目录
首先搭建一个SpringBoot框架,参见:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.0</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.qcby</groupId>
<artifactId>springboot2</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>springboot2</name>
<description>springboot2</description>
<properties>
<java.version>8</java.version>
</properties>
<dependencies>
<!-- Spring Boot Starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<!-- Spring Boot Starter Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Boot Starter Test -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- Spring Boot DevTools -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<optional>true</optional>
</dependency>
<!-- Spring Boot Starter Thymeleaf -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- jQuery WebJar -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>jquery</artifactId>
<version>3.3.1</version>
</dependency>
<!-- Spring Boot Starter JDBC -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<!-- Druid 数据库连接池 -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.2.8</version>
</dependency>
<!-- MySQL 数据库驱动 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<!-- MyBatis Spring Boot Starter -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.4</version> <!-- 更新版本以兼容Spring Boot 2.7 -->
</dependency>
<!-- Log4j 日志框架 -->
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
注:我演示的jdk版本比较低,所以框架版本用的是2.7.*系列(这里用的是2.7.0),然后里面是能够互相兼容的一套版本。
一、数据源配置
在默认情况下, 数据库连接可以使用DataSource池进行自动配置,如果Hikari可用, Springboot将使用它。
在 Spring Boot 2.x 及以后的版本中,默认的数据库连接池是 HikariCP,所在这里引入Druid连接池。
老规矩,先来解读一下springboot框架自配的数据源的源码(存在这个路径下:org.springframework.boot.autoconfigure.jdbc.DataSourceProperties)
配置项
在配置文件中可以修改这些属性:
配置项 | 描述 | 对应字段 |
---|---|---|
spring.datasource.url | 数据库连接URL | url |
spring.datasource.username | 数据库用户名 | username |
spring.datasource.password | 数据库密码 | password |
spring.datasource.driver-class-name | 数据库驱动类名 | driverClassName |
spring.datasource.type | 数据源类型(如 HikariCP、Druid 等) | type |
spring.datasource.hikari.* | HikariCP 的特定配置项 | hikari (嵌套属性) |
spring.datasource.druid.* | Druid 的特定配置项 | druid (嵌套属性) |
注:
这里面的两个属性需要导入相应的依赖后才会存在。
spring.datasource.hikari.*
spring.datasource.druid.*
示例
根据上面的属性,就可以在application.yml中编写(application.properties也行,只是格式问题):
spring:
datasource:
# 数据库用户名
username: root
# 数据库密码
password: 2020
# 数据库连接 URL
url: jdbc:mysql://localhost:3306/boot_demo
# 数据库驱动类名,推荐使用 MySQL 8.x 的驱动
driver-class-name: com.mysql.cj.jdbc.Driver
# 指定使用 Druid 数据源
type: com.alibaba.druid.pool.DruidDataSource
# 初始化时创建的连接数
initialSize: 5
# 最小空闲连接数
minIdle: 5
# 最大活跃连接数
maxActive: 20
# 获取连接的最大等待时间(单位:毫秒)
maxWait: 60000
# 检测连接是否空闲的周期(单位:毫秒)
timeBetweenEvictionRunsMillis: 60000
# 连接空闲多久后被释放(单位:毫秒)
minEvictableIdleTimeMillis: 300000
# 用于检测连接是否有效的 SQL 查询
validationQuery: SELECT 1 FROM DUAL
# 是否在空闲时检测连接
testWhileIdle: true
# 是否在借出连接时检测
testOnBorrow: false
# 是否在归还连接时检测
testOnReturn: false
# 是否缓存预编译语句,可以提高性能
poolPreparedStatements: true
# Druid 的监控过滤器
filters: stat,wall,log4j
# 每个连接缓存的预编译语句数量
maxPoolPreparedStatementPerConnectionSize: 20
