1.创建链表
#include <iostream>
#include <stdlib.h>
using namespace std;
struct List
{
int data;
struct List* next;
};
void chreat(struct List *L)
{
int m;
cin>>m;
while(m)
{
struct List* p = (struct List *)malloc(sizeof(struct List));
p->data = m;
p->next = NULL;
L->next = p;
L = L->next;
cin>>m;
}
}
void show(struct List* L)
{
cout<<"输出结果为:";
while(L->next)
{
cout<<L->next->data<<' ';
L = L->next;
}
cout<<endl;
}
int main()
{
struct List* head_list = (struct List *)malloc(sizeof(struct List));
head_list->next = NULL;
cout<<"输入的值为:";
chreat(head_list);
show(head_list);
return 0;
}
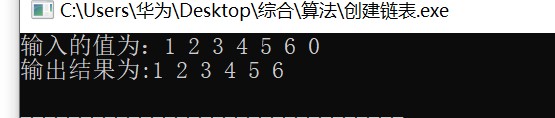
2.插入链表
#include <iostream>
#include <stdlib.h>
using namespace std;
struct List
{
int data;
struct List* next;
};
void chreat(struct List *L)
{
int m;
cin>>m;
while(m)
{
struct List* p = (struct List *)malloc(sizeof(struct List));
p->data = m;
p->next = NULL;
L->next = p;
L = L->next;
cin>>m;
}
}
void show(struct List* L)
{
cout<<"输出结果为:";
while(L->next)
{
cout<<L->next->data<<' ';
L = L->next;
}
cout<<endl;
}
void add(struct List* L,int k,int m)
{
struct List* q = NULL;
struct List* p = (struct List *)malloc(sizeof(struct List));
p->data = m;
p->next = NULL;
while(k--&&L->next)
{
q = L;
L = L->next;
}
if(k>0)
{
L->next = p;
}
else
{
q->next = p;
p->next = L;
}
}
int main()
{
int k,m;
struct List* head_list = (struct List *)malloc(sizeof(struct List));
head_list->next = NULL;
cout<<"输入的值为:";
chreat(head_list);
cout<<"请输入第几位前插入元素几:"<<endl;
cin>>k>>m;
add(head_list,k,m);
show(head_list);
return 0;
}
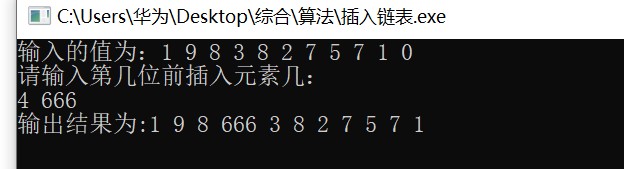
1.后插入链表
#include <iostream>
using namespace std;
struct List
{
int data;
struct List* next;
};
void ba_add(struct List* &head)
{
cout<<"请输入数据:";
struct List* q = head;
int m;
cin>>m;
while(m)
{
struct List* p = (struct List *)malloc(sizeof(struct List));
p->data = m;
p->next = NULL;
q->next = p;
q = p;
cin>>m;
}
}
void show(struct List* &head)
{
cout<<"输出结果为:";
while(head->next)
{
cout<<head->next->data<<' ';
head = head->next;
}
cout<<endl;
}
int main()
{
struct List* head = (struct List *)malloc(sizeof(struct List));
head->next = NULL;
ba_add(head);
show(head);
return 0;
}
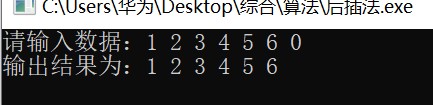
2.前插入链表
#include <iostream>
using namespace std;
struct List
{
int data;
struct List* next;
};
void ba_add(struct List* &head)
{
cout<<"请输入数据:";
struct List* L = head;
int m;
cin>>m;
while(m)
{
struct List* p = (struct List *)malloc(sizeof(struct List));
p->data = m;
p->next = NULL;
p->next = L->next;
L->next = p;
cin>>m;
}
}
void show(struct List* &head)
{
cout<<"输出结果为:";
while(head->next)
{
cout<<head->next->data<<' ';
head = head->next;
}
cout<<endl;
}
int main()
{
struct List* head = (struct List *)malloc(sizeof(struct List));
head->next = NULL;
ba_add(head);
show(head);
return 0;
}
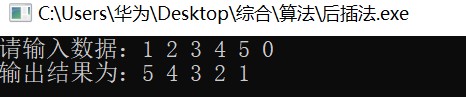
3.删除链表
#include <iostream>
#include <stdlib.h>
using namespace std;
struct List
{
int data;
struct List* next;
};
void chreat(struct List *L)
{
int m;
cin>>m;
while(m)
{
struct List* p = (struct List *)malloc(sizeof(struct List));
p->data = m;
p->next = NULL;
L->next = p;
L = L->next;
cin>>m;
}
}
void show(struct List* L)
{
cout<<"输出结果为:";
while(L->next)
{
cout<<L->next->data<<' ';
L = L->next;
}
cout<<endl;
}
void del(struct List* &head_list, int i)
{
int j=0;
struct List *q;
struct List *p = head_list;
while((p->next)&&(j<i-1))
{
p = p->next;
++j;
}
if(!(p->next)||j>i-1) cout<<"此处删除位置不合理";
q = p->next;
p->next = q->next;
free(q);
}
int main()
{
int i;
struct List* head_list = (struct List *)malloc(sizeof(struct List));
head_list->next = NULL;
cout<<"输入的值为:";
chreat(head_list);
cout<<"请输入要删除第几位:"<<endl;
cin>>i;
del(head_list,i);
show(head_list);
return 0;
}
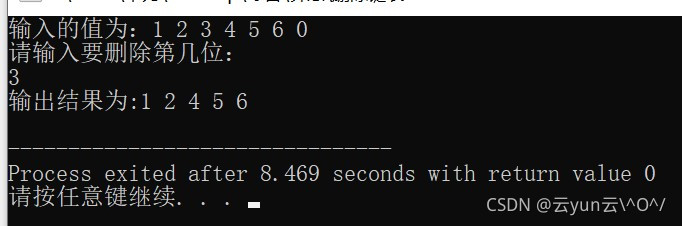