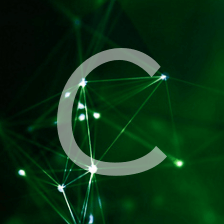
剑指offer
之井
路漫漫其修远兮
展开
-
【2022初春】【LeetCode】876. 链表的中间结点
/** * Definition for singly-linked list. * public class ListNode { * int val; * ListNode next; * ListNode() {} * ListNode(int val) { this.val = val; } * ListNode(int val, ListNode next) { this.val = val; this.next = next; } * }原创 2022-02-28 10:29:06 · 125 阅读 · 0 评论 -
【2022初春】【剑指offer】60. n个骰子的点数
前面讲的都很复杂,其实就是每摇一个骰子就更新一下概率,乘1/6class Solution { public double[] dicesProbability(int n) { double[] res = new double[6]; Arrays.fill(res,1.0/6.0); for(int i=2; i<=n; i++){ double[] tmp = new double[5*i+1];原创 2022-01-22 12:29:51 · 159 阅读 · 0 评论 -
【2022初春】【剑指offer】51. 数组中的逆序对
归并的思路是看懂了,但是写起来还是很迷茫class Solution { int[] nums,tmp; public int reversePairs(int[] nums) { this.nums = nums; tmp = new int[nums.length]; return manage(0,nums.length-1); } public int manage(int l,int r){ if(l原创 2022-01-22 10:41:55 · 354 阅读 · 0 评论 -
【2022初春】【剑指offer】44. 数字序列中某一位的数字
思路是对的,但是找count的时候,和n-1的细节处理要注意class Solution { public int findNthDigit(int n) { int digit=1; long count=9; long start=1; while(n>count){ n-=count; start*=10; digit++; c原创 2022-01-21 15:33:46 · 275 阅读 · 0 评论 -
【2021秋冬】【剑指offer】43. 1~n 整数中 1 出现的次数
这题看着简单,但是这个算每一位1个数的公式还是没有很推明白再慢慢想想吧class Solution { public int countDigitOne(int n) { int digit = 1, res = 0; int high = n/10, cur = n%10, low = 0; while(high !=0 || cur !=0){ if(cur == 0){ res += h原创 2022-01-19 22:58:48 · 170 阅读 · 0 评论 -
【2021秋冬】【剑指offer】 49. 丑数
丑数,算是看明白递归了,只是之前没想好怎么递归class Solution { public int nthUglyNumber(int n) { int a=1,b=1,c=1; int[] dp = new int[n+1]; dp[1]=1; for(int i=2; i<=n; i++){ dp[i] = Math.min(Math.min(dp[a]*2,dp[b]*3),dp[c]*5);原创 2022-01-19 22:56:32 · 406 阅读 · 0 评论 -
【2021秋冬】【剑指offer】38. 字符串的排列
递归回溯还没太想明白为什么用交换函数就可以实现排列class Solution { List<String> res = new LinkedList<>(); char[] c; public String[] permutation(String s) { c = s.toCharArray(); dfs(0); return res.toArray(new String[res.size()]);原创 2022-01-17 12:04:21 · 137 阅读 · 0 评论 -
【2021秋冬】【剑指offer】19. 正则表达式匹配
递归写法class Solution { public boolean isMatch(String s, String p) { if(s.length() == 0){ if(p.length()%2 !=0 ) return false; for(int i=1; i<p.length(); i+=2){ if(p.charAt(i) != '*') return false;原创 2022-01-17 11:14:12 · 149 阅读 · 0 评论 -
【2021秋冬】【剑指offer】37. 序列化二叉树
思路是对的,用层序遍历解决但是层序遍历反推二叉树需要再熟悉一下/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode(int x) { val = x; } * } */public class Codec { // Encodes a tree to a原创 2022-01-13 11:12:43 · 88 阅读 · 0 评论 -
【2021秋冬】【剑指offer】59 - II. 队列的最大值
大概的思路是对的,但是没有找到合适的数据结构注意判断弹出值是否相等的时候不能用==,要用equals,但是没想明白为什么class MaxQueue { Queue<Integer> q; Deque<Integer> dq; public MaxQueue() { q = new LinkedList<>(); dq = new LinkedList<>(); } publ原创 2022-01-12 11:49:02 · 86 阅读 · 0 评论 -