# 是否使用全局数据源统计
useGlobalDataSourceStat: true
# Druid 连接属性配置
connectionProperties: druid.stat.mergeSql=true;druid.stat.slowSqlMillis=500
上面的配置以作参考,可以根据自己的需求自行更改。
编写数据源配置类
package com.qcby.sbdatedemo.config;
import com.alibaba.druid.pool.DruidDataSource;
import com.alibaba.druid.support.http.StatViewServlet;
import com.alibaba.druid.support.http.WebStatFilter;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.boot.web.servlet.FilterRegistrationBean;
import org.springframework.boot.web.servlet.ServletRegistrationBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import javax.sql.DataSource;
import java.util.*;
/**
* 创建数据源注册类
* */
@Configuration
public class DruidConfig {
@ConfigurationProperties(prefix = "spring.datasource")
@Bean
public DataSource dataSource(){
return new DruidDataSource();
}
/**
* 配置druid运行期监控
* */
@Bean
public ServletRegistrationBean statViewServlet(){
ServletRegistrationBean bean = new ServletRegistrationBean(new StatViewServlet(),
"/druid/*");
Map<String,String> initParams = new HashMap<>();
initParams.put("loginUsername","root"); //用户名
initParams.put("loginPassword","root"); //密码
initParams.put("allow","");//默认就是允许所有访问
initParams.put("deny","192.168.15.21");
bean.setInitParameters(initParams);
return bean;
}
//2、配置一个web监控的filter
@Bean
public FilterRegistrationBean webStatFilter(){
FilterRegistrationBean bean;
bean = new FilterRegistrationBean();
bean.setFilter(new WebStatFilter());
Map<String,String> initParams = new HashMap<>();
initParams.put("exclusions","*.js,*.css,/druid/*");
