1、运算符重载
#include <iostream>
using namespace std;
class Person
{
private:
int age;
int height;
public:
//无参构造
Person():height(190)
{
age=19;
}
//有参构造
Person(int age,int height)
{
this->age=age;
this->height=height;
}
//拷贝构造函数
Person(Person &other)
{
age=other.age;
height=other.height;
cout<<"调用了拷贝构造函数1"<<endl;
}
//拷贝赋值函数
/*Person &operator=(Person &other)
{
age=other.age;
height=other.height;
cout<<"调用了拷贝赋值函数2"<<endl;
return *this;
}*/
//析构函数
~Person()
{
cout<<"--调用了析构函数--ending"<<endl;
}
void show()
{
cout<<"age="<<age<<endl;
cout<<"height="<<height<<endl;
}
//算数运算符重载
Person operator+(Person &p2)
{
Person temp;
temp.age=this->age+p2.age;
temp.height=this->height+p2.height;
return temp;
}
Person operator-(Person &p2)
{
Person temp;
temp.age=this->age-p2.age;
temp.height=this->height-p2.height;
return temp;
}
Person operator*(Person &p2)
{
Person temp;
temp.age=this->age*p2.age;
temp.height=this->height*p2.height;
return temp;
}
Person operator/(Person &p2)
{
Person temp;
temp.age=this->age/p2.age;
temp.height=this->height/p2.height;
return temp;
}
Person operator%(Person &p2)
{
Person temp;
temp.age=this->age%p2.age;
temp.height=this->height%p2.height;
return temp;
}
// 赋值运算符
Person &operator+=(Person &p2)
{
this->age+=p2.age;
this->height+=p2.height;
return *this;
}
Person &operator-=(Person &p2)
{
this->age-=p2.age;
this->height-=p2.height;
return *this;
}
Person &operator*=(Person &p2)
{
this->age*=p2.age;
this->height*=p2.height;
return *this;
}
Person &operator/=(Person &p2)
{
this->age/=p2.age;
this->height/=p2.height;
return *this;
}
Person &operator%=(Person &p2)
{
this->age%=p2.age;
