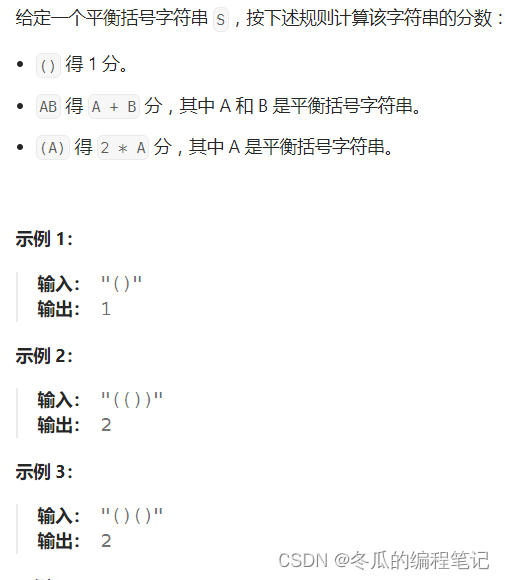
/** 栈 : 使用栈记录分数
* 思路: 遇到左括号向栈中存入0,遇到右括号弹出栈顶分数,若这个分数为0,
* 表示这个平衡括号字符串形式为 ‘()',然后再加上与这个字符串深度相同的字符串分数,例如:()(),
* 若这个分数不为0,则分数乘以2再加上与这个字符串深度相同的字符串分数,例如:()(A),
* @auther start
* @create 2023-11-20 20:22
*/
public class DL856 {
public int scoreOfParentheses(String s) {
Deque<Integer> stack = new LinkedList<>();
//存入0,防止出现同等深度的字符串只用一个的情况,例如:()
stack.push(0);
for (int i = 0; i < s.length(); i++) {
if (s.charAt(i) == '(') {
stack.push(0);
} else {
//弹出当前括号内的分数,如果当前括号内的分数为0,则不是 (A) 这个形式
//然后将这个括号的分数加上这个括号前面的分数,重新入栈
int v = stack.pop();
int top = stack.pop() + Math.max(2 * v, 1);
stack.push(top);
}
}
return stack.peek();
}
}