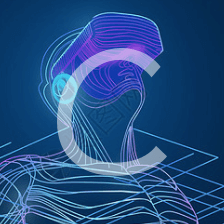
leetcode
溪水流不停
远处的是风景,近处的才是人生!
展开
-
leetcode:Binary Tree Inorder Traversal
class Solution {public: vector inorderTraversal(TreeNode *root) { vector res; stack s; TreeNode * p = root; while(p!=NULL||!s.empty()) { while(原创 2015-04-02 16:32:22 · 555 阅读 · 0 评论 -
leetcode:Climbing Stairs
class Solution {public: int climbStairs(int n) { vector<int> table; table.push_back(0); table.push_back(1); table.push_back(2); if(n<=2) return原创 2015-04-03 21:15:45 · 516 阅读 · 0 评论 -
leetcode:Subsets
class Solution {public: vector<vector<int> > subsets(vector<int> &S) { vector<vector<int>> res; vector<int> list; if(S.size()==0) { res.push_back(list);原创 2015-04-04 17:59:06 · 539 阅读 · 0 评论 -
leetcode:Sort Colors
1:class Solution {public: void sortColors(int A[], int n) { int b[3] = {0,0,0}; for(int i=0;i<n;i++) { b[A[i]]++; } int i=0,j=0,k=0; for原创 2015-04-11 00:43:16 · 688 阅读 · 0 评论 -
leetcode:Symmetric Tree
/** * Definition for binary tree * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode(int x) : val(x), left(NULL), right(NULL) {} * }; */class Solutio原创 2015-04-05 00:17:03 · 493 阅读 · 0 评论 -
leetcode:Number of Islands
其实该题为求连通子图的个数,可以借助DFS的思想:class Solution {public: bool dfs(int i,int j,vector<vector<char>> &grid) { if(i>=0&&i<grid.size()&&j>=0&&j<grid[i].size()&&grid[i][j]=='1') {原创 2015-04-18 13:45:09 · 3723 阅读 · 0 评论 -
leetcode:Roman to Integer
class Solution {public: int romanToInt(string s) { int map[128]; if(s.size()==0) return 0; map['I']=1; map['V']=5; map['X']=10; map['L'原创 2015-04-18 17:14:11 · 694 阅读 · 0 评论 -
leetcode:Rotate List
/** * Definition for singly-linked list. * struct ListNode { * int val; * ListNode *next; * ListNode(int x) : val(x), next(NULL) {} * }; */class Solution {public: ListNode *rot原创 2015-04-11 23:10:26 · 568 阅读 · 0 评论 -
leetcode:Factorial Trailing Zeroes
注意越界!class Solution {public: int trailingZeroes(int n) { int sum = 0; long long i =5; while(i<=n) { sum += n/i; i *= 5; } re原创 2015-04-11 22:16:44 · 618 阅读 · 0 评论 -
leetcode:Search for a Range
class Solution {public: int searchLowerBound(int A[], int n, int target) { int left=0,right=n-1,res=-1; while(left<=right) { int mid = left+((right-left)>>原创 2015-04-01 16:09:30 · 355 阅读 · 0 评论 -
leetcode:Permutations
class Solution { vector > ret; int N; public: void perm(vector &num, int i){ if( i == N){ ret.push_back(num); } for(int j = i; j < N;原创 2015-04-01 16:08:24 · 384 阅读 · 0 评论 -
leetcode:Partition List
/** * Definition for singly-linked list. * struct ListNode { * int val; * ListNode *next; * ListNode(int x) : val(x), next(NULL) {} * }; */class Solution {public: ListNode *pa原创 2015-04-01 16:07:05 · 412 阅读 · 0 评论 -
leetcode:Binary Tree Preorder Traversal
class Solution {public: vector preorderTraversal(TreeNode *root) { vector res; stack s; TreeNode * p = root; while(p!=NULL||!s.empty()) { while原创 2015-04-02 16:30:19 · 574 阅读 · 0 评论 -
leetcode:Binary Tree Postorder Traversal
class Solution {public: vector postorderTraversal(TreeNode *root) { vector res; stack>s; TreeNode *p = root; while(p!=NULL||!s.empty()) { while原创 2015-04-02 16:29:08 · 662 阅读 · 0 评论 -
leetcode:Divide Two Integers
class Solution {public: int divide(int dividend, int divisor) { long long div = dividend,dis = divisor; div = abs(div); dis = abs(dis); long long res = 0; w原创 2015-04-03 23:38:49 · 614 阅读 · 0 评论 -
leetcode:Populating Next Right Pointers in Each Node
/** * Definition for binary tree with next pointer. * struct TreeLinkNode { * int val; * TreeLinkNode *left, *right, *next; * TreeLinkNode(int x) : val(x), left(NULL), right(NULL), next(NULL) {原创 2015-04-03 20:14:13 · 523 阅读 · 0 评论 -
leetcode:Rotate Array
class Solution {public: void rotate(int nums[], int n, int k) { k = k%n; reverse(nums,0,n-k-1); reverse(nums,n-k,n-1); reverse(nums,0,n-1); } voi原创 2015-04-01 16:04:57 · 353 阅读 · 0 评论 -
leetcode:Repeated DNA Sequences
class Solution {public: char hash[1024*1024]; vector findRepeatedDnaSequences(string s) { vector res; if(s.size()<10) return res; char convertor[26];原创 2015-04-01 16:06:37 · 452 阅读 · 0 评论 -
leetcode:Number of 1 Bits
1:class Solution {public: int hammingWeight(uint32_t n) { int count = 0; while(n) { if(n&0x1==1) { count++; }原创 2015-04-01 16:01:27 · 427 阅读 · 0 评论 -
leetcode:Reverse Bits
class Solution {public: uint32_t reverseBits(uint32_t n) { uint32_t m = 0; for(int i=0;i<32;i++) { m = (m<<1)+(n&0x1); n = n>>1; }原创 2015-04-01 16:03:00 · 413 阅读 · 0 评论 -
leetcode:Validate Binary Search Tree
最开始我犯了个错误,直接递归判断根节点的值是否在左孩子节点和右孩子节点值得中间,忽略了应该是根节点的值大于左子树所有节点的值,小于右子树所有节点的值,导致代码如下:bool isValidBST(TreeNode* root) { if(root==NULL) return true; else if(root->left&&!root->ri原创 2015-05-29 00:28:15 · 992 阅读 · 0 评论