一.创建SpringBoot项目
(1)idea直接选择Spring initializr可创建SpringBoot项目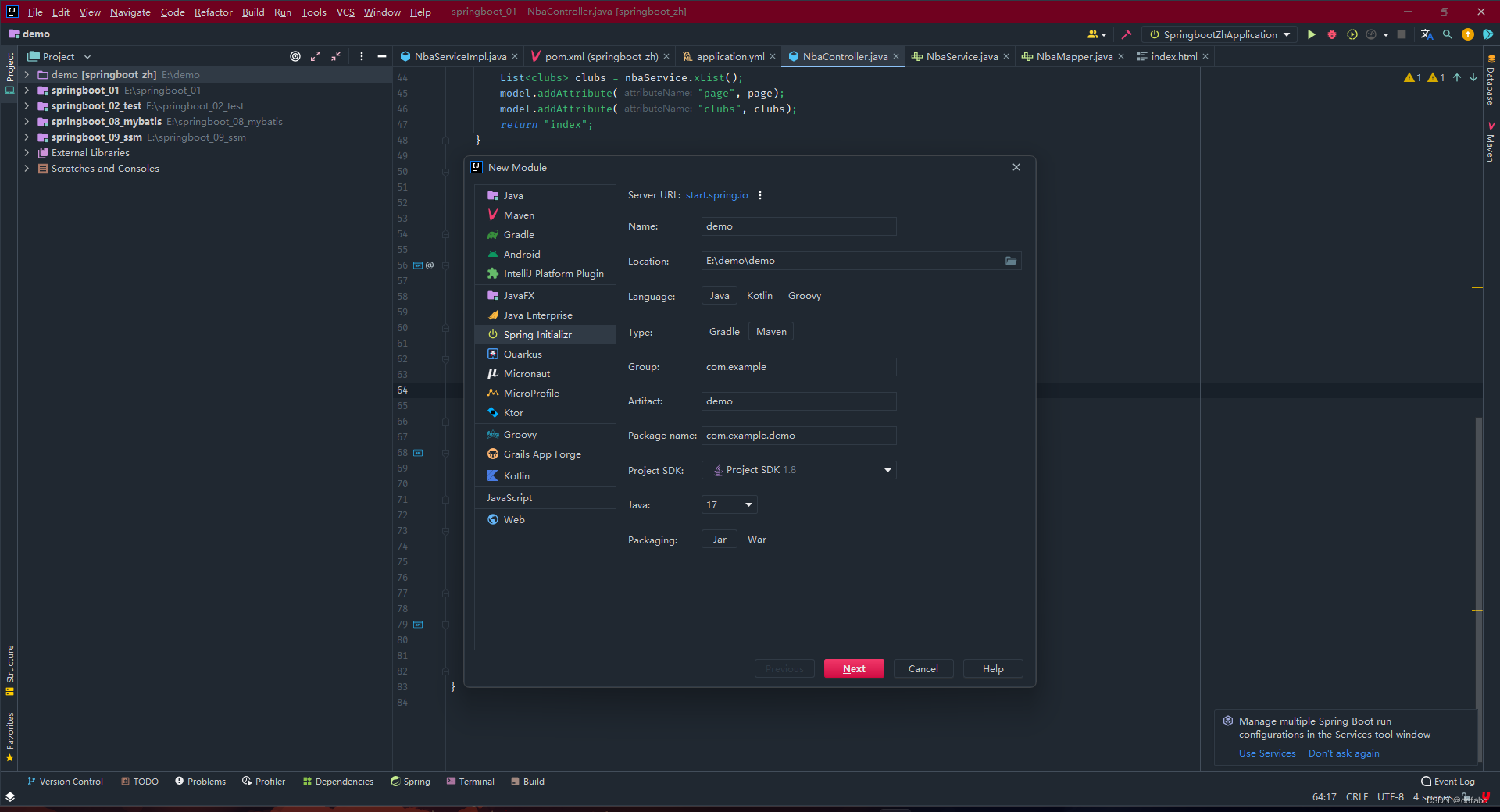
注意java版本选8,type选maven
(2)选择依赖
根据个人需求选择依赖,选择好后会直接在pom.xml中
项目架构
(3)配置
application.yml
spring:
main:
allow-circular-references: true //防止插件循环报错
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/nba?serverTimezone=UTC
username: root
password: root
type: com.alibaba.druid.pool.DruidDataSource
thymeleaf:
prefix: classpath:/templates/
suffix: .html
mode: LEGACYHTML5
//端口号
server:
port: 8080
//分页插件
pagehelper:
auto-dialect: true
reasonable: true
supportMethodsArguments: true
params: countSql
二.代码编写
pojo类
players表
package com.xu.pojo;
import org.springframework.format.annotation.DateTimeFormat;
import java.util.Date;
/**
* @Auther:الطريق إلى حادس
* @Date:2022/11/19
* @Description:
* @VERSON:1.8
**/
public class players {
private Integer pid;
private String pname;
@DateTimeFormat(pattern = "yyyy-MM-dd")
private Date birthday;
private Integer height;
private Integer weight;
private String position;
private Integer cid;
private String cname;
private clubs c;
public players() {
}
public players(Integer pid, String pname, Date birthday, Integer height, Integer weight, String position, Integer cid, String cname, clubs c) {
this.pid = pid;
this.pname = pname;
this.birthday = birthday;
this.height = height;
this.weight = weight;
this.position = position;
this.cid = cid;
this.cname = cname;
this.c = c;
}
public Integer getPid() {
return pid;
}
public void setPid(Integer pid) {
this.pid = pid;
}
public String getPname() {
return pname;
}
public void setPname(String pname) {
this.pname = pname;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
public Integer getHeight() {
return height;
}
public void setHeight(Integer height) {
this.height = height;
}
public Integer getWeight() {
return weight;
}
public void setWeight(Integer weight) {
this.weight = weight;
}
public String getPosition() {
return position;
}
public void setPosition(String position) {
this.position = position;
}
public Integer getCid() {
return cid;
}
public void setCid(Integer cid) {
this.cid = cid;
}
public String getCname() {
return cname;
}
public void setCname(String cname) {
this.cname = cname;
}
public clubs getC() {
return c;
}
public void setC(clubs c) {
this.c = c;
}
@Override
public String toString() {
return "players{" +
"pid=" + pid +
", pname='" + pname + '\'' +
", birthday=" + birthday +
", height=" + height +
", weight=" + weight +
", position='" + position + '\'' +
", cid=" + cid +
", cname='" + cname + '\'' +
", c=" + c +
'}';
}
}
clubs表
package com.xu.pojo;
/**
* @Auther:الطريق إلى حادس
* @Date:2022/11/19
* @Description:
* @VERSON:1.8
**/
public class clubs {
private Integer cid;
private String cname;
private String city;
public clubs() {
}
public clubs(Integer cid, String cname, String city) {
this.cid = cid;
this.cname = cname;
this.city = city;
}
public Integer getCid() {
return cid;
}
public void setCid(Integer cid) {
this.cid = cid;
}
public String getCname() {
return cname;
}
public void setCname(String cname) {
this.cname = cname;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
@Override
public String toString() {
return "clubs{" +
"cid=" + cid +
", cname='" + cname + '\'' +
", city='" + city + '\'' +
'}';
}
}
mapper
/**
* 两表联查
* @param name ture
* @param id ture
* @return List<players>
*/