bean.setInitParameters(initParams);
bean.setUrlPatterns(Arrays.asList("/*"));
return bean;
}
}
这里为什么用配置类不用配置文件可以参考下面这篇文章中的对比表格:
配置好后运行,访问这个路径:http://localhost:8080/druid
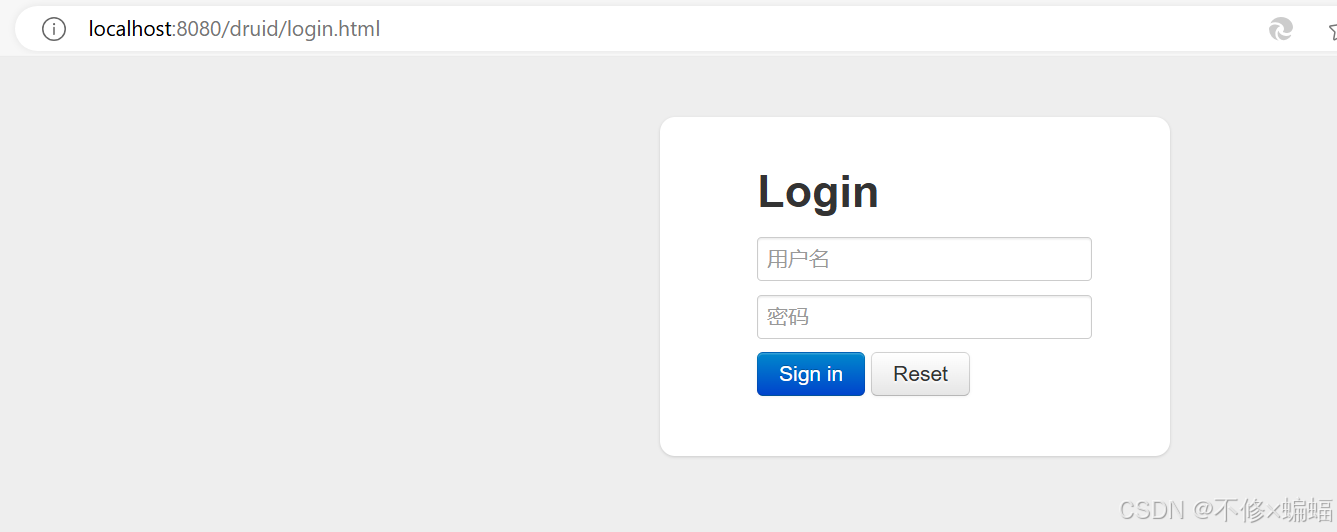
然后输入自己提前配置的密码和用户名:
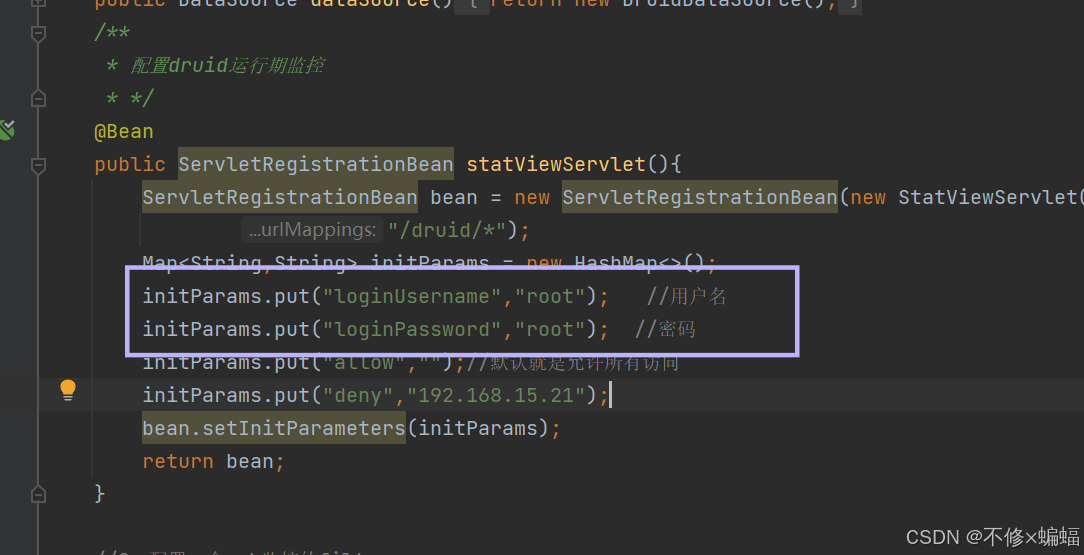
就可以进到这里面:
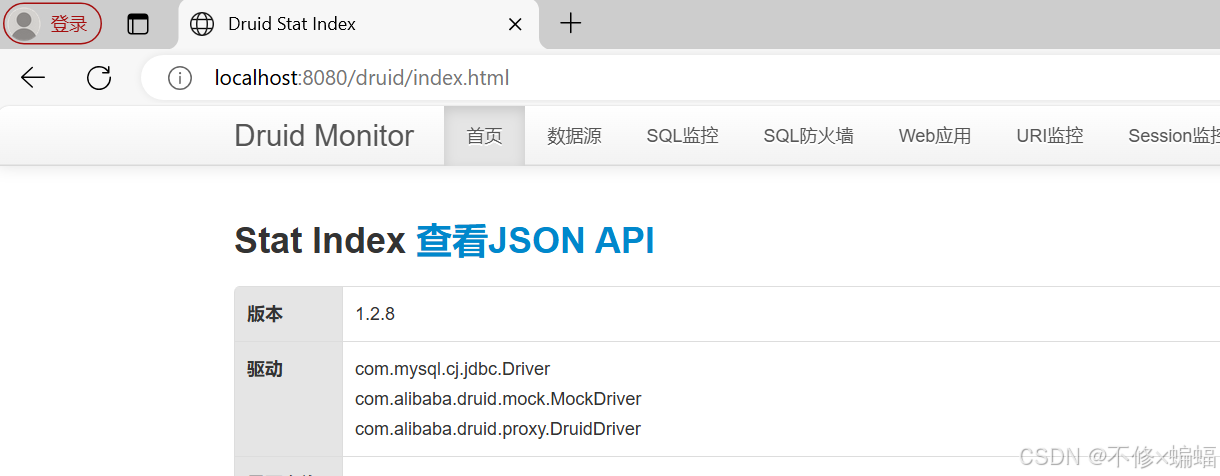
二、springboot整合jdbcTemplate
在数据源建表
SET FOREIGN_KEY_CHECKS=0;
-- ----------------------------
-- Table structure for tx_user
-- ----------------------------
DROP TABLE IF EXISTS `tx_user`;
CREATE TABLE `tx_user` (
`username` varchar(10) DEFAULT NULL,
`userId` int(10) NOT NULL,
`password` varchar(10) DEFAULT NULL,
PRIMARY KEY (`userId`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
如下:
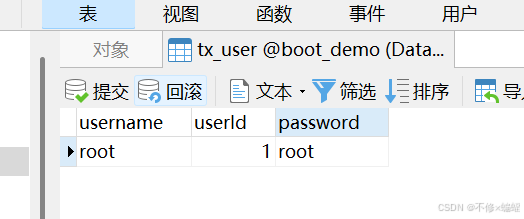
附带上我的目录结构:
![]()
不用管我这里写了很多文件,只看一下目录结构
创建 controller类
package com.qcby.sbdatedemo.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.jdbc.core.JdbcTemplate;
import java.util.*;
@Controller
public class TestController {
@Autowired
JdbcTemplate jdbcTemplate;
@ResponseBody
@RequestMapping("/query")
public List<Map<String, Object>> query(){
List<Map<String, Object>> maps = jdbcTemplate.queryForList("SELECT * FROM tx_user");
return maps;
}
}
启动项目,访问:http://localhost:8080/query
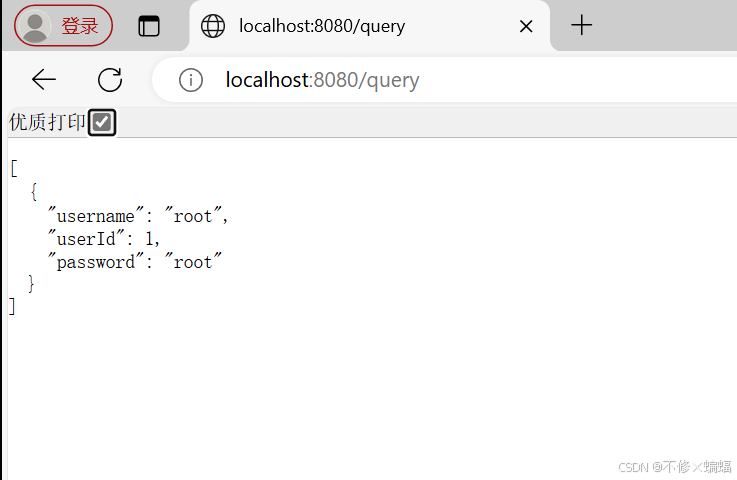
注:Springboot中提供了JdbcTemplateAutoConfiguration的自动配置(就在这个路径的包下:org.springframework.boot.autoconfigure.jdbc.JdbcTemplateAutoConfiguration)
源码如下:
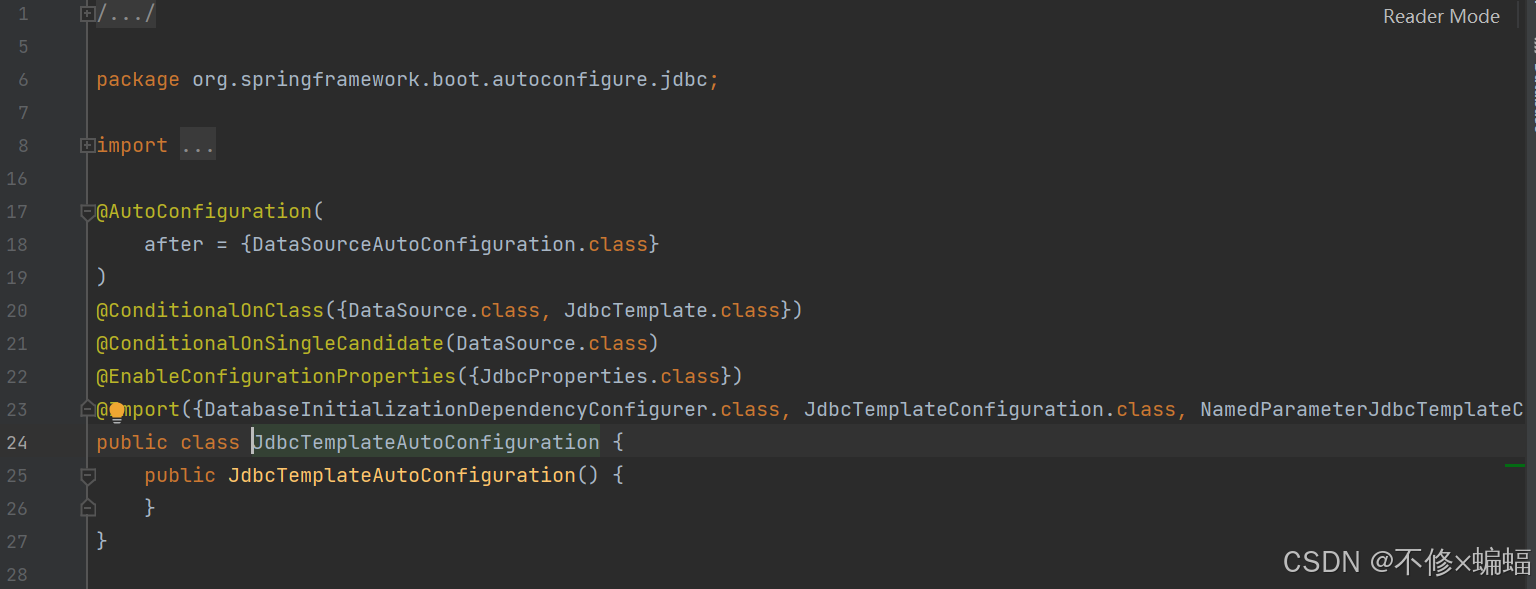
三、Springboot整合mybatis注解版
依赖:(当然文章开头的pom.xml参考中已经涵盖了这部分):
