1 首先给出错误的程序 great.c
#include <Python.h>
int great_function(int a) {
return a + 1;
}
static PyObject * _great_function(PyObject *self, PyObject *args)
{
int _a;
int res;
if (!PyArg_ParseTuple(args, "i", &_a))
return NULL;
res = great_function(_a);
return PyLong_FromLong(res);
}
static PyMethodDef GreateModuleMethods[] = {
{
"great_function",
_great_function,
METH_VARARGS,
""
},
{NULL, NULL, 0, NULL}
};
PyMODINIT_FUNC initgreat_module(void) {
(void) Py_InitModule("great_module", GreateModuleMethods);
}
2 编译
Linux终端输入命令:
gcc -fPIC -shared great.c -I/usr/include/python3.10 -o great.so
注意:
编译时,先查看安装python的版本是多少,然后根据python版本号修改编译命令

如安装的python是3.xx.yy,那么编译命令
gcc -fPIC -shared great.c -I/usr/include/python3.xx -o great.so
编译报错
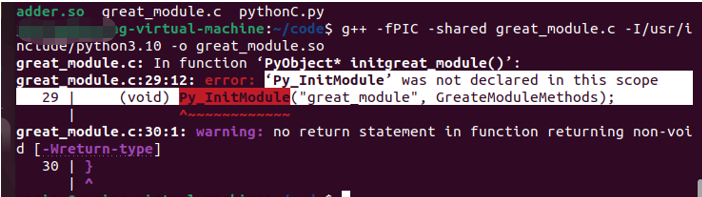
原因
在python3中,Py_InitModule模块已被淘汰
解决
修改great.c文件的部分:创建一个PyModuleDef结构,然后将其引用传递给PyModule_Create。
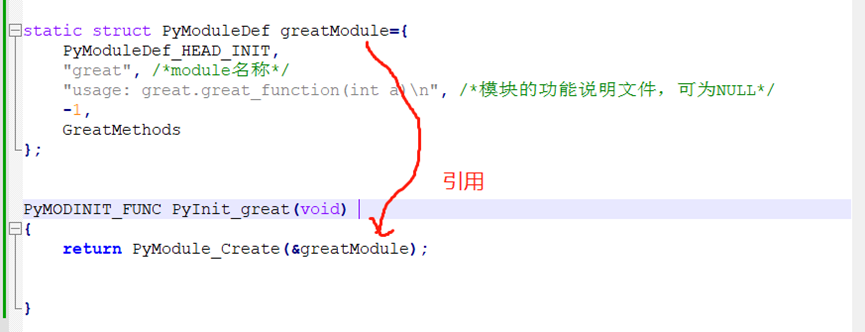
再次编译并运行
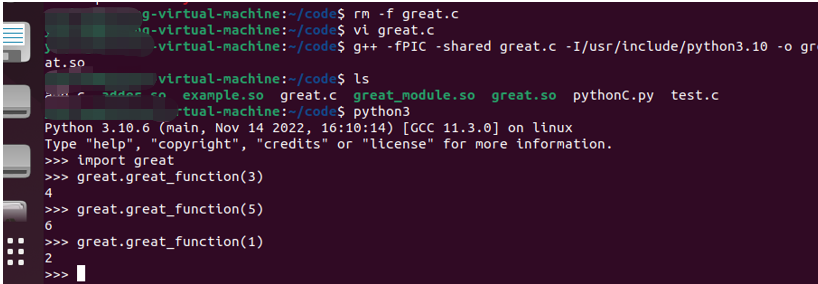
3完整程序
#include <Python.h>
int great_function(int a) {
return a + 1;
}
static PyObject * _great_function(PyObject *self, PyObject *args)
{
int _a;
int res;
if (!PyArg_ParseTuple(args, "i", &_a))
return NULL;
res = great_function(_a);
return PyLong_FromLong(res);
}
static PyMethodDef GreatMethods[] = {
{
"great_function",
_great_function,
METH_VARARGS,
""
},
{NULL, NULL, 0, NULL}
};
static struct PyModuleDef greatModule={
PyModuleDef_HEAD_INIT,
"great", /*module名称*/
"usage: great.great_function(int a)\n", /*模块的功能说明文件,可为NULL*/
-1,
GreatMethods
};
PyMODINIT_FUNC PyInit_great(void)
{
return PyModule_Create(&greatModule);
}
great.c文件参考:Python与C/C++混合编程 - 知乎 (zhihu.com)