Java CompletableFuture 解析
CompletableFuture 是 Java 8 引入的 异步编程工具,允许我们在不阻塞主线程的情况下执行任务,并支持链式操作、异常处理和组合多个异步任务。
1. 创建 CompletableFuture
(1) 直接创建一个异步任务
java
CompletableFuture<String> future = CompletableFuture.supplyAsync(() > {
return "Hello, CompletableFuture!";
});
System.out.println(future.get()); // Hello, CompletableFuture!
supplyAsync(() > {...}) 适用于有返回值的异步任务。
get() 是 阻塞操作,会等待结果返回。
2. thenApply / thenAccept / thenRun
这三个方法用于处理 CompletableFuture 的结果:
thenApply(Function<T, R>):对结果 转换 并返回新 CompletableFuture。
thenAccept(Consumer<T>):对结果 消费,但不返回值。
thenRun(Runnable):无参数执行任务,不关心结果。
java
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() > 10)
.thenApply(result > result 2) // 20
.thenApply(result > result + 5); // 25
System.out.println(future.get()); // 25
java
CompletableFuture.supplyAsync(() > "Hello")
.thenAccept(result > System.out.println("Result: " + result)); // Result: Hello
java
CompletableFuture.supplyAsync(() > "Task done!")
.thenRun(() > System.out.println("No input, just run!")); // No input, just run!
3. 组合多个 CompletableFuture
(1) thenCombine 组合两个 CompletableFuture 结果
java
CompletableFuture<Integer> future1 = CompletableFuture.supplyAsync(() > 10);
CompletableFuture<Integer> future2 = CompletableFuture.supplyAsync(() > 20);
CompletableFuture<Integer> result = future1.thenCombine(future2, (a, b) > a + b);
System.out.println(result.get()); // 30
(2) allOf 等待所有 CompletableFuture 完成
java
CompletableFuture<Integer> f1 = CompletableFuture.supplyAsync(() > 10);
CompletableFuture<Integer> f2 = CompletableFuture.supplyAsync(() > 20);
CompletableFuture<Integer> f3 = CompletableFuture.supplyAsync(() > 30);
CompletableFuture<Void> allFutures = CompletableFuture.allOf(f1, f2, f3);
allFutures.join(); // 等待所有任务完成
int sum = f1.get() + f2.get() + f3.get();
System.out.println(sum); // 60
(3) anyOf 任意一个完成即返回
java
CompletableFuture<String> f1 = CompletableFuture.supplyAsync(() > {
try { Thread.sleep(1000); } catch (InterruptedException e) {}
return "Task 1";
});
CompletableFuture<String> f2 = CompletableFuture.supplyAsync(() > {
try { Thread.sleep(500); } catch (InterruptedException e) {}
return "Task 2";
});
CompletableFuture<Object> result = CompletableFuture.anyOf(f1, f2);
System.out.println(result.get()); // Task 2(因为 f2 先完成)
4. 处理异常
(1) exceptionally 发生异常时提供默认值
java
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() > {
if (true) throw new RuntimeException("Something went wrong!");
return 10;
}).exceptionally(ex > {
System.out.println("Error: " + ex.getMessage());
return 0;
});
System.out.println(future.get()); // Error: Something went wrong! > 0
(2) handle 处理正常 & 异常情况
java
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() > {
if (true) throw new RuntimeException("Error!");
return 10;
}).handle((result, ex) > {
if (ex != null) {
System.out.println("Handled exception: " + ex.getMessage());
return 1;
}
return result;
});
System.out.println(future.get()); // Handled exception: Error! > 1
5. thenCompose vs thenApply
thenApply 返回普通值
java
CompletableFuture<CompletableFuture<Integer>> nestedFuture = CompletableFuture.supplyAsync(() > 10)
.thenApply(value > CompletableFuture.supplyAsync(() > value 2));
System.out.println(nestedFuture.get().get()); // 20
thenCompose 返回扁平化 CompletableFuture
java
CompletableFuture<Integer> flatFuture = CompletableFuture.supplyAsync(() > 10)
.thenCompose(value > CompletableFuture.supplyAsync(() > value 2));
System.out.println(flatFuture.get()); // 20
✅ thenCompose 适用于异步任务嵌套时消除 CompletableFuture<CompletableFuture<T>>
6. 线程池控制
默认情况下,CompletableFuture 使用 ForkJoinPool 线程池。可以传入自定义线程池:
java
ExecutorService executor = Executors.newFixedThreadPool(3);
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() > {
System.out.println("Running in thread: " + Thread.currentThread().getName());
return 10;
}, executor);
✅ 适用于高并发任务,避免线程过载。
应用场景
✅ 高并发任务:数据查询、日志处理、批量任务
✅ API 并行调用:如多个远程 HTTP 请求并行执行
✅ I/O 操作:文件读写、数据库查询、网络请求
✅ 避免阻塞:不阻塞主线程,提高响应速度
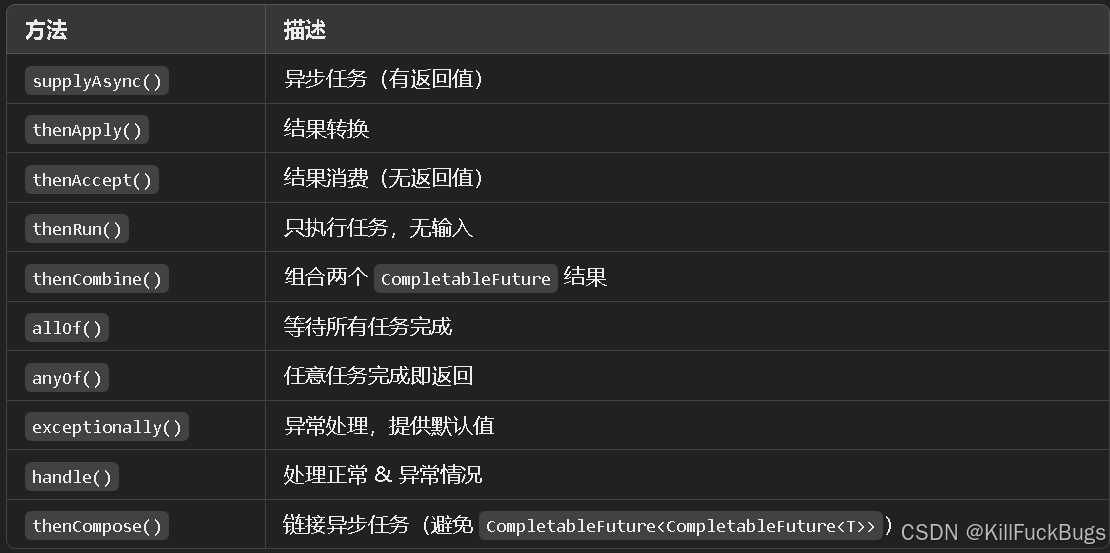