void save(stu_t* head,const char* filename){
FILE* fp = fopen(filename,"w");
stu_t* p = head->next;
while(p != NULL){
fwrite(p,sizeof(stu_t),1,fp);
p = p->next;
}
perror("save:");
fclose(fp);
}
void load(stu_t* head,const char* filename){
FILE* fp = fopen(filename,"rb");
if(fp == NULL){
printf("文件不存在\n");
return;
}
struct Data data = {0};
while( fread(&data,sizeof(stu_t),1,fp) == 1){
insert_stu(head,data);
}
perror("load:");
fclose(fp);
}
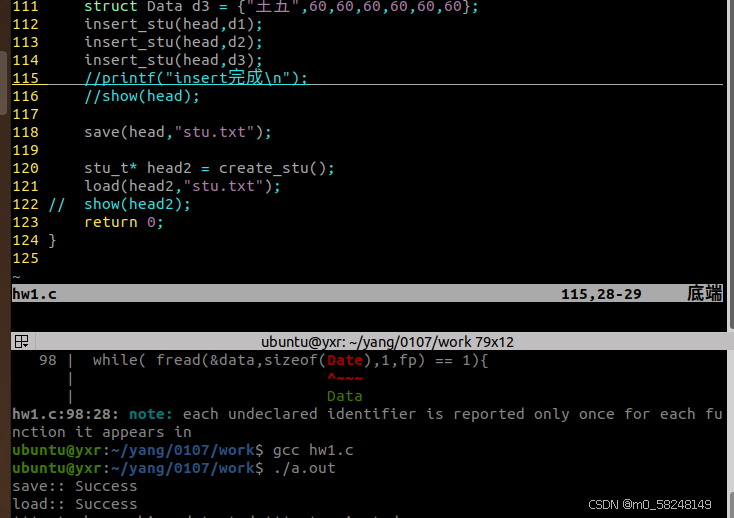
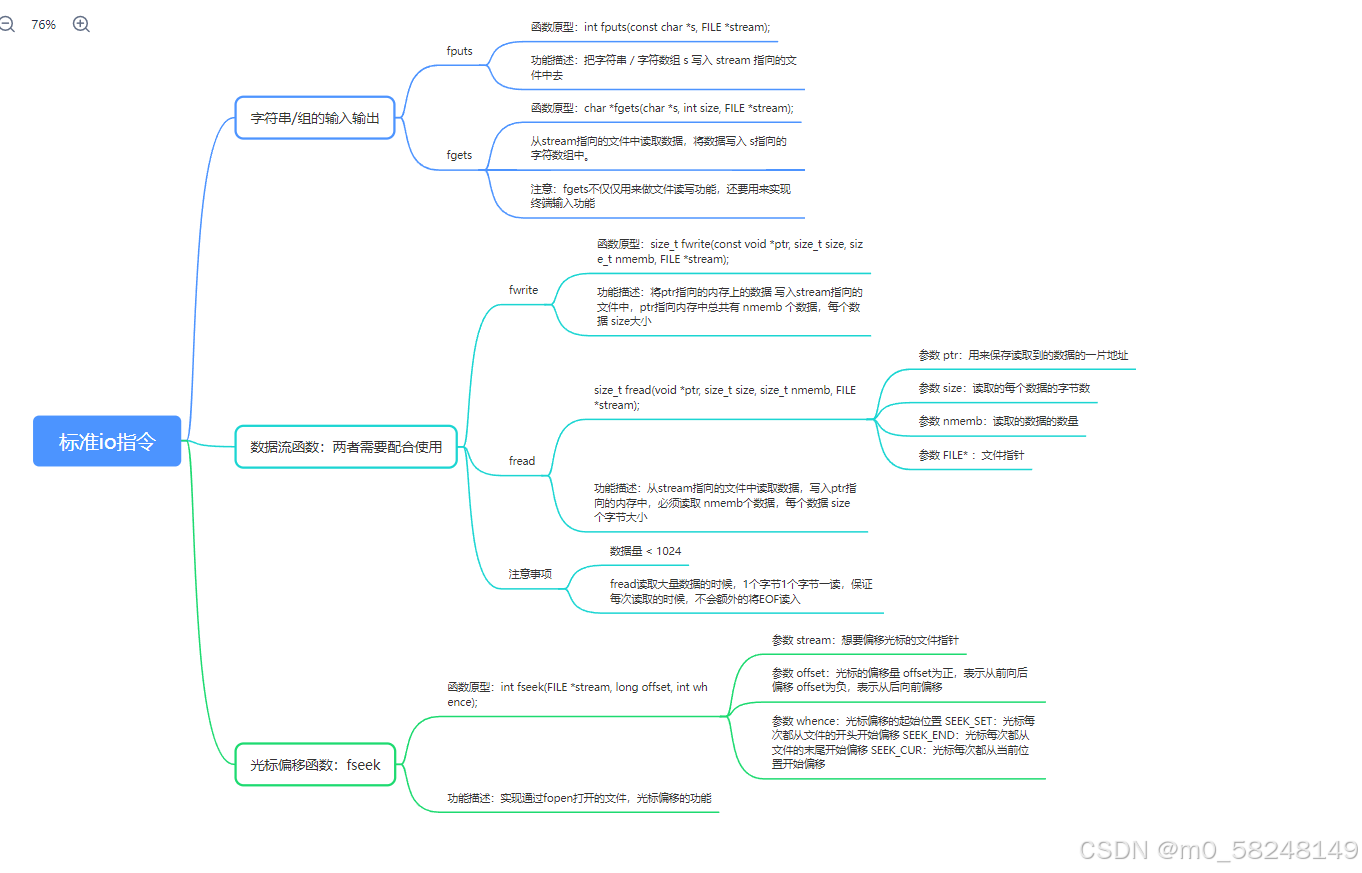
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main(int argc, const char *argv[])
{
FILE* fp=fopen("rising_freedom.bmp","r+");
if(fp == NULL)
{
perror("fopen");
