六、普利姆算法
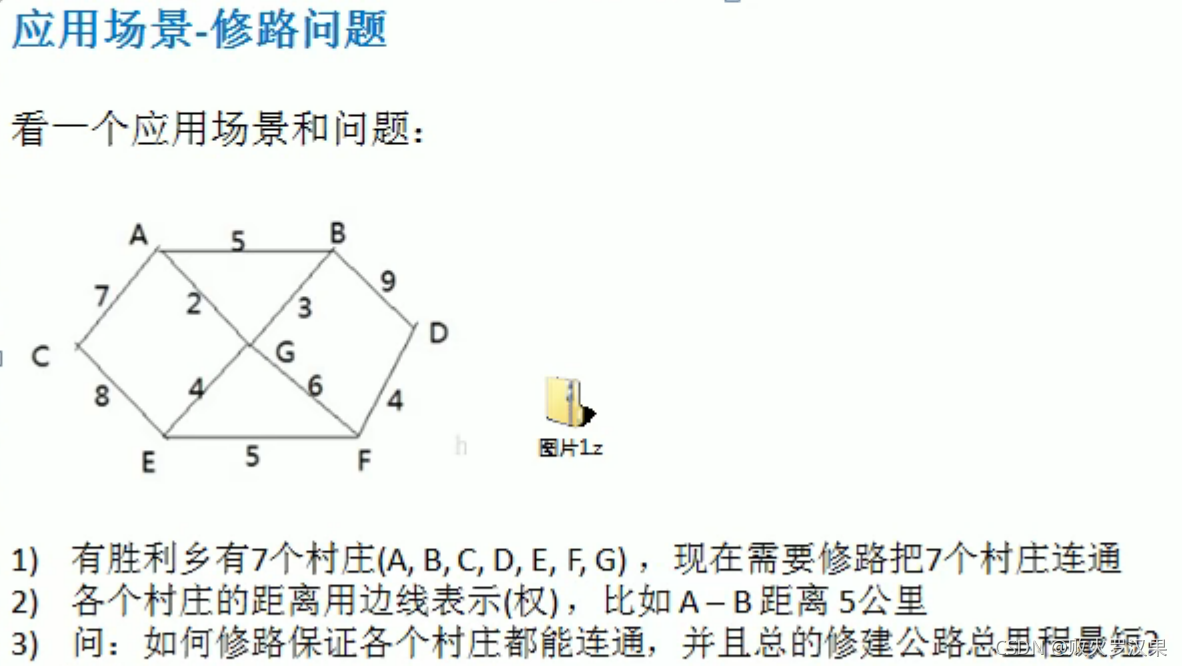
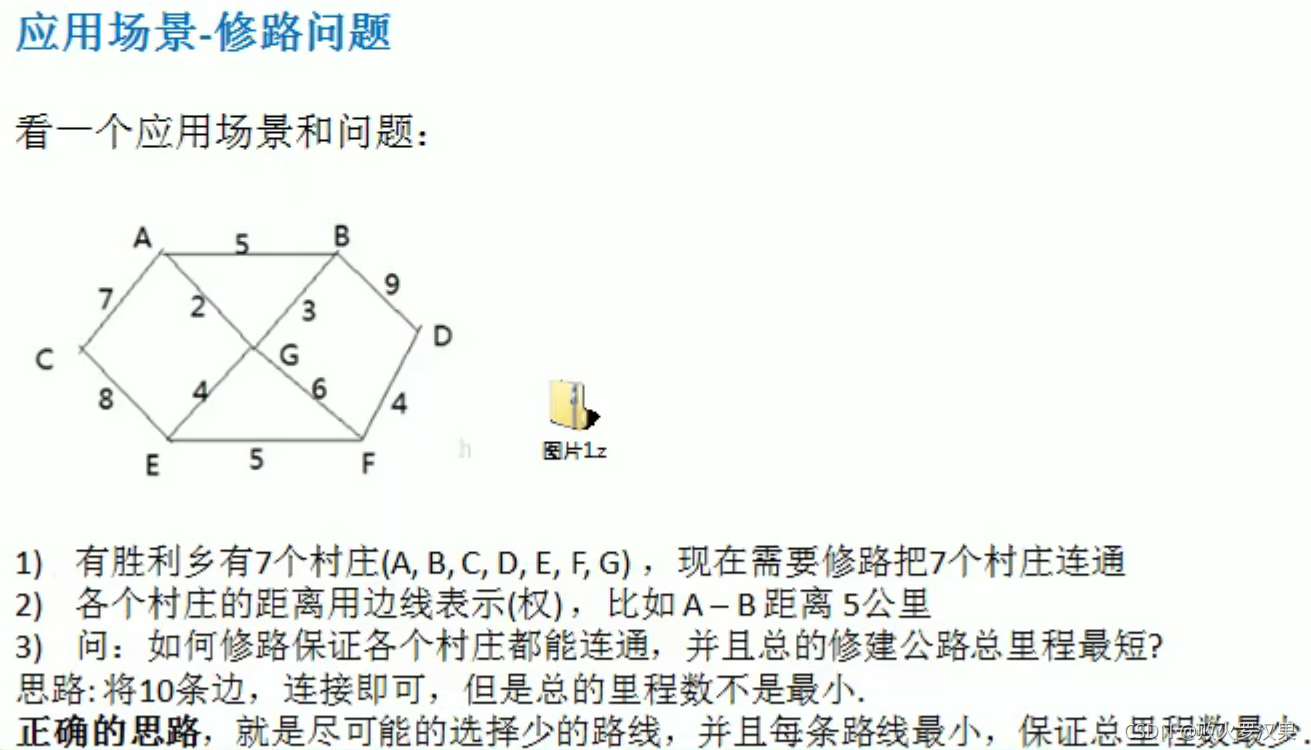
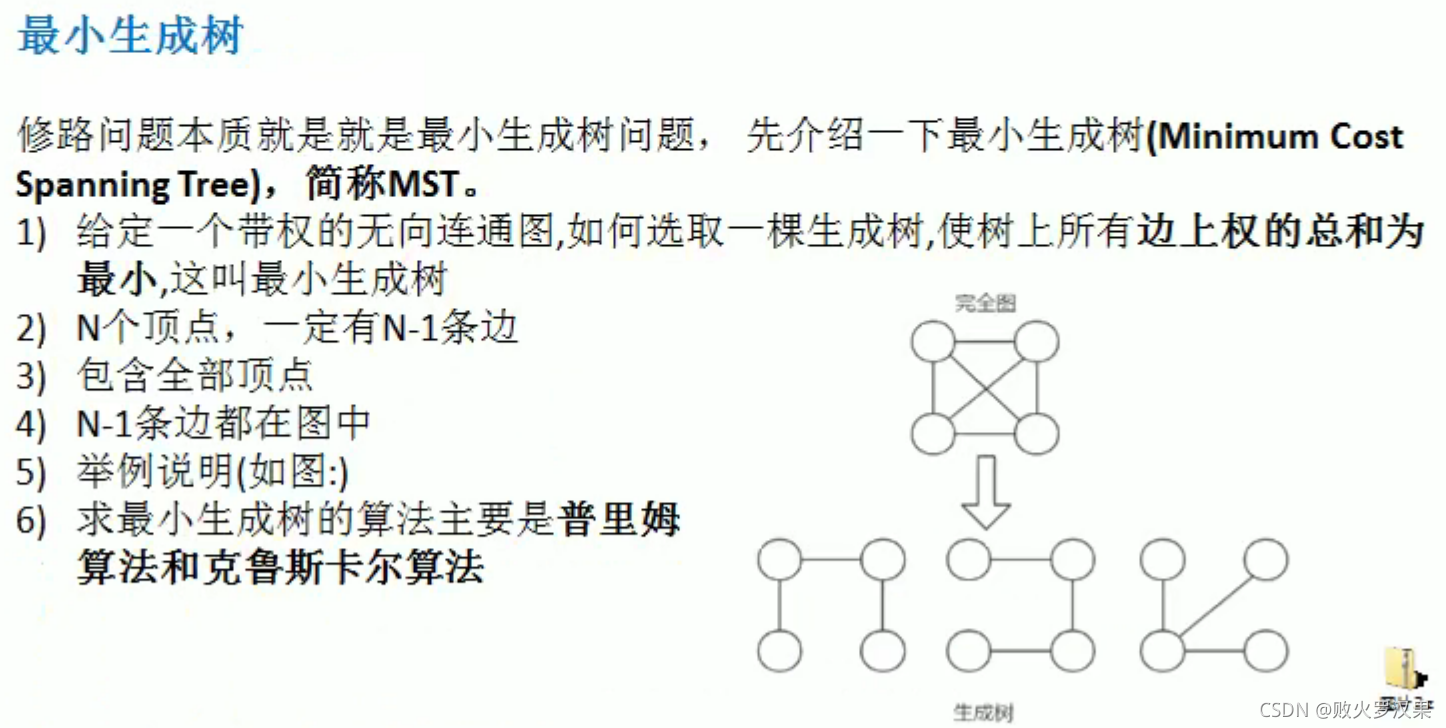
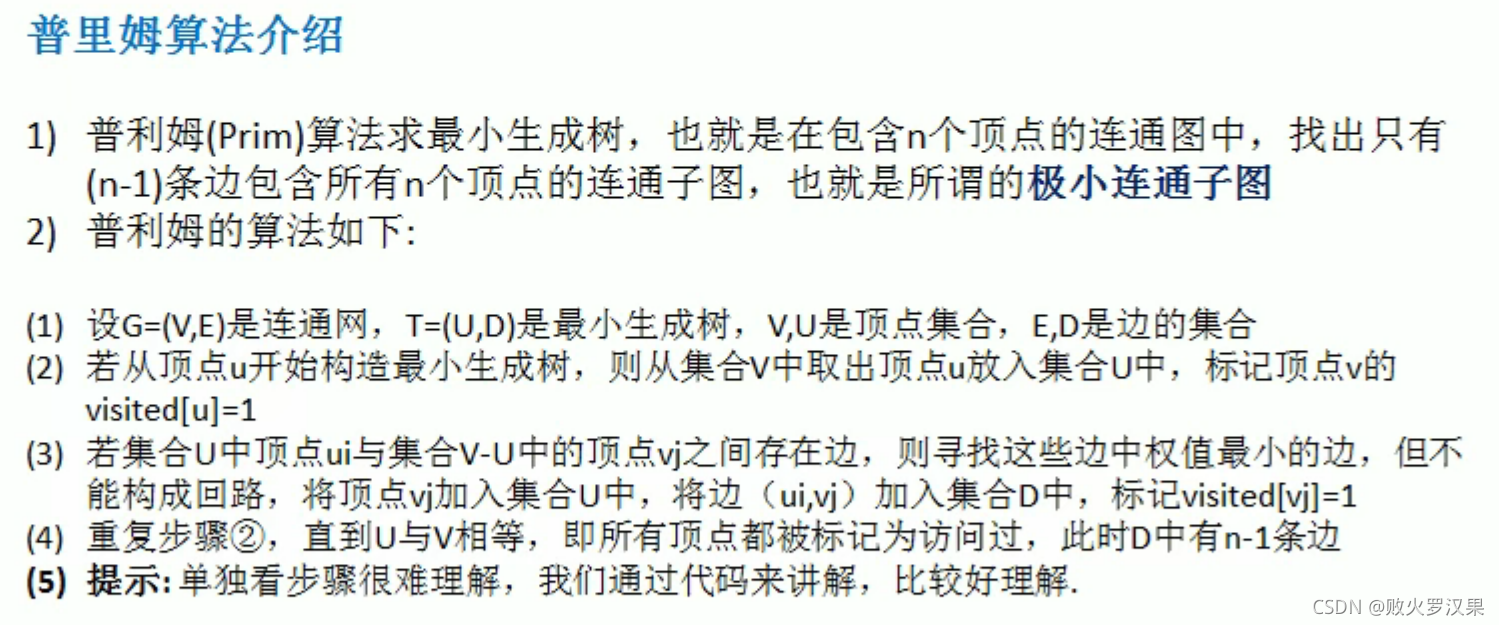
代码实现:
package Algorithm.Prim;
import java.util.Arrays;
public class Prim {
public static void main(String[] args) {
MGraph graph = new MGraph(7);
MinTree minTree = new MinTree();
char[] data ={'A','B','C','D','E','F','G'};
//临界矩阵中用很大的数表示两点之间不连通
int[][] weight = new int[][]{
{100,5,7,100,100,100,2},
{5,100,100,9,100,100,3},
{7,100,100,100,8,100,100},
{100,9,100,100,100,4,100},
{100,100,8,100,100,5,4},
{100,100,100,4,5,100,6},
{2,3,100,100,4,6,100}
};