@Select("select * from players pla left join clubs clu on pla.cid = clu.cid ")
List<players> listAll(@Param("name") String name, @Param("id") Integer id);
@Select(" select * from clubs")
List<clubs> xList();
service
PageInfo<players> list(Integer pageNum, String name, Integer id);
serviceimpl
@Override
public PageInfo<players> list(Integer pageNum, String name, Integer id) {
PageHelper.startPage(pageNum, 3);
//查询所有的信息
List<players> list = nbaMapper.listAll(name,id);
//获取分页相关数据
PageInfo<players> page = new PageInfo<players>(list, 5);
return page;
}
controller
/**
* 分页
*
* @param pageNum true
* @param name true
* @param id true
* @param model true
* @return String
*/
@RequestMapping(value = "list/{pageNum}",method = RequestMethod.GET)
public String list(@PathVariable("pageNum") Integer pageNum, String name, Integer id, Model model) {
PageInfo<players> page = nbaService.list(pageNum, name, id);
//下拉框查询
List<clubs> clubs = nbaService.xList();
model.addAttribute("page", page);
model.addAttribute("clubs", clubs);
return "index";
}
前端页面 html
index.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>美国职业篮球联盟(NBA)球员信息</h1>
<form th:action="@{/list/1}" method="get">
球员姓名:<input type="text" name="name" >
所属球队:<select name="id">
<option value="">--请选择--</option>
<option th:each="cmsType:${clubs}" th:value="${cmsType.cid}">[[${cmsType.cname}]]</option>
</select>
<input type="submit" value="查询">
<button th:formaction="add">添加球员信息</button>
</form>
<table border="1">
<tr>
<th>球员编号</th>
<th>球员名称</th>
<th>出生时间</th>
<th>球员生高</th>
<th>球员体重</th>
<th>球员位置</th>
<th>所属球队</th>
<th>相关操作</th>
</tr>
<tr th:each="page:${page.list}">
<td th:text="${page.pid}"></td>
<td th:text="${page.pname}"></td>
<td th:text="${page.birthday}"></td>
<td th:text="${page.height}"></td>
<td th:text="${page.weight}"></td>
<td th:text="${page.position}"></td>
<td th:text="${page.cname}">所属球队</td>
<td><a th:href="@{'/delete/'+${page.pid}}">删除</a></td>
</tr>
</table>
<div>
<a th:if="${page.hasPreviousPage}" th:href="@{/list/1}">首页</a>
<a th:if="${page.hasPreviousPage}" th:href="@{'/list/'+${page.prePage}}">上一页</a>
<span th:each="num:${page.navigatepageNums}">
<a th:if="${page.pageNum == num}" style="color:red;" th:href="@{'/list/'+${num}}"
th:text="'['+${num}+']'"></a>
<a th:if="${page.pageNum != num}" th:href="@{'/list/'+${num}}" th:text="${num}"></a>
</span>
<a th:if="${page.hasNextPage}" th:href="@{'/list/'+${page.nextPage}}">下一页</a>
<a th:if="${page.hasNextPage}" th:href="@{'/list/'+${page.pages}}">尾页</a>
</div>
</body>
</html>
add.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>添加球员</h1>
<form th:action="addList" method="post">
球员名称:<input type="text" name="pname"><br/><br/>
出生时间:<input type="text" name="birthday"><br/><br/>
球员生高:<input type="text" name="height"><br/><br/>
球员体重:<input type="text" name="weight"><br/><br/>
球员位置:<input type="radio" name="position" value="控球后卫">控球后卫
<input type="radio" name="position" value="得分后卫">得分后卫
<input type="radio" name="position" value="小前锋">小前锋
<input type="radio" name="position" value="大前锋">大前锋
<input type="radio" name="position" value="中锋">中锋
<br/><br/>
所属球队:<select name="cid">
<option value="">--请选择--</option>
<option th:each="cmsType:${clubs}" th:value="${cmsType.cid}">[[${cmsType.cname}]]</option>
</select>
<br/><br/>
相关操作:<input value="新增" type="submit">
<button th:formaction="1">返回</button>
</form>
</body>
</html>
完成