return 1;
}
int bmp_size = 0,bmp_width=0,bmp_height= 0;
fseek(fp,2,SEEK_SET);
fread(&bmp_size,4,1,fp);
printf("%d\n",bmp_size);
fseek(fp,18,SEEK_SET);
fread(&bmp_width,4,1,fp);
fread(&bmp_height,4,1,fp);
printf("%d*%d\n",bmp_width,bmp_height);
fseek(fp,54,SEEK_SET);
unsigned char bgr[3]= {0,200,200};
for(int i=0;i<bmp_width*bmp_height/3;i++)
{
fwrite(bgr,3,1,fp);
}
fseek(fp,54,SEEK_CUR);
unsigned char bgr2[3]= {0,0,222};
for(int i=0;i<bmp_width*bmp_height/3;i++)
{
fwrite(bgr2,3,1,fp);
}
fseek(fp,54,SEEK_CUR);
unsigned char bgr3[3]= {0,0,0};
for(int i=0;i<bmp_width*bmp_height/3;i++)
{
fwrite(bgr3,3,1,fp);
}
fclose(fp);
return 0;
}
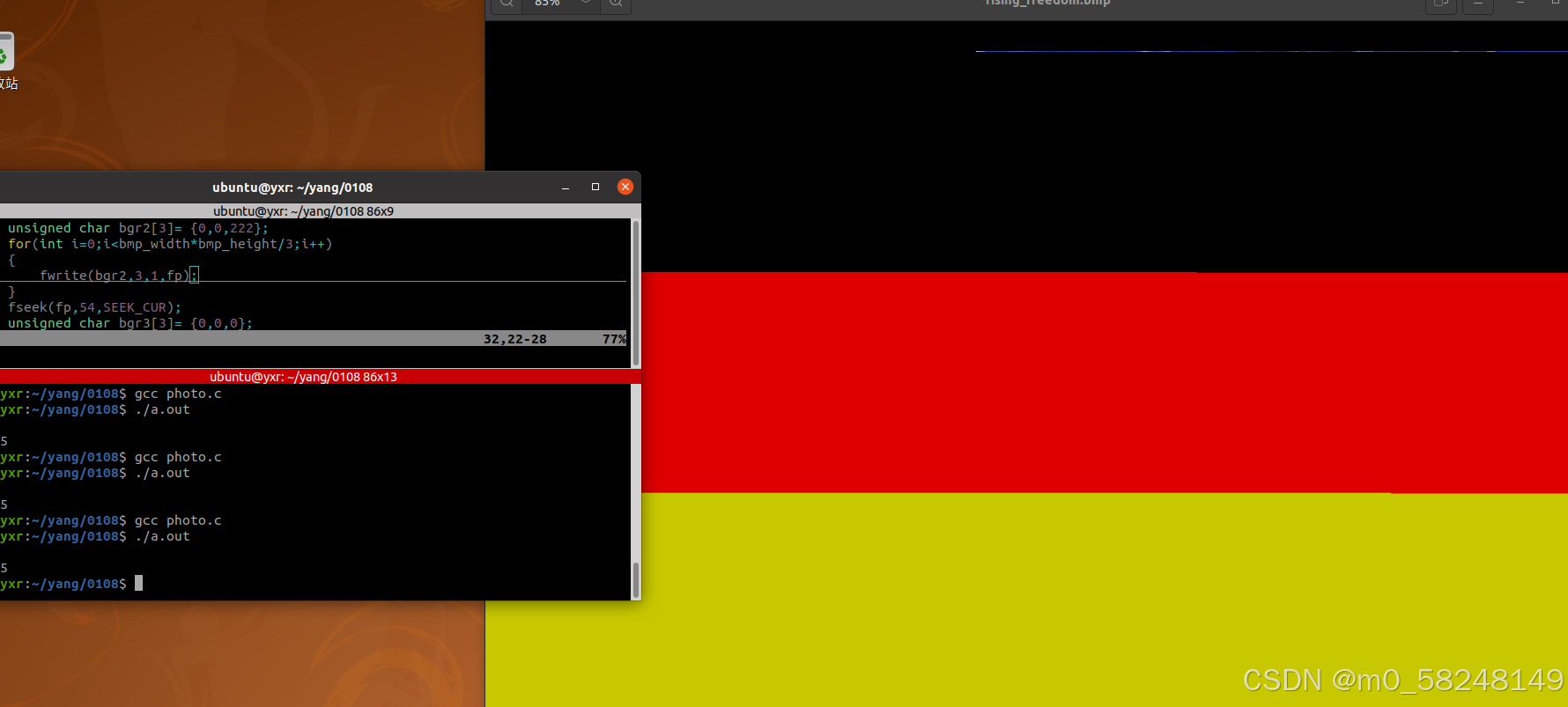