【2021秋冬】【剑指offer】59 - I. 滑动窗口的最大值
单调队列,滑动窗口思路还是要再缕清楚一些class Solution { public int[] maxSlidingWindow(int[] nums, int k) { if(nums.length==0||k==0) return new int[0]; int len = nums.length; Deque<Integer> q = new LinkedList<>(); int[] res =原创 2022-01-12 11:18:27 · 68 阅读 · 0 评论 -
【2021秋冬】【剑指offer】67. 把字符串转换成整数
java的int大小:-2147483648——2147483647这道题主要的难点是怎么判断出界class Solution { public int strToInt(String str) { int len = str.length(); int index = 0; int hasflag = 1; int res = 0; int bndry = Integer.MAX_VALUE / 10;原创 2022-01-11 21:02:53 · 128 阅读 · 0 评论 -
【2021秋冬】【剑指offer】20. 表示数值的字符串
字符串的题就是很麻烦class Solution { public boolean isNumber(String s) { int len = s.length(); int index = 0; int hasE = 0, hasnum = 0, hasflag = 0, hasdot = 0; while(index<len&&s.charAt(index)==' ') index++; w原创 2022-01-11 20:38:29 · 68 阅读 · 0 评论 -
【2021秋冬】【剑指offer】31. 栈的压入、弹出序列
用一个辅助栈来解决问题不要想的太复杂class Solution { public boolean validateStackSequences(int[] pushed, int[] popped) { Stack<Integer> res = new Stack<>(); int i=0; for(int num : pushed){ res.push(num); whil原创 2022-01-11 19:59:07 · 204 阅读 · 0 评论 -
【2021秋冬】【剑指offer】29. 顺时针打印矩阵
所谓的模拟就是,按想法写出来?注意定义的上下左右不是长度,是边界class Solution { public int[] spiralOrder(int[][] matrix) { if(matrix == null || matrix.length == 0) return new int[0]; int row = matrix.length, col = matrix[0].length; int[] res = new in原创 2022-01-11 17:27:23 · 221 阅读 · 0 评论 -
【2021秋冬】【剑指offer】62. 圆圈中最后剩下的数字
约瑟夫环问题反正是数学规律回推class Solution { public int lastRemaining(int n, int m) { int ans = 0; for(int i=2; i<=n; i++){ ans = (ans+m)%i; } return ans; }}原创 2022-01-11 16:52:22 · 75 阅读 · 0 评论 -
【2021秋冬】【剑指offer】57 - II. 和为s的连续正数序列
滑动窗口写法只能向右走,注意sum加减的顺序class Solution { public int[][] findContinuousSequence(int target) { List<int[]> res = new ArrayList<>(); int i=1, j=2, sum=3; while(i<j){ if(sum < target){ j原创 2022-01-11 16:02:06 · 66 阅读 · 0 评论 -
【2021秋冬】【剑指offer】14- I. 剪绳子
只看懂了贪心的写法动规的没太看明白class Solution { public int cuttingRope(int n) { if(n < 4) return n-1; int res = 1; while(n>4){ res *= 3; n -= 3; } return res*n; }}...原创 2022-01-10 20:25:23 · 254 阅读 · 0 评论 -
【2021秋冬】【剑指offer】66. 构建乘积数组
构建上下两个三角数组分别循环乘注意下标的问题class Solution { public int[] constructArr(int[] a) { int len = a.length; int[] b = new int[len]; if(len == 0) return b; b[0] = 1; for(int i=1; i<len; i++){ b[i] = b[i-原创 2022-01-10 20:08:48 · 216 阅读 · 0 评论 -
【2021秋冬】【剑指offer】56 - I. 数组中数字出现的次数
位操作还有很多地方需要细想class Solution { public int[] singleNumbers(int[] nums) { int n = 0; for(int num : nums){ n ^= num; } int m = 1; while((n&m)==0) m <<= 1; int x=0,y=0;原创 2022-01-06 15:12:43 · 187 阅读 · 0 评论 -
