pHead);
PNode pCur = pMeetNode;
int count = 1;
if (NULL == pMeetNode)
return 0;
while (pCur->_pNext != pMeetNode)
{
count++;
pCur = pCur->_pNext;
}
return count;
}
PNode GetEnterNode(PNode pHead, PNode pMeetNode)
{
PNode PH = pHead;
PNode PM = pMeetNode;
if (NULL == pHead || NULL == pMeetNode)
return NULL;
while (PH != PM)
{
PH = PH->_pNext;
PM = PM->_pNext;
}
return PM;
}
int IsListCrossWithCircle(PNode pHead1, PNode pHead2)
{
PNode pMeetNode1 = NULL;
PNode pMeetNode2 = NULL;
if (NULL == pHead1 || NULL == pHead2)
return 0;
pMeetNode1 = IsCircle(pHead1);
pMeetNode2 = IsCircle(pHead2);
if (NULL == pMeetNode1&&NULL == pMeetNode2)
{
PNode pTail1 = pHead1;
PNode pTail2 = pHead2;
while (pTail1->_pNext)
pTail1 = pTail1->_pNext;
while (pTail2->_pNext)
pTail2 = pTail2->_pNext;
if (pTail1 == pTail2)
return 1;
}
else if (pMeetNode1 && pMeetNode2)
{
PNode pCur = pMeetNode1;
while (pCur->_pNext != pMeetNode1)
{
if (pCur == pMeetNode2)
return 2;
pCur = pCur->_pNext;
}
if (pCur == pMeetNode2)
return 2;
}
return 0;
}
PCListNode CopyComplexList(PCListNode pHead)
{
PCListNode pOldNode = pHead;
PCListNode pNewNode = NULL;
PCListNode pNewHead = NULL;
if (NULL == pHead)
return NULL;
while (pOldNode)
{
pNewHead = (PCListNode)malloc(sizeof(CListNode));
pNewHead->_data = pOldNode->_data;
pNewNode->_pNext = NULL;
pNewNode->_pRandom = NULL;
if (NULL == pNewNode)
return NULL;
pNewNode->_pNext = pOldNode->_pNext;
pOldNode->_pNext = pNewNode;
pOldNode = pNewNode->_pNext;
}
pOldNode = pHead;
while (pOldNode)
{
pNewNode = pOldNode->_pNext;
if (NULL == pOldNode->_pRandom)
pNewNode->_pRandom = NULL;
else
pNewNode->_pRandom = pOldNode->_pRandom->_pNext;
pOldNode = pNewNode->_pNext;
}
pOldNode = pHead;
pNewHead = pOldNode->_pNext;
while (pOldNode->_pNext)
{
pNewNode = pOldNode->_pNext;
pOldNode->_pNext = pNewNode->_pNext;
pOldNode = pNewNode;
}
return pNewHead;
}