minTree.createTree(graph, graph.count, data,weight);
minTree.showGraph(graph);
minTree.prim(graph,0);
}
}
//创建最小生成树
class MinTree{
public void createTree(MGraph graph, int count,char[] data,int[][] weight){
// for (int i = 0; i < data.length; i++) {
// graph.data[i] = data[i];
// }
graph.data = data;
graph.weight = weight;
graph.count = count;
}
public void showGraph(MGraph graph){
for (int[] link : graph.weight) {
System.out.println(Arrays.toString(link));
}
}
//v表示从第几个结点开始生成
public void prim(MGraph graph,int v){
int[] visited = new int[graph.count];
//标记为已读
visited[v]=1;
//记录两个顶点的下标
int h1 = -1;
int h2 = -1;
for (int k = 1; k < graph.count; k++) {//n个点对应n-1条边
int minWeight = 100;//初始化一个较大值
//确定每一次生成的子图,和哪个节点的距离最近
for (int i = 0; i < graph.count; i++) {//i表示已被访问过的节点,j表示相邻未访问过的节点
for (int j = 0; j < graph.count; j++) {
if (visited[i]==1&&visited[j]==0&& graph.weight[i][j]<minWeight){
minWeight = graph.weight[i][j];
h1=i;
h2=j;
}
}
}
//退出循环后找到了一条边最小
System.out.println(""+graph.data[h1]+graph.data[h2]+graph.weight[h1][h2]);
visited[h2]=1;
}
}
}
class MGraph{
int count;//表示结点的个数
char[] data;//存放节点的数据
int[][] weight;//邻接矩阵存储边的权值
public MGraph(int count) {
this.count = count;
this.data = new char[count];
this.weight = new int[count][count];
}
}