【2021秋冬】【剑指offer】56 - II. 数组中数字出现的次数 II
统计尾数,不能整除三的说明此位为1更新结果时也要左移 用或 |=class Solution { public int singleNumber(int[] nums) { int res = 0; for(int i=0; i<32; i++){ int cun = 0; for(int j=0; j<nums.length; j++){ cun += (nums[j]&原创 2022-01-06 11:31:40 · 249 阅读 · 0 评论 -
【2021秋冬】【剑指offer】65. 不用加减乘除做加法
用异或和与左移,实现加法class Solution { public int add(int a, int b) { while(b != 0){ int c = (a&b)<<1; a ^= b; b = c; } return a; }}这样写,结果好像是对的,但是稍微大一点就超时了,,还不是很理解class Solutio原创 2022-01-06 10:25:07 · 254 阅读 · 0 评论 -
【2021秋冬】【剑指offer】15. 二进制中1的个数
& 相同为1,不同为0,注意判断条件不能用>0,因为java存在负号的可能性,无符号位移用>>>public class Solution { // you need to treat n as an unsigned value public int hammingWeight(int n) { int res = 0; while(n!=0){ if((n&原创 2022-01-06 10:03:36 · 59 阅读 · 0 评论 -
【2021秋冬】【剑指offer】33. 二叉搜索树的后序遍历序列
二叉搜索树,后序遍历写树,就左右判断class Solution { public boolean verifyPostorder(int[] postorder) { return recur(postorder,0,postorder.length-1); } boolean recur(int[] postorder, int i, int j){ if(i>=j) return true; int p = i;原创 2022-01-05 11:27:15 · 1089 阅读 · 0 评论 -
【2021秋冬】【剑指offer】68 - II. 二叉树的最近公共祖先
自己想不出来只能说学习一下寻找最近公共祖先的写法了/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode(int x) { val = x; } * } */class Solution { public TreeNode lowestCommonAnces原创 2022-01-04 20:25:59 · 242 阅读 · 0 评论 -
【2021秋冬】【剑指offer】68 - I. 二叉搜索树的最近公共祖先
二叉搜索树自己学会了思路,但是写的时候出错,因为递归时需要加return/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode(int x) { val = x; } * } */class Solution { public TreeNode lowest原创 2022-01-04 19:58:16 · 151 阅读 · 0 评论 -
【2021秋冬】【剑指offer】64. 求 1 + 2 + … + n
不能用乘除法,和循环之类的,所以公式求和不行class Solution { public int sumNums(int n) { return (1+n)*n/2; }}递归需要判断,用&& || 的短路法class Solution { int res = 0; public int sumNums(int n) { boolean x = n > 1 && sumNums(n-1)>原创 2022-01-04 17:21:37 · 73 阅读 · 0 评论 -
【2021秋冬】【剑指offer】16. 数值的整数次方
快速幂;看下来其实就是幂运算拆成位运算的方法精简class Solution { public double myPow(double x, int n) { if(x==0) return 0; long b = n; if(b < 0){ x = 1/x; b = -b; } double res = 1.0; while(b>0){原创 2022-01-04 17:01:39 · 140 阅读 · 0 评论 -
【2021秋冬】【剑指offer】55 - II. 平衡二叉树
时间复杂度比较高的一种写法,但是思路能想到,就是一层一层判断当前节点是否平衡。题解称之为先序遍历/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode(int x) { val = x; } * } */class Solution { public bool原创 2022-01-04 16:08:01 · 246 阅读 · 0 评论 -
【2021秋冬】【剑指offer】55 - I. 二叉树的深度
递归参数的理解还是不到位/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode(int x) { val = x; } * } */class Solution { public int maxDepth(TreeNode root) { if原创 2022-01-04 15:43:12 · 99 阅读 · 0 评论 -
【2021秋冬】【剑指offer】41. 数据流中的中位数
