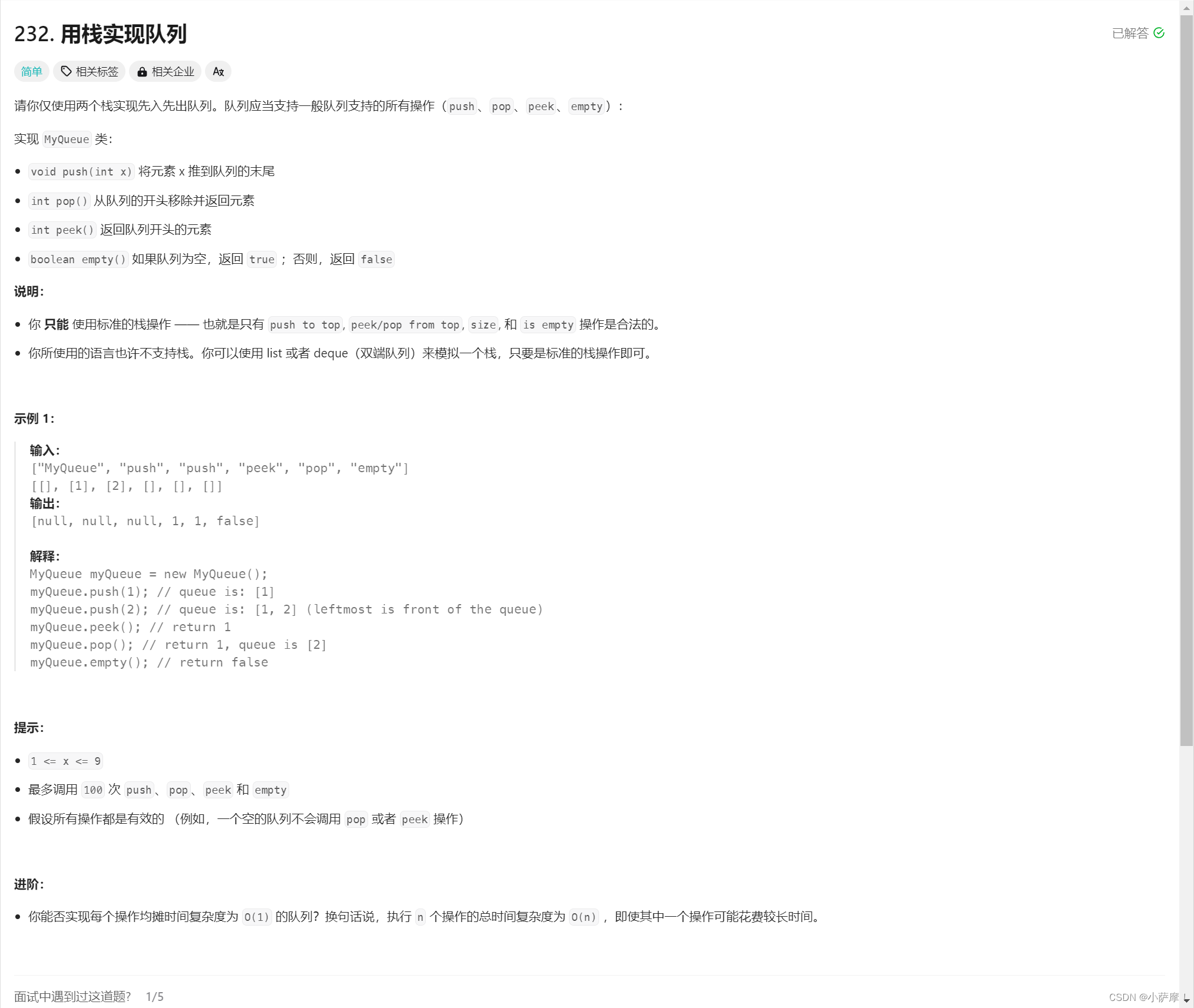
var MyQueue = function() {
this.input = [];
this.output = [];
};
MyQueue.prototype.move = function(x) {
if(this.output.length) {
return true;
}
this.output = this.input.reverse().concat(this.output);
this.input = [];
}
MyQueue.prototype.push = function(x) {
this.input.push(x);
};
MyQueue.prototype.pop = function() {
this.move();
return this.output.pop();
};
MyQueue.prototype.peek = function() {
this.move();
return this.output[this.output.length - 1];
};
MyQueue.prototype.empty = function() {
return !this.output.length && !this.input.length
};