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>1.3.1</version>
</dependency>
配置数据源相关属性
前面已经在配置类中实现了
给数据库建表
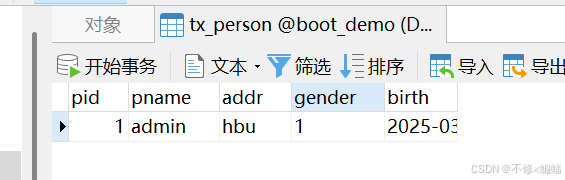
创建JavaBean(实体类)
package com.qcby.sbdatedemo.entity;
import java.util.Date;
public class TxPerson {
private int pid;
private String pname;
private String addr;
private int gender;
private Date birth;
public int getPid() {
return pid;
}
public void setPid(int pid) {
this.pid = pid;
}
public String getPname() {
return pname;
}
public void setPname(String pname) {
this.pname = pname;
}
public String getAddr() {
return addr;
}
public void setAddr(String addr) {
this.addr = addr;
}
public int getGender() {
return gender;
}
public void setGender(int gender) {
this.gender = gender;
}
public Date getBirth() {
return birth;
}
public void setBirth(Date birth) {
this.birth = birth;
}
@Override
public String toString() {
return "TxPerson{" +
"pid=" + pid +
", pname='" + pname + '\'' +
", addr='" + addr + '\'' +
", gender=" + gender +
", birth=" + birth +
'}';
}
}
创建Mapper
package com.qcby.sbdatedemo.mapper;
import com.qcby.sbdatedemo.entity.TxPerson;
import org.apache.ibatis.annotations.*;
import java.util.*;
@Mapper
public interface TxPersonMapper {
@Select("select * from tx_person")
public List<TxPerson> getPersons();
@Select("select * from tx_person t where t.pid = #{id}")
public TxPerson getPersonById(int id);
@Options(useGeneratedKeys =true, keyProperty = "pid")
@Insert("insert into tx_person(pid, pname, addr,gender, birth)" +
" values(#{pid}, #{pname}, #{addr},#{gender}, #{birth})")
