第六章:树
一、二叉树
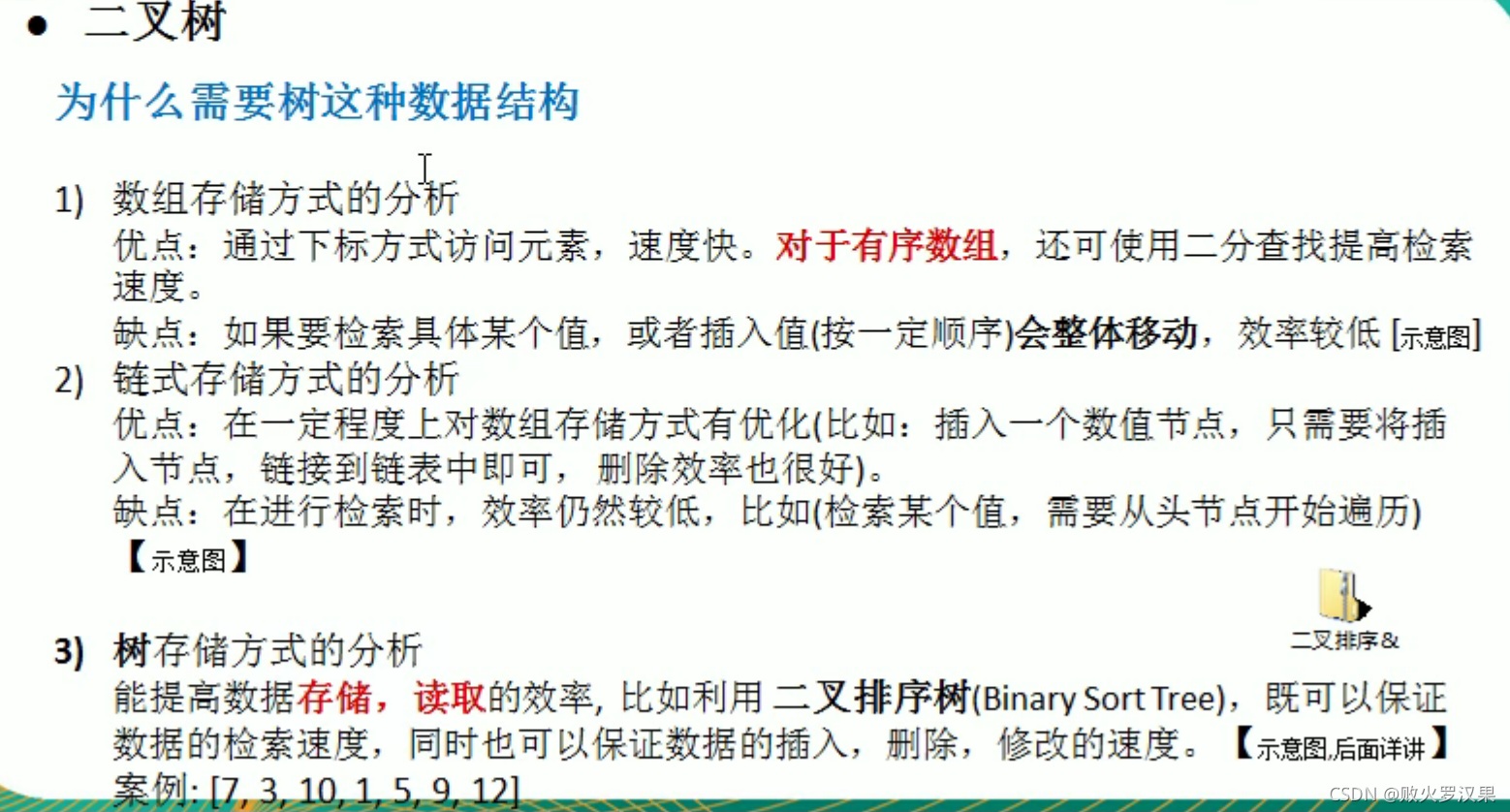
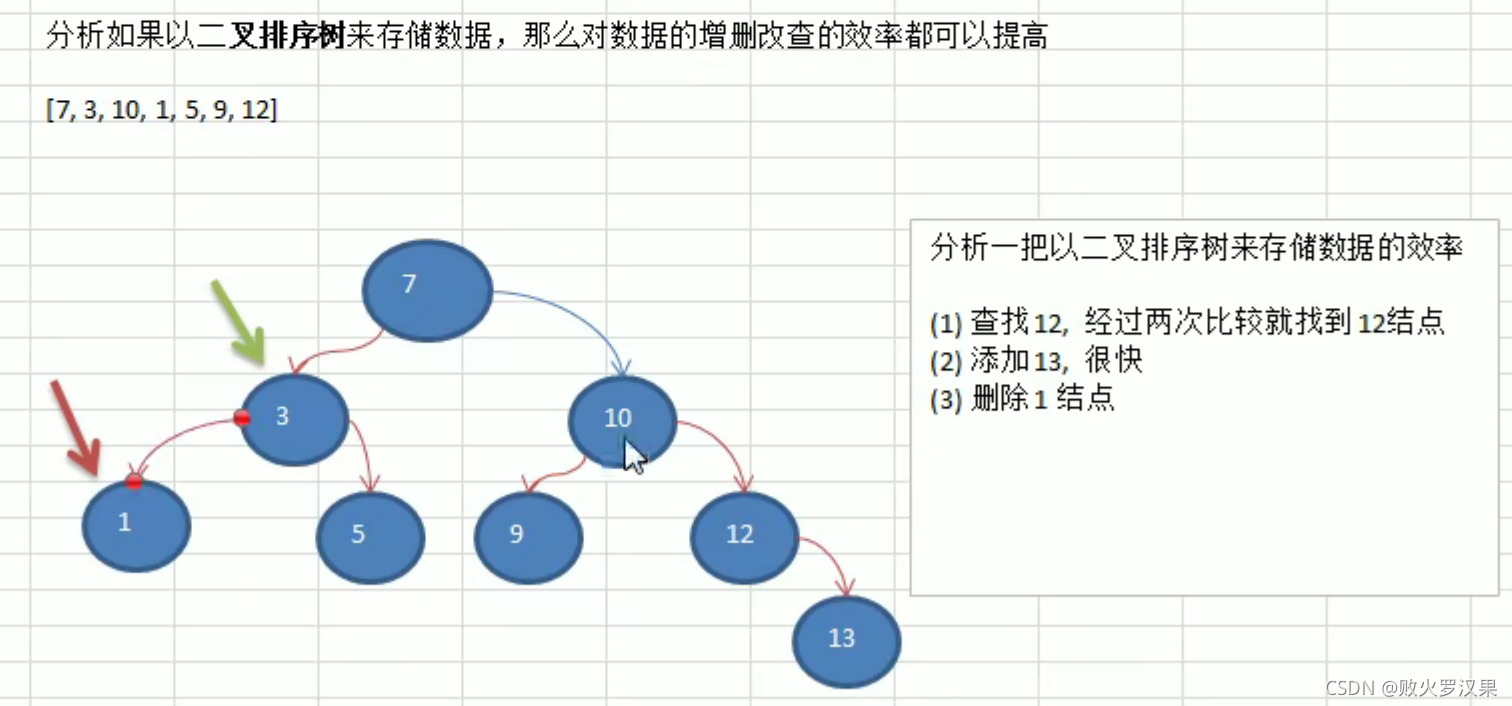
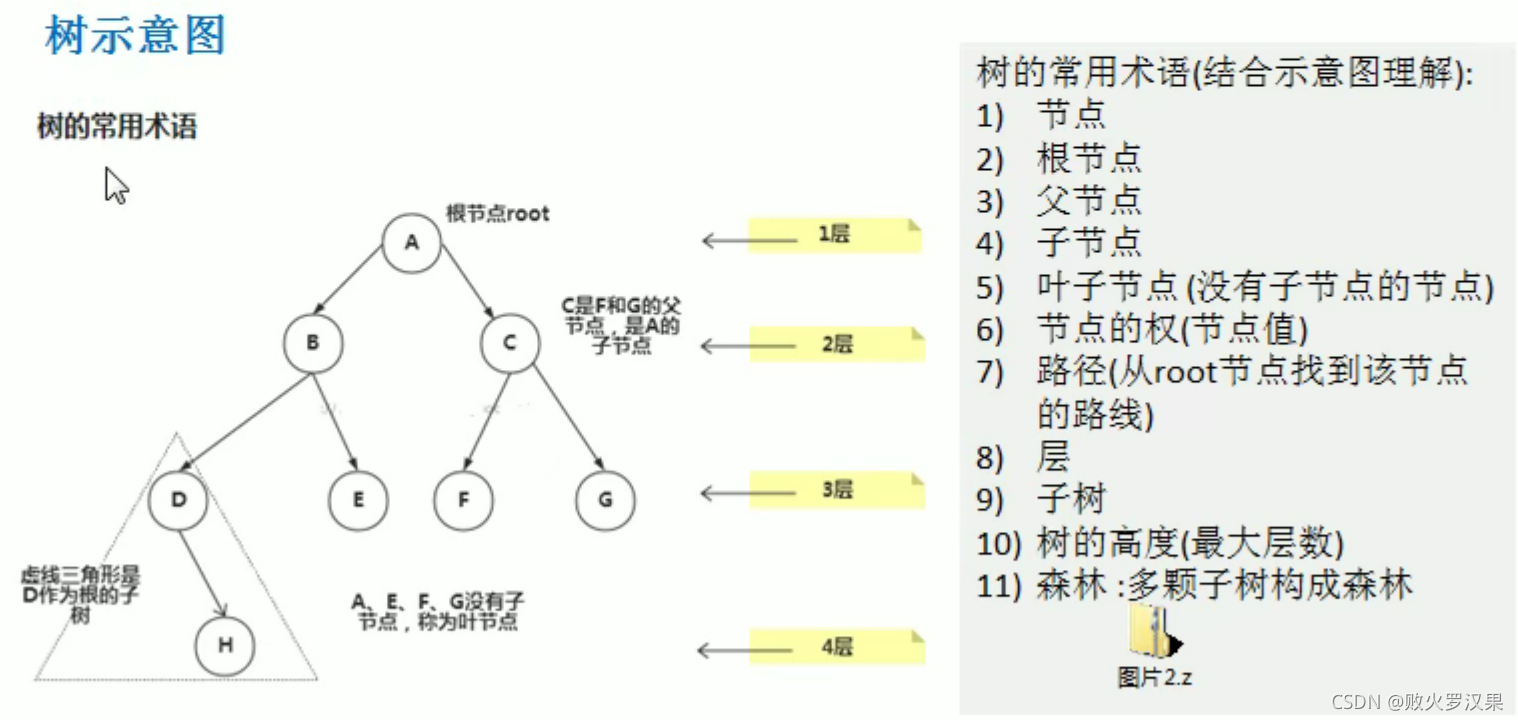
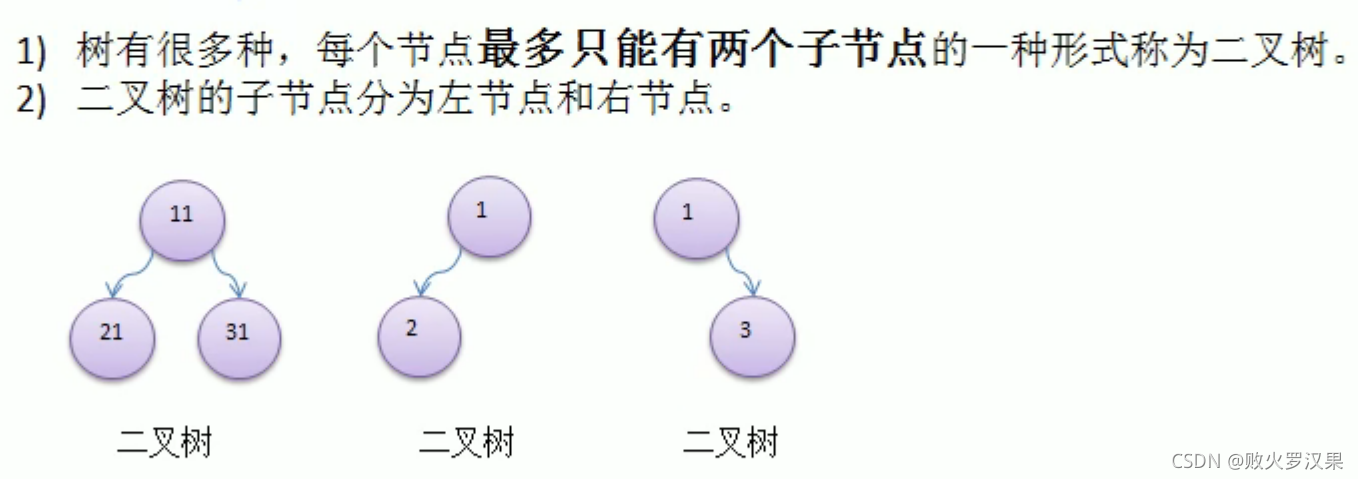
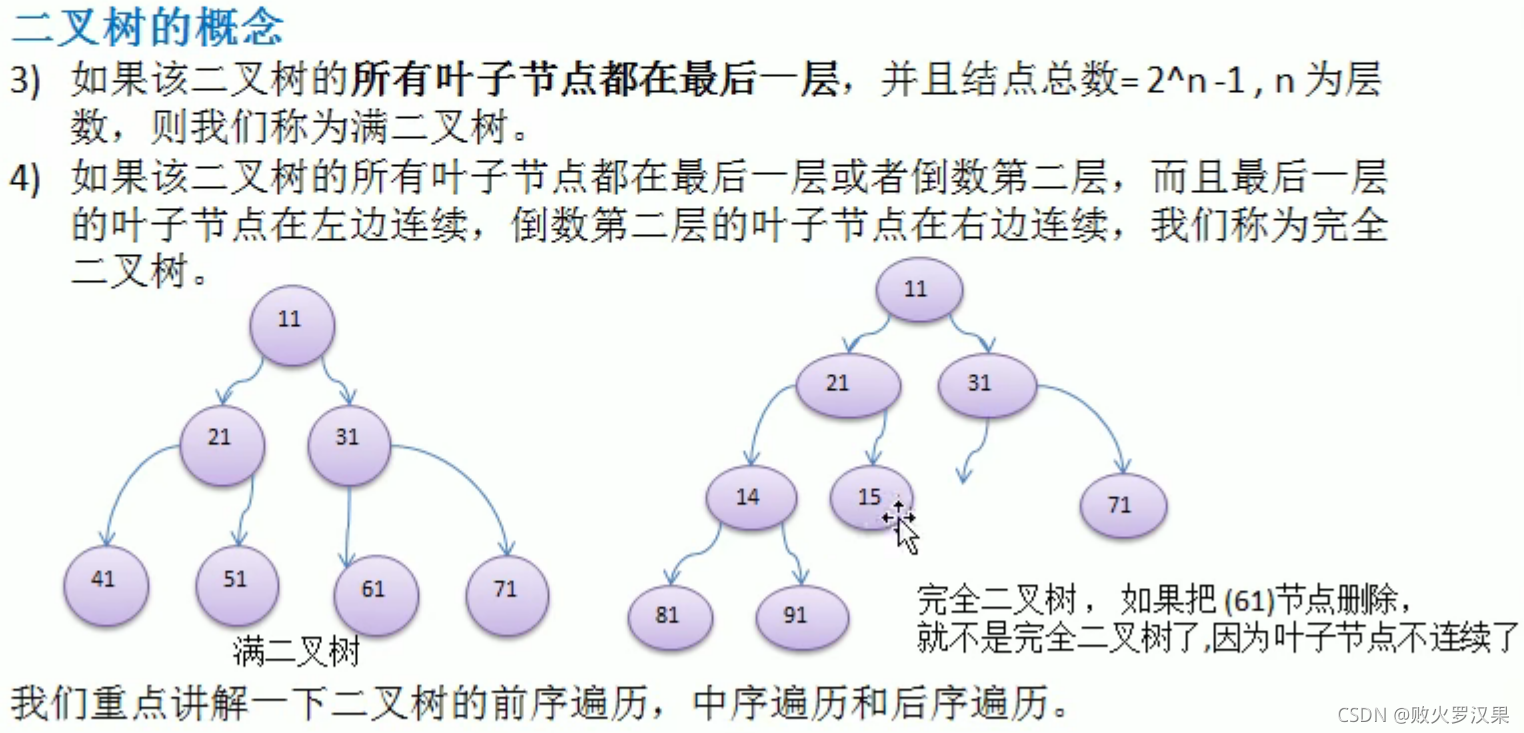
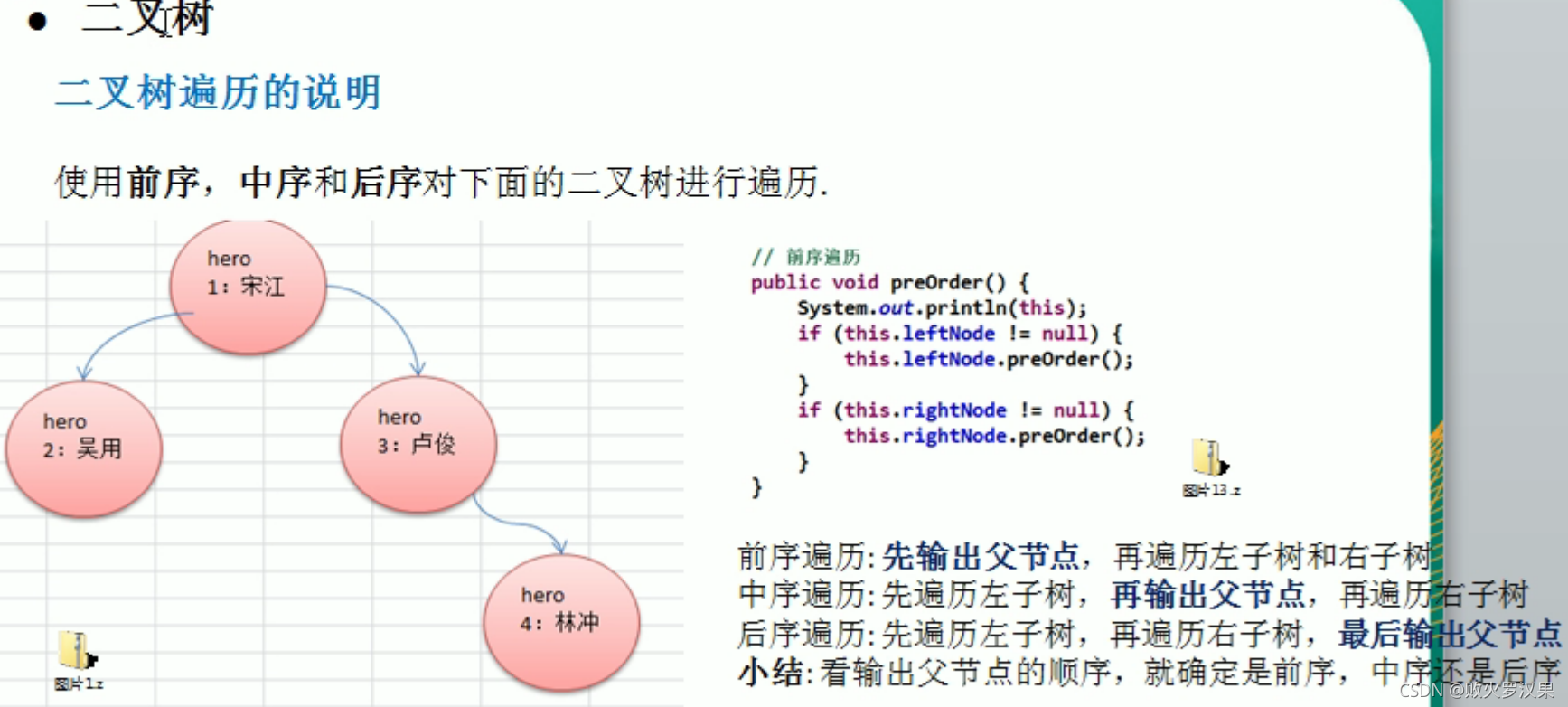
思路分析
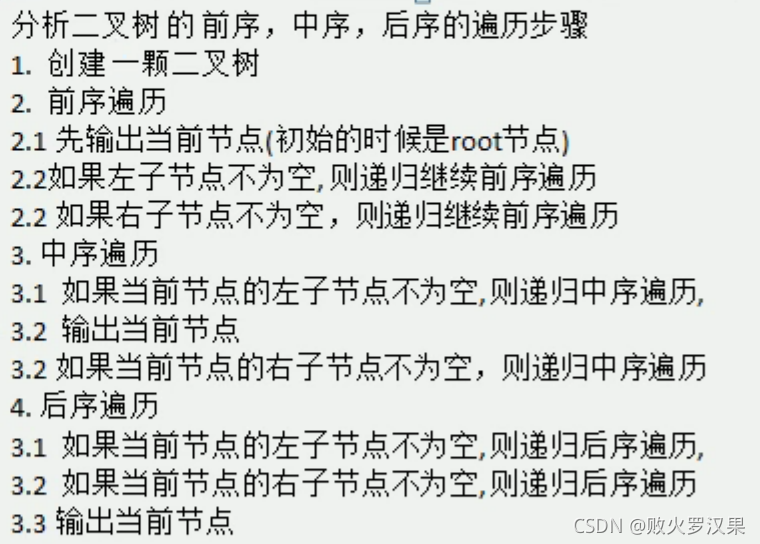
节点提供具体实现方法,树实现接口调用
二、查找指定节点
思路分析
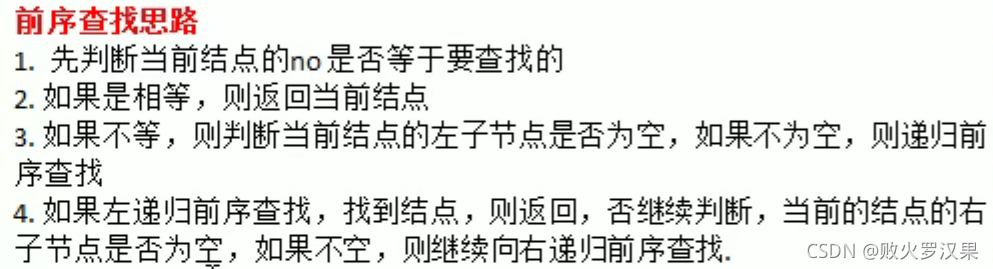
三、二叉树删除节点
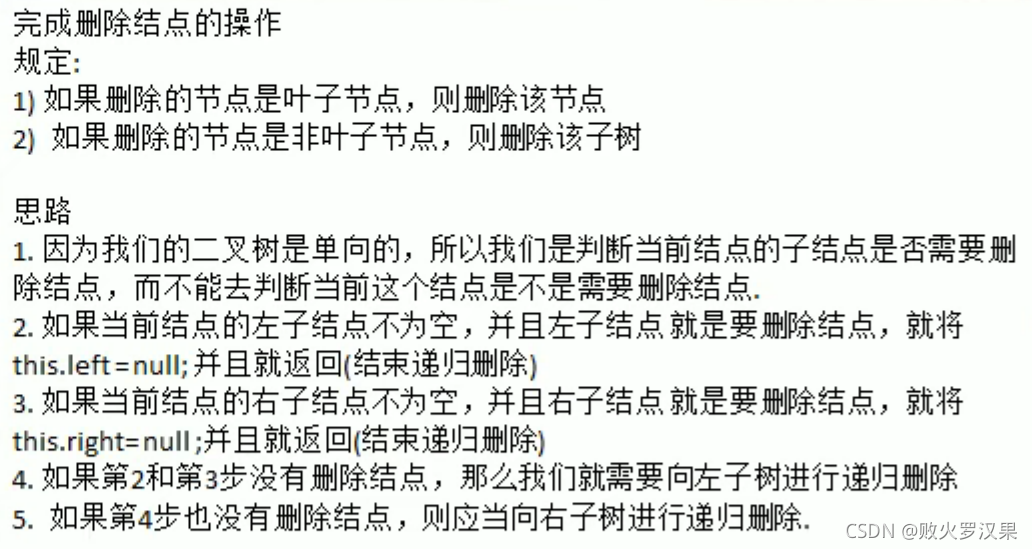
课后作业:
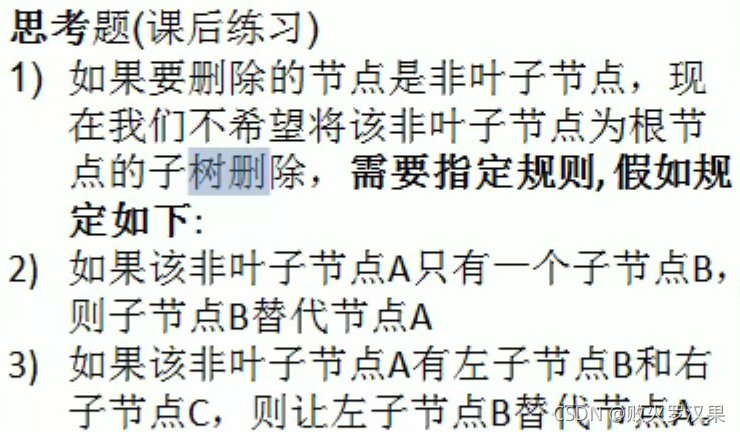
代码实现
package Tree.BinaryTree;
public class Demo01 {
public static void main(String[] args) {
//创建一棵二叉树
BinaryTree binaryTree = new BinaryTree();