this->height%=p2.height;
return *this;
}
//逻辑与与逻辑或运算符
bool operator&&(Person &p2)
{
return (this->age&&p2.age)&&(this->height&&p2.height);
}
bool operator||(Person &p2)
{
return (this->age||p2.age)||(this->height||p2.height);
}
//关系运算符
bool operator==(Person &p2)
{
return (this->age==p2.age)&&(this->height==p2.height);
}
bool operator!=(Person &p2)
{
return (this->age!=p2.age)||(this->height!=p2.height);
}
bool operator>=(Person &p2)
{
return (this->age>=p2.age)&&(this->height>=p2.height);
}
bool operator<=(Person &p2)
{
return (this->age<=p2.age)&&(this->height<=p2.height);
}
bool operator>(Person &p2)
{
return (this->age>p2.age)&&(this->height>p2.height);
}
bool operator<(Person &p2)
{
return (this->age<p2.age)&&(this->height<p2.height);
}
//单目运算符
bool operator!()
{
return !(this->age||this->height);
}
Person operator~()
{
Person temp;
temp.age=~this->age;
temp.height=~this->height;
return temp;
}
Person &operator++()//前缀自增
{
++this->age;
++this->height;
return *this;
}
Person &operator++(int)//后缀自增
{
static Person temp;
temp=*this;
this->age++;
this->height++;
return temp;
}
Person &operator--()//前缀自增
{
--this->age;
--this->height;
return *this;
}
Person &operator--(int)//后缀自增
{
Person temp;
temp=*this;
this->age--;
this->height--;
return temp;
}
Person operator-()//负号
{
static Person temp;
temp.age=-this->age;
temp.height=-this->height;
return temp;
}
};
int main()
{
Person p1;
p1.show();
Person p2;
p2.show();
Person p3;
p3=p1+p2;
p3.show();
cout<<(p1&&p2)<<endl;
cout<<(!p1)<<endl;
Person p4=p1++;
p4.show();
(-p1).show();
(~p1).show();
return 0;
}
2、思维导图
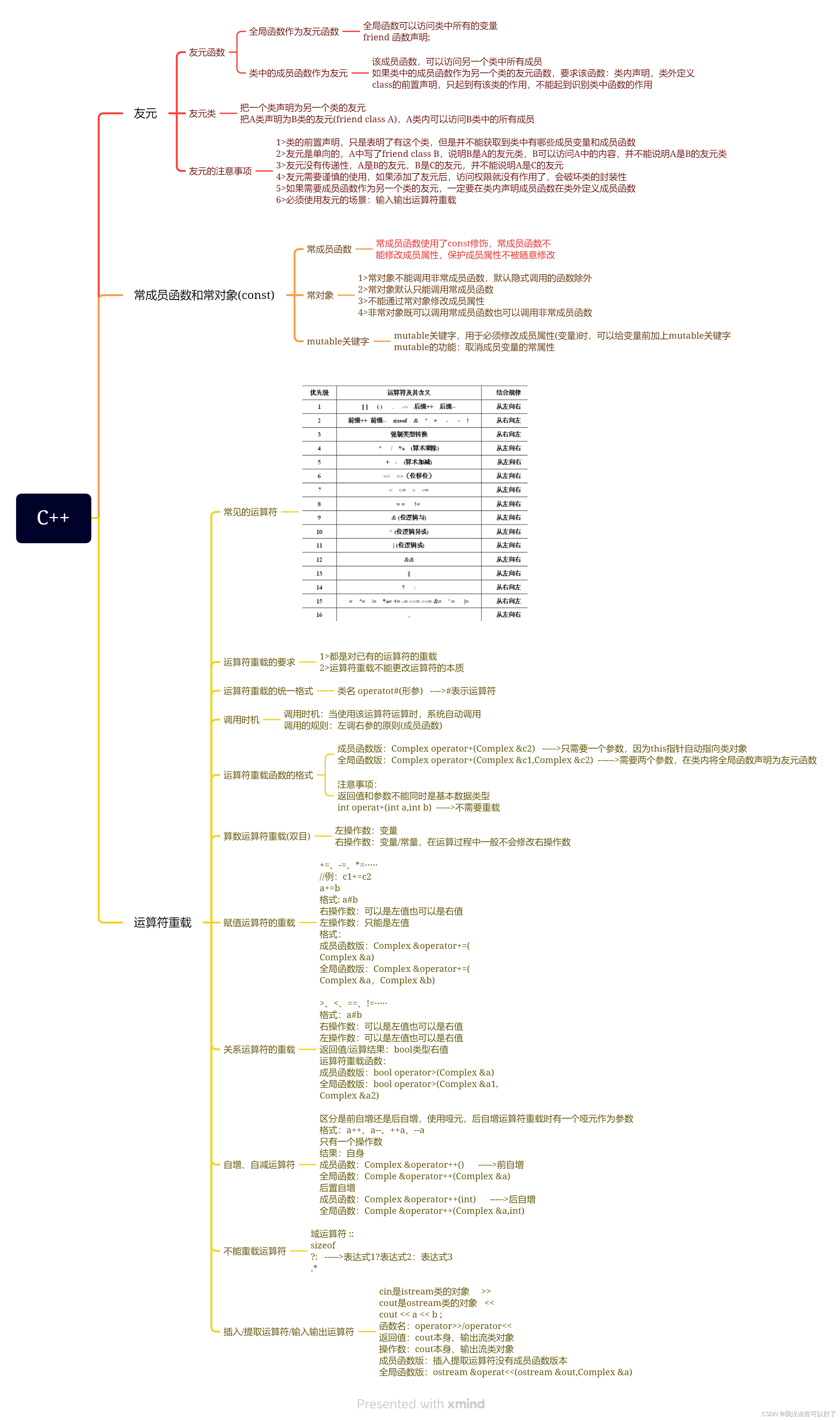