目录
1.1.IOC构造注入 (constructor-arg):
异常通知: 在出现异常时,Spring AOP会自动捕获异常,并使用代理对象处理。
最终通知 Spring AOP的最终通知是通过finally实现的,无论发生任何情况,都会执行。
环绕通知 环绕通知功能非常强大,包含了四种全部通知类型,主要是通过暴露的原生方法调用所执行的目标方法,因此可以获取到原生方法的所有状态加以处理
3.3 使用Autowired或者是Resources来完成DI依赖注入
4.2 在配置文件中,添加开启自动代理的功能,不然Annotation不会运行
1.IOC的注入方式扩展:
以往我们完成注入的方式是通过基本参数类型进行注入,那么引用数据类型呢?答案当然是可以的,接下来,来学习其他注入的几种方式:
1.1.IOC构造注入 (constructor-arg):
使用内部bean
使用ref引用形式
index(按位置) type (按类型) name(按名称)
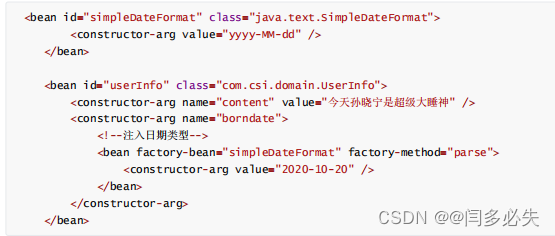
1.2 设值注入(set) 与构造注入的区别
1.3 集合、数组、Map、Properties类型注入
2. AOP扩展使用
//添加依赖
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.9.9.1</version>
</dependency>
异常通知: 在出现异常时,Spring AOP会自动捕获异常,并使用代理对象处理。
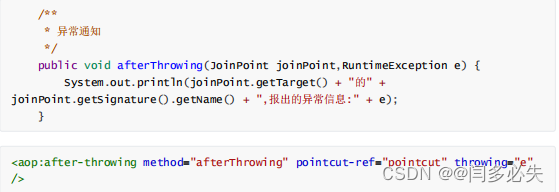
最终通知 Spring AOP的最终通知是通过finally实现的,无论发生任何情况,都会执行。
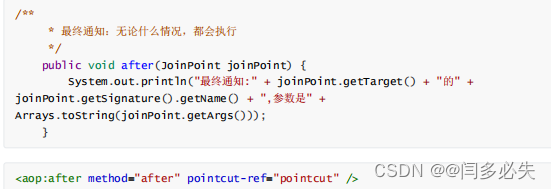
环绕通知 环绕通知功能非常强大,包含了四种全部通知类型,主要是通过暴露的原生方法调用所执行的目标方法,因此可以获取到原生方法的所有状态加以处理
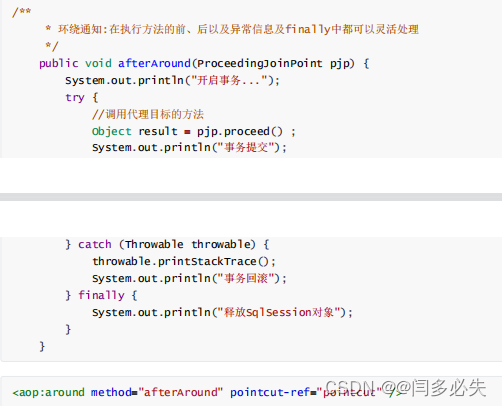
3. 使用注解实现IoC及DI依赖注入
3.1 在配置文件中打开包扫描机制
3.2 注解实现IoC (直接使用注解实现加载到容器当中)
3.3 使用Autowired或者是Resources来完成DI依赖注入
1.@Autowird : 按照类型实现自动装配,也就意味着在容器中必须拥有对应类型。如果出现了多个要 注入的类型,此时,IoC容器就会发现不知道该如何注入,可以通过@Qualifier来完成以名称为标识进行注入。
添加依赖:
@Autowired@Qualifier ( "userService" ) // 在没有自定义的情况下,采用要找到的类的名称首字母小写。private UserService userService ;
@Resourceprivate UserService userServiceImpl ;
默认情况下Resource会采用名称的匹配方式,要求变量名与被注解修饰的组件名称要一致! 如果不一致,此时Resource就不再通过名称来注入了,而是采用类型方式进行注入!
4.以Annotation注解形式实现AOP
4.1自定义一个全注解的AOP的类
package com.csi.proxy;
import org.aopalliance.intercept.Joinpoint;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.*;
import org.springframework.stereotype.Component;
import java.util.Arrays;
@Component
@Aspect
public class LogManagerAdvice {
//构造
public LogManagerAdvice(){
System.out.println("建立数据库连接.....");
}
@Pointcut("execution(* com.csi.service..*.*(..))")
public void pointcut(){ }
//前置增强
@Before("pointcut()")
public void before(JoinPoint joinpoint){
System.out.println("被增强的类:"+joinpoint.getTarget().getClass().getName()
+ "被拦截的方法:" +joinpoint.getSignature().getName()
+"参数是:"+ Arrays.toString(joinpoint.getArgs()));
System.out.println("开始记录日志。。。。");
}
//后置增强
@AfterReturning(pointcut = "pointcut()",returning = "o")
public void afterReturning(JoinPoint joinPoint ,Object o){
System.out.println("结束日志记录。。。");
}
//异常通知
@AfterThrowing(pointcut = "pointcut()",throwing = "e")
public void afterThrowing(JoinPoint joinPoint,RuntimeException e){
System.out.println(joinPoint.getTarget()+"的"+joinPoint.getSignature().getName()+",报出的信息是:"+e);
}
//最终通知
@After("pointcut()")
public void after(JoinPoint joinPoint){
System.out.println("最终通知:"+joinPoint.getTarget()+"的"+joinPoint.getSignature().getName()+",参数是"+Arrays.toString(joinPoint.getArgs()));
}
//环绕通知
@Around("pointcut()")
public void afterAround(ProceedingJoinPoint pjp){
System.out.println("开启事务.....");
try {
Object result = pjp.proceed();
System.out.println("事务提交....");
} catch (Throwable e) {
e.printStackTrace();
System.out.println("事务回滚....");
}finally {
System.out.println("释放SqlSession对象");
}
}
}