//创建需要的节点
HeroNode root = new HeroNode(1, "宋江");
HeroNode n2 = new HeroNode(2, "吴用");
HeroNode n3 = new HeroNode(3, "卢俊义");
HeroNode n4 = new HeroNode(4, "林冲");
HeroNode n5 = new HeroNode(5, "nana");
//先手动创建二叉树
root.left = n2;
root.right = n3;
n3.right = n4;
n3.left = n5;
binaryTree.setRoot(root);
binaryTree.preOrder();
binaryTree.infixOrder();
binaryTree.postOrder();
System.out.println(binaryTree);
System.out.println("===================");
System.out.println(binaryTree.preOrderSearch(3));
System.out.println(binaryTree.infixOrderSearch(5));
System.out.println(binaryTree.postOrderSearch(7));
System.out.println("====================");
binaryTree.del(3);
binaryTree.preOrder();
}
}
//定义一个树
class BinaryTree {
private HeroNode root;
public void setRoot(HeroNode root) {
this.root = root;
}
//前序遍历
public void preOrder() {
if (root != null) {
this.root.preOrder();
} else {
System.out.println("当前二叉树为空,无法遍历");
}
}
//中序遍历
public void infixOrder() {
if (root != null) {
this.root.infixOrder();
} else {
System.out.println("当前二叉树为空,无法遍历");
}
}
//后序遍历
public void postOrder() {
if (root != null) {
this.root.postOrder();
} else {
System.out.println("当前二叉树为空,无法遍历");
}
}
//前序查找
public HeroNode preOrderSearch(int no) {