public void insert(TxPerson person);
@Delete("delete from tx_person where pid = #{id}")
public void update(int id);
}
配置解决命名
package com.qcby.sbdatedemo.config;
import org.mybatis.spring.boot.autoconfigure.ConfigurationCustomizer;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
/**
* 解决驼峰模式和数据库中下划线不能映射问题的配置类(加@Configuration类的配置文件作用范围是项目整体)
* */
@Configuration
public class MybatisConfig {
@Bean
public ConfigurationCustomizer getCustomizer(){
return new ConfigurationCustomizer() {
@Override
public void customize(org.apache.ibatis.session.Configuration configuration) {
configuration.setMapUnderscoreToCamelCase(true);
}
};
}
}
编写Test类
package com.qcby.sbdatedemo.test;
import com.qcby.sbdatedemo.entity.TxPerson;
import com.qcby.sbdatedemo.mapper.TxPersonMapper;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.context.ApplicationContext;
import org.springframework.test.context.junit.jupiter.SpringExtension;
import javax.sql.DataSource;
import static org.junit.jupiter.api.Assertions.assertNotNull;
@ExtendWith(SpringExtension.class)
@SpringBootTest
public class TxPersonMapperTest {
@Autowired
private TxPersonMapper txPersonMapper;
@Autowired
private ApplicationContext context; // 注入 ApplicationContext
@Test
public void contextLoads() {
DataSource bean = (DataSource) context.getBean("dataSource");
assertNotNull(bean);
System.out.println(bean);
}
@Test
public void testMybatis() {
TxPerson p = txPersonMapper.getPersonById(1);
assertNotNull(p);
System.out.println(p);
}
}
运行
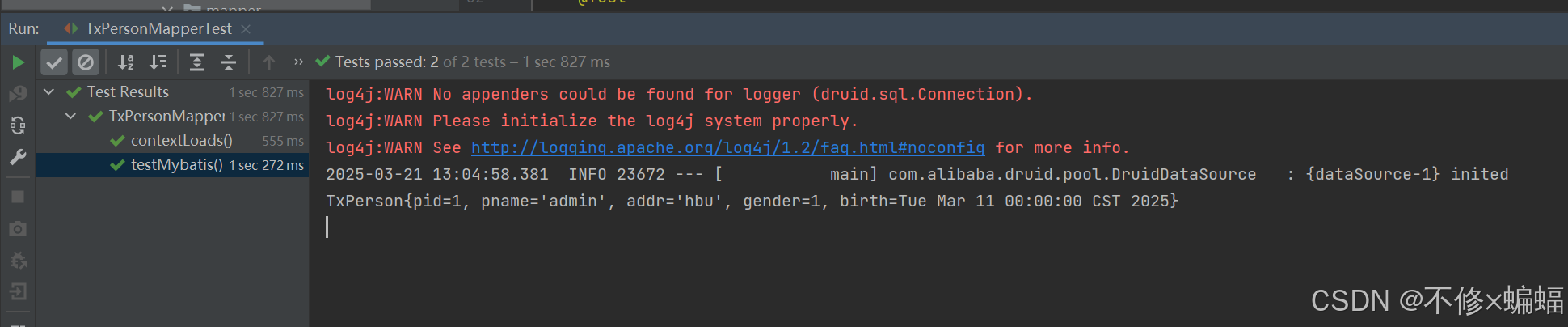