链表实现FIFO功能;
push:结点压入链表
pop:弹出最后一个被压入的结点
printnode:打印整个链表
freenode:释放制定的结点占据的内存
freelist:释放整个链表
getlen:获取链表长度
reverse:链表翻转
find:寻找指定的结点,没有返回NULL,有多个返回查找到的第一个结点
insert:在指定位置插入一个结点
PS:在链表的翻转以及排序问题上,利用find指定位置的结点,直接交换结点内的内容可以简化操作。
/*
singly linked list;
*/
#include<stdio.h>
#include<stdlib.h>
typedef struct listnode
{
struct listnode* next;
int data;
}Node;
int push(Node**head,int data);
int pop(Node**head);
void Printnode(Node*head);
void freenode(Node*node);
void freelist(Node** head);
int getlen(Node*head);
void reverse(Node**head);
void find(Node* head,int data,Node**node);
int insert(Node*head,int data,int position);
void printnode(Node*head)
{
if(head!=NULL)
{
int nodeval=0;
do{
printf("node %d ,value is %d\n",nodeval,head->data);
nodeval++;
head=head->next;
}while(head!=NULL);
}
else
{
printf("list is null\n");
}
}
void reverse(Node**head)
{
if(getlen(*head)==0||getlen(*head)==1)
{
return ;
}
Node*newhead=NULL;
Node*temphead=*head;
do{
if(newhead==NULL)
{
newhead=temphead;
temphead=temphead->next;
newhead->next=NULL;
}
else
{
Node* n=temphead;
temphead=temphead->next;
n->next=newhead;
newhead=n;
}
}while(temphead!=NULL);
*head=newhead;
}
int getlen(Node*head)
{
int ret=0;
if(head==NULL)
{
return ret;
}
do{
ret++;
head=head->next;
}while(head!=NULL);
return ret;
}
void find(Node*head,int data,Node**node)
{
Node*target=NULL;
int count=getlen(head);
int i;
for(i=0;i<count;i++)
{
if(head->data!=data)
{
head=head->next;
}
else
{
target=head;
}
}
*node=target;
}
int insert(Node *head,int data,int position)
{
if(getlen(head)<position || position<0)
{
printf("position is not avaliable\n");
return 0;
}
int num=0;
while(1)
{
if(num!=position)
{
head=(head)->next;
num++;
}
else
{
Node*node=(Node*)malloc(sizeof(node));
node->data=data;
if(head->next==NULL)
{
node->next=NULL;
head->next=node;
}
else
{
node->next=head->next;
head->next=node;
}
return 1;
}
}
}
void freelist(Node**head)
{
if(*head!=NULL)
{
do{
Node*node=*head;
*head=(*head)->next;
freenode(node);
}while(*head!=NULL);
}
}
int push(Node**head,int data)
{
Node *node=(Node*)malloc(sizeof(node));
if(node==NULL)
{
return 0;
}
node->data=data;
if(*head ==NULL)
{
node->next=NULL;
*head=node;
return 1;
}
else
{
node->next=(*head);
*head=node;
return 1;
}
}
int pop(Node**head)
{
if(*head==NULL)
{
return 0;
}
Node*node=*head;
(*head)=(*head)->next;
freenode(node);
return 1;
}
void freenode(Node*node)
{
if(node!=NULL)
{
free(node);
}
}
void main()
{
Node* head=NULL;
push(&head,5);
push(&head,4);
push(&head,3);
push(&head,2);
push(&head,1);
printf("list length is %d\n",getlen(head));
printf("show list:\n");
printf("-------------------\n");
printnode(head);
printf("-------------------\n");
printf("\n");
insert(head,99,4);
printf("insert one element,new list is:\n");
printf("-------------------\n");
printnode(head);
printf("-------------------\n");
printf("\n");
reverse(&head);
printf("show reversed list:\n");
printf("-------------------\n");
printnode(head);
printf("-------------------\n");
printf("\n");
pop(&head);
printf("one element poped,new list is:\n");
printf("-------------------\n");
printnode(head);
printf("-------------------\n");
printf("\n");
printf("find node with data=3...\n");
Node*t=NULL;
find(head,3,&t);
if(t==NULL)
{
printf("no element with data=3 found\n");
}
else
{
printf("element found,data is %d\n",t->data);
}
freelist(&head);
}
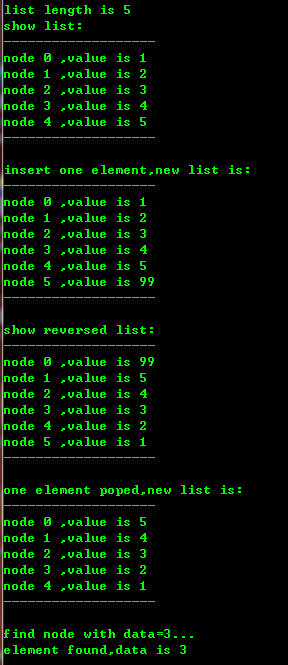