if (root != null) {
HeroNode res = this.root.preOrderSearch(no);
if (res.no == no) {
return res;
}
return null;
} else {
return null;
}
}
//中序查找
public HeroNode infixOrderSearch(int no) {
if (root != null) {
HeroNode res = this.root.infixOrderSearch(no);
if (res.no == no) {
return res;
}
return null;
} else {
return null;
}
}
//后序查找
public HeroNode postOrderSearch(int no) {
if (root != null) {
HeroNode res = this.root.postOrderSearch(no);
if (res.no == no) {
return res;
}
return null;
} else {
return null;
}
}
//删除
public void del(int no) {
if (root != null) {
if (root.no == no) {
root = null;
} else {
this.root.del(no);
}
} else {
System.out.println("二叉树为空,无法继续删除");
}
}
}
//先创建HeroNode节点
class HeroNode {
public int no;
public String name;
public HeroNode left;
public HeroNode right;
public HeroNode() {
}
public HeroNode(int no, String name) {
this.no = no;
this.name = name;
}
@Override
public String toString() {
return "HeroNode{" +
"no=" + no +
", name='" + name + '\'' +
'}';
}
//前序遍历的方法
public void preOrder() {
System.out.println(this);//先输出当前节点(初始化时是根节点)
if (this.left != null) {//向左递归前序遍历
this.left.preOrder();
}
if (this.right != null) {//向右递归前序遍历
this.right.preOrder();
}
}
//中序遍历的方法
public void infixOrder() {