主要思路是会用大顶堆和小顶堆,因为题意是保持排序,取中间值,刚好用两个堆来维持中间值。注意堆的更新class MedianFinder { /** initialize your data structure here. */ Queue<Integer> minQ,maxQ; public MedianFinder() { minQ = new PriorityQueue<>(); maxQ = new PriorityQ原创 2022-01-04 15:35:46 · 137 阅读 · 0 评论 -
【2021秋冬】【剑指offer】61. 扑克牌中的顺子
一开始没看明白题意,其实就是找除了0意外的数字,相差不大于5,等于五也不行还有一个排序的方法也可class Solution { public boolean isStraight(int[] nums) { int minn = 0,maxn = 0; Set<Integer> set = new HashSet<>(); for(int i=0; i<nums.length; i++){ i原创 2022-01-04 14:56:48 · 321 阅读 · 0 评论 -
【2021秋冬】【剑指offer】45. 把数组排成最小的数
可以用冒泡,也可以用快排,改写内置函数class Solution { public String minNumber(int[] nums) { String strs[] = new String[nums.length]; for(int i=0; i<nums.length; i++) strs[i] = String.valueOf(nums[i]); Arrays.sort(strs,(x,y)->(x原创 2022-01-03 23:02:38 · 229 阅读 · 0 评论 -
【2021秋冬】【剑指offer】54. 二叉搜索树的第k大节点
思路还是差不多对的,但是其他参数的控制需要考虑清楚/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode(int x) { val = x; } * } */class Solution { int res,index = 0; public int原创 2021-12-31 16:43:46 · 267 阅读 · 0 评论 -
【2021秋冬】【剑指offer】36. 二叉搜索树与双向链表
能想到是中序遍历,但是链表的构造没有想到题解是类似指针标记/*// Definition for a Node.class Node { public int val; public Node left; public Node right; public Node() {} public Node(int _val) { val = _val; } public Node(int _val,Node _left,Node原创 2021-12-31 14:27:28 · 537 阅读 · 0 评论 -
【2021秋冬】【剑指offer】34. 二叉树中和为某一值的路径
思路是对的,但是路径在递归里的循环和存储需要注意/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode() {} * TreeNode(int val) { this.val = val; } * TreeNode(int val, TreeNode l原创 2021-12-31 10:49:49 · 194 阅读 · 0 评论 -
【2021秋冬】【剑指offer】39. 数组中出现次数超过一半的数字
HashMap方法class Solution { public int majorityElement(int[] nums) { int len = nums.length; Map<Integer,Integer> map = new HashMap<>(); for(int i=0; i<len; i++){ map.put(nums[i],map.getOrDefault(nums[i]原创 2021-12-30 17:00:06 · 125 阅读 · 0 评论 -
【2021秋冬】【剑指offer】13. 机器人的运动范围
整体思路倒是大差不差,注意标记和细节class Solution { int res = 0; public int movingCount(int m, int n, int k) { boolean[][] visited = new boolean[m][n]; dfs(m,n,visited,k,0,0); return res; } void dfs(int m, int n,boolean[][] visited原创 2021-12-30 15:45:19 · 138 阅读 · 0 评论 -
【2021秋冬】【剑指offer】57. 和为 s 的两个数字
自己思路的双指针指的不对,超时了,要前后相撞指针,排除法class Solution { public int[] twoSum(int[] nums, int target) { int[] res = new int[2]; if(nums.length == 0 || target <= nums[0]) return null; int i=0,j=nums.length - 1; while(i<j){原创 2021-12-29 15:12:24 · 333 阅读 · 0 评论 -
【2021秋冬】【剑指offer】21. 调整数组顺序使奇数位于偶数前面
双指针,思路是对的,判断条件要注意class Solution { public int[] exchange(int[] nums) { //int[] res = new int[nums.length]; int i = 0, j = nums.length - 1; while(i<j){ while(i<j&&nums[i]%2!=0) i++; while(i&l原创 2021-12-29 10:27:37 · 75 阅读 · 0 评论