if (this.left != null) {
this.left.infixOrder();
}
System.out.println(this);
if (this.right != null) {
this.right.infixOrder();
}
}
//后序遍历的方法
public void postOrder() {
if (this.left != null) {
this.left.postOrder();
}
if (this.right != null) {
this.right.postOrder();
}
System.out.println(this);
}
//前序查找
public HeroNode preOrderSearch(int no) {
//先判断父节点
if (this.no == no) {
return this;
} else {
HeroNode resNode = null;
if (this.left != null) {//递归前序查找
resNode = this.left.preOrderSearch(no);
}
if (resNode != null) {//说明左子树找到
return resNode;
}
if (this.right != null) {
resNode = this.right.preOrderSearch(no);
}
return resNode;//为空说明都没找到,不为空说明在右子树找到,最后的判断放在上层做
}
}
//中序查找
public HeroNode infixOrderSearch(int no) {
HeroNode resNode = null;
if (this.left != null) {//递归前序查找
resNode = this.left.infixOrderSearch(no);
}
if (resNode != null) {//说明左子树找到
return resNode;
}
if (this.no == no) {
return this;
}
if (this.right != null) {
resNode = this.right.infixOrderSearch(no);
}
return resNode;
}
//后序查找
public HeroNode postOrderSearch(int no) {
HeroNode resNode = null;
if (this.left != null) {
resNode = this.left.postOrderSearch(no);
}
if (resNode != null) {
return resNode;
}
if (this.right != null) {
resNode = this.right.postOrderSearch(no);
}
if (resNode != null) {
return resNode;
}
return this;
}
//删除
public void del(int no) {
if (this.left != null && this.left.no == no) {
if (this.left.left!=null){
this.left = this.left.left;
} else if (this.left.right!=null){
this.left = this.left.right;
} else {
this.left=null;
}
return;
}
if (this.right != null && this.right.no == no) {
if (this.right.left!=null){
this.right = this.right.left;
} else if (this.right.right!=null){
this.right = this.right.right;
} else {
this.right=null;
}
return;
}
if (this.left != null) {
this.left.del(no);
}
if (this.right != null) {
this.right.del(